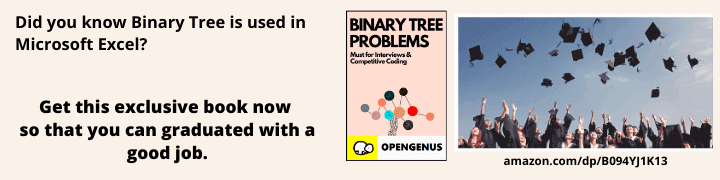
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of content
- PyTorch - Some of unique features
- Installation process
- Tensors
- Datasets and DataLoaders
- Deep Learning Model
- PyTorch vs Tensorflow
PyTorch is an open-source deep learning framework primarily developed by Facebook's AI Research lab (FAIR). It provides a flexible and dynamic computational graph, making it particularly suitable for research and experimentation in deep learning tasks. Some of its unique features include:
- Dynamic Computational Graphs: PyTorch uses a dynamic computational graph approach, where the graph is built dynamically as operations are performed, allowing for more flexibility and easier debugging compared to static graphs used in frameworks like TensorFlow.
- Imperative Programming Style: PyTorch adopts an imperative programming style, meaning operations are executed as they are called, similar to NumPy. This makes it easier to understand and debug code, especially for beginners and researchers.
- TorchScript: PyTorch offers TorchScript, a way to create serializable and optimizable models that can be deployed independently from the Python runtime. This feature is particularly useful for production deployment and mobile applications.
- Rich Ecosystem: PyTorch has a vibrant and growing ecosystem with a vast collection of libraries and tools built on top of it, including torchvision for computer vision tasks, torchaudio for audio processing, and torchtext for natural language processing.
- Native CUDA Support: PyTorch seamlessly integrates with NVIDIA's CUDA platform, allowing for easy acceleration of computations on GPUs, which is crucial for training large deep learning models efficiently.
- Automatic Differentiation: PyTorch provides automatic differentiation functionality through its autograd package, which automatically computes gradients of tensors with respect to a given loss function. This feature simplifies the implementation of gradient-based optimization algorithms.
- Dynamic Neural Networks: PyTorch enables the creation of dynamic neural networks, where the structure of the network can be altered on-the-fly during runtime. This flexibility is particularly useful for building models with variable input sizes or complex architectures.
Installation process -
Installing PyTorch can be done using various methods depending on your system configuration and preferences. Here, I'll outline the steps for installing PyTorch via pip, which is the recommended method for most users.
Prerequisites
Before installing PyTorch, ensure you have Python installed on your system. PyTorch supports Python versions 3.6 and above.
pip install torch torchvision torchaudio
This command installs PyTorch along with torchvision, which provides utility functions for working with image and video data, and torchaudio, which provides utility functions for audio data.
After the installation completes, you can verify that PyTorch is installed correctly by opening a Python interpreter or creating a Python script and running the following code:
import torch
print(torch.__version__)
Additional Options:
CUDA Support: If you have an NVIDIA GPU and want to utilize CUDA for accelerated computations, you can install the appropriate version of PyTorch with CUDA support. Replace the above pip install command with the following command:
pip install torch torchvision torchaudio cudatoolkit=version
Replace 'version' with the version of CUDA installed on your system.
Conda: If you prefer using Conda, PyTorch can also be installed via Conda. However, the pip installation method is generally more straightforward for most users.
That's it! You've now successfully installed PyTorch on your system and are ready to start building deep learning models.
Tensors -
In PyTorch, tensors are the fundamental data structures used for representing data. They are similar to NumPy arrays but come with additional functionalities optimized for deep learning operations, such as automatic differentiation for gradient-based optimization.
import torch
#Create a tensor from a Python list
tensor_list = torch.tensor([1, 2, 3, 4, 5])
#Create a tensor of zeros
zeros_tensor = torch.zeros(3, 4)
# Creates a 3x4 tensor filled with zeros
#Create a tensor of ones
ones_tensor = torch.ones(2, 3)
# Creates a 2x3 tensor filled with ones
#Create a tensor with random values
random_tensor = torch.rand(3, 3)
# Creates a 3x3 tensor with random values
# Create a tensor with a specific data type
dtype_tensor = torch.tensor([1.0, 2.0, 3.0], dtype=torch.float64)
Operations on Tensors:
Element-wise addition
result_tensor = tensor1 + tensor2
Element-wise multiplication
result_tensor = tensor1 * tensor2
Matrix multiplication
result_tensor = torch.matmul(matrix1, matrix2)
Transpose
transposed_tensor = tensor.T
Reshaping
reshaped_tensor = tensor.view(new_shape)
Indexing and slicing
subset_tensor = tensor[indices]
Automatic Differentiation:
One of the key features of PyTorch is its ability to perform automatic differentiation, which is essential for training neural networks. PyTorch tracks operations performed on tensors and automatically computes gradients with respect to input tensors.
#Define tensors with requires_grad=True to enable gradient #tracking
x = torch.tensor(2.0, requires_grad=True)
y = torch.tensor(3.0, requires_grad=True)
#Perform operations
z = x * y
loss = z**2
#Compute gradients
loss.backward()
#Access gradients
print(x.grad) # Gradient of loss w.r.t. x
print(y.grad) # Gradient of loss w.r.t. y
Datasets and DataLoaders
In PyTorch, datasets and data loaders are essential components for handling data in deep learning tasks, facilitating efficient data loading, preprocessing, and batching during model training and evaluation.
Datasets:
A dataset in PyTorch represents a collection of data samples and their corresponding labels, if applicable. PyTorch provides several built-in datasets and utilities to work with popular benchmark datasets like MNIST, CIFAR-10, and ImageNet. Additionally, users can create custom datasets by subclassing the torch.utils.data.Dataset class and implementing the __len__
and __getitem__
methods.
import torch
from torch.utils.data import Dataset, DataLoader
from torchvision import datasets, transforms
class CustomDataset(Dataset):
def __init__(self, data, targets, transform=None):
self.data = data
self.targets = targets
self.transform = transform
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
sample, label = self.data[idx], self.targets[idx]
if self.transform:
sample = self.transform(sample)
return sample, label
DataLoaders:
Data loaders are responsible for creating batches of data from a dataset, shuffling the data, and parallelizing data loading. They provide an iterable interface for accessing the data during model training and evaluation efficiently. Data loaders can also handle automatic batching and parallel data loading using multiple workers.
# Define transform
transform = transforms.Compose([
transforms.ToTensor(), # Convert image to tensor
transforms.Normalize((0.5,), (0.5,)) #Normalize image data
])
# Create dataset
train_dataset = datasets.MNIST(root='./data', train=True, transform=transform, download=True)
# Create DataLoader
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True)
Deep Learning Model
Building a deep learning model in PyTorch typically involves defining the model architecture, specifying how data flows through the network, and implementing the forward pass. Here's a step-by-step guide to building a simple neural network model using PyTorch:
Step 1: Define the Model Architecture
Start by defining the architecture of your neural network. You can create a custom model by subclassing torch.nn.Module
and implementing the __init__
and forward
methods.
import torch
import torch.nn as nn
class SimpleNN(nn.Module):
def __init__(self, input_size, hidden_size, num_classes):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(hidden_size, num_classes)
def forward(self, x):
out = self.fc1(x)
out = self.relu(out)
out = self.fc2(out)
return out
Step 2: Instantiate the Model
Create an instance of the model by providing the input size, hidden size, and number of classes.
input_size = 784 # Example: for MNIST dataset (28x28 images)
hidden_size = 128
num_classes = 10 # Example: for MNIST dataset (10 classes)
model = SimpleNN(input_size, hidden_size, num_classes)
Step 3: Define Loss Function and Optimizer
Specify the loss function to optimize and the optimizer to update the model parameters during training.
# Cross-entropy loss for classification tasks
criterion = nn.CrossEntropyLoss()
# Stochastic Gradient Descent (SGD) optimizer
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
Step 4: Training Loop
Iterate over the dataset using a data loader, perform forward and backward passes, and update model parameters.
for epoch in range(num_epochs):
for batch_idx, (data, targets) in enumerate(train_loader):
# Forward pass
outputs = model(data)
loss = criterion(outputs, targets)
# Backward pass and optimization
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (batch_idx+1) % 100 == 0:
print(f'Epoch [{epoch+1}/{num_epochs}],
Step[{batch_idx+1}/{total_steps}],Loss{loss.item():.4f}')
Step 5: Evaluation
with torch.no_grad():
correct = 0
total = 0
for data, labels in test_loader:
outputs = model(data)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f'Accuracy of the model on the test images: {100 * correct / total}%')
By following these steps, you can build, train, and evaluate a simple neural network model using PyTorch. Experiment with different architectures, loss functions, and optimization techniques to improve model performance for your specific task.
PyTorch vs Tensorflow
- Dynamic vs. Static Computational Graphs:
- TensorFlow uses a static computational graph paradigm, where you define the computational graph once and then execute it multiple times. This can be beneficial for performance optimization and deployment on production systems.
- PyTorch, on the other hand, employs a dynamic computational graph approach. This means that the graph is built on-the-fly during the execution of the program, allowing for more flexibility and ease of debugging.
- Ease of Use:
- PyTorch is often praised for its simplicity and ease of use. Its dynamic graph construction makes it more Pythonic and intuitive, especially for researchers and beginners.
- TensorFlow has a steeper learning curve, particularly for those new to deep learning frameworks. However, TensorFlow 2.x introduced the Keras API as its high-level API, which significantly improved its usability and made it more accessible.
- Community and Ecosystem:
- TensorFlow has a larger user base and a more mature ecosystem with extensive documentation, tutorials, and community support. It's backed by Google, which contributes to its widespread adoption in industry.
- PyTorch has been rapidly gaining popularity, especially in the research community, partly due to its ease of use and dynamic graph capabilities. It has a growing ecosystem with many third-party libraries and contributions from both academia and industry.
- Deployment:
- TensorFlow has strong support for deployment in production environments, with tools like TensorFlow Serving, TensorFlow Lite for mobile and embedded devices, and TensorFlow.js for deploying models in web browsers.
- PyTorch provides deployment options as well, but TensorFlow's ecosystem and tooling for production deployment are more mature and widely adopted.
- Visualization and Debugging:
- TensorFlow offers TensorBoard, a powerful visualization toolkit for visualizing and debugging computational graphs, monitoring training metrics, and exploring model performance.
- PyTorch has tools like TensorBoardX and third-party integrations with TensorBoard for visualization, but its visualization ecosystem may not be as extensive as TensorFlow's.
Ultimately, the choice between TensorFlow and PyTorch often depends on factors such as personal preference, project requirements, existing infrastructure, and the specific use case at hand. Both frameworks are powerful and capable of handling a wide range of machine learning and deep learning tasks.