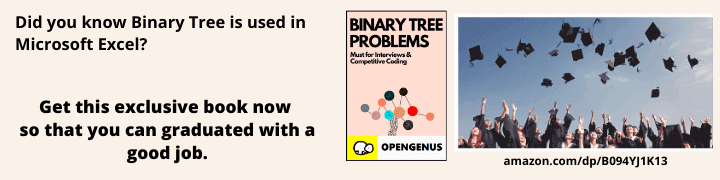
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Abstract
This OpenGenus article explores the application of machine learning techniques in predicting waiter tips based on factors such as total bill, day and time. Written in Python, the code employs one-hot encoding and Linear Regression, offering an understanding of these concepts. Additionally, it discusses the potential of advanced methods like decision trees and deep learning for intricate pattern recognition.
Table of Contents
Topics | |
---|---|
1. | Introduction |
2. | The Code |
3. | The CSV File |
4. | About the Modules |
- Pandas | |
- Scikit-Learn | |
5. | Core Concepts |
- One-Hot Encoding | |
- Linear Regression | |
6. | About the Code |
7. | Comparison with Other Approaches |
8. | Conclusion |
Introduction
In the bustling world of restaurants and bars, understanding customer behavior is crucial. One particular challenge faced by waitstaff is predicting tips accurately. Predicting tips aids in service optimization, leading to satisfied customers and a thriving business.
In this article, we explore the application of machine learning techniques to solve this problem efficiently. Our approach utilizes a Linear Regression model, a foundational algorithm in machine learning, to predict tips based on factors such as total bill, day, time, and party size. The choice of Linear Regression is apt here because it assumes a linear relationship between input features and the target variable, which aligns with the intuitive understanding that these factors contribute linearly to the tip amount. This method offers a good balance between simplicity and accuracy, making it an ideal starting point for prediction tasks in the restaurant context.
The Code
Here's the code:
import pandas as pd
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import OneHotEncoder
import joblib
data = pd.read_csv('dataset.csv')
X = data[['total_bill', 'day', 'time', 'size']]
y = data['tip']
encoder = OneHotEncoder(sparse=False, drop='first')
X_encoded = encoder.fit_transform(X[['day', 'time']])
feature_names = encoder.get_feature_names_out(['day', 'time'])
X_encoded_df = pd.DataFrame(X_encoded, columns=feature_names)
X = pd.concat([X.drop(['day', 'time'], axis=1), X_encoded_df], axis=1)
model = LinearRegression()
model.fit(X, y)
joblib.dump(model, 'tip_prediction_model.pkl')
loaded_model = joblib.load('tip_prediction_model.pkl')
new_data = pd.DataFrame({
'total_bill': [40.00],
'day': ['Sun'],
'time': ['Dinner'],
'size': [4]
})
new_data_encoded = encoder.transform(new_data[['day', 'time']])
new_data_encoded_df = pd.DataFrame(new_data_encoded, columns=feature_names)
new_data_final = pd.concat([new_data.drop(['day', 'time'], axis=1), new_data_encoded_df], axis=1)
predicted_tip = loaded_model.predict(new_data_final)
formatted_tip = "{:.2f}".format(predicted_tip[0])
print('Predicted Tip: ', formatted_tip)
The CSV file:
Here we are reading data from a csv file called, 'dataset.csv'. To make it easier to understand, here's what 'dataset.csv' might look like:
total_bill,day,time,size,tip
50.81,Sun,Dinner,3,10
20.75,Thu,Lunch,2,3
31.68,Sun,Dinner,2,4
About the modules:
The modules used here are pandas and scikit-learn.
Pandas: Pandas is a powerful and popular open-source Python library for data manipulation and analysis. It provides versatile data structures like DataFrames and Series, which enable users to efficiently work with structured data, such as CSV files, databases, and Excel spreadsheets. Pandas simplifies data cleaning, transformation, and exploration tasks, making it an essential tool for data scientists, analysts, and researchers.
Scikit-Learn: Scikit-Learn, often referred to as sklearn, is a powerful and widely used open-source Python library for machine learning and data science. It provides a user-friendly and consistent interface for various machine learning tasks, including classification, regression, clustering, dimensionality reduction, and more.
Joblib: Joblib provides tools for efficiently saving and loading Python objects, including large data arrays, with a focus on numerical data. It is particularly useful for serializing machine learning models and complex objects, allowing for faster processing and sharing across different Python environments.
Some core concepts:
One-Hot Encoding:
Dealing with categorical variables like 'day' and 'time' is challenging. One-hot encoding comes to help here. For example, when we encode 'Sun' and 'Dinner', we create binary columns (1s and 0s) representing each category. This ensures our algorithm comprehends the categorical data effectively.
Linear Regression:
Linear regression is a fundamental machine learning algorithm. It assumes a linear relationship between the input variables and the target variable ('tip' in this case). Through mathematical computations, the algorithm finds the best-fit line that predicts tips based on our chosen features.
About the code
Let me now explain my approach of predicting the waiter's tip through the above code:
import pandas as pd
This line imports the 'pandas' library and assigns it the alias 'pd'. Pandas is used for data manipulation and analysis.
from sklearn.linear_model import LinearRegression
This line imports the 'LinearRegression' class from the scikit-learn (sklearn) library.
from sklearn.preprocessing import OneHotEncoder
This line imports the OneHotEncoder class from scikit-learn.
import joblib
This imports the joblib module in Python. joblib is a library that provides tools for Python to save and load Python objects.
data = pd.read_csv('dataset.csv')
This line reads a CSV file named 'dataset.csv' using pandas. It loads the data from the CSV file into a DataFrame called data.
X = data[['total_bill', 'day', 'time', 'size']]
y = data['tip']
We select specific columns from the data DataFrame and store them in another DataFrame called X. These columns ('total_bill', 'day', 'time', 'size') are our input features or clues. We extract the 'tip' column from the data DataFrame and store it in the variable y. This column represents our target variable or the answer we want to predict.
encoder = OneHotEncoder(sparse=False, drop='first')
Instance of the OneHotEncoder class is created with specific settings. sparse=False ensures that it doesn't produce a sparse matrix (a more memory-efficient but less readable format), and drop='first' removes the first category to avoid multicollinearity in the encoded features.
X_encoded = encoder.fit_transform(X[['day', 'time']])
We use the encoder to transform the 'day' and 'time' columns from our input DataFrame X into a numerical format. The result is stored in X_encoded.
feature_names = encoder.get_feature_names_out(['day', 'time'])
We obtain the feature names for the encoded columns, which is necessary for creating a DataFrame.
X_encoded_df = pd.DataFrame(X_encoded, columns=feature_names)
We create a new DataFrame called X_encoded_df from the encoded data, and we set the column names to be the feature names obtained from the encoder.
X = pd.concat([X.drop(['day', 'time'], axis=1), X_encoded_df], axis=1)
This line combines our original input features (X.drop(['day', 'time'], axis=1)) with the newly encoded features (X_encoded_df) along the columns (axis=1).
model = LinearRegression()
model.fit(X, y)
We create an instance of the LinearRegression model, which is a machine learning algorithm used for regression tasks. We train (fit) our LinearRegression model using the input features (X) and the target variable (y).
joblib.dump(model, 'tip_prediction_model.pkl')
This allows us to serialize and save Python objects to a file.
loaded_model = joblib.load('tip_prediction_model.pkl')
The joblib.load function allows us to deserialize and load Python objects from a file.
new_data = pd.DataFrame({
'total_bill': [40.00],
'day': ['Sun'],
'time': ['Dinner'],
'size': [4]
})
In this line, we create a new DataFrame new_data with a single data point. This data point has values for 'total_bill', 'day', 'time', and 'size', which we want to use to make a prediction.
new_data_encoded = encoder.transform(new_data[['day', 'time']])
new_data_encoded_df = pd.DataFrame(new_data_encoded, columns=feature_names)
new_data_final = pd.concat([new_data.drop(['day', 'time'], axis=1), new_data_encoded_df], axis=1)
We encode the categorical features ('day' and 'time') in our new data point new_data using the same encoder we used earlier. This ensures consistency in encoding between training and prediction. We create a DataFrame new_data_encoded_df to store the encoded values, and then we combine these encoded values with the other numerical feature ('size') from new_data to create a complete set of features for prediction, stored in new_data_final.
predicted_tip = loaded_model.predict(new_data_final)
Our trained LinearRegression model is used to make a prediction on the new data point new_data_final. This line calculates the predicted tip based on the features of new_data_final.
formatted_tip = "{:.2f}".format(predicted_tip[0])
This line of code formats the predicted tip value to have two decimal places and stores it as a formatted string.
print('Predicted Tip:', predicted_tip[0])
Finally, we print out the predicted tip, which is the result of our machine learning prediction. This is the estimated tip amount based on the given input features.
Comparison with Other Approaches
While our approach utilizes Linear Regression, other advanced techniques could be explored to further enhance prediction accuracy. Decision tree algorithms, for example, offer the ability to capture intricate interactions between variables, providing a more nuanced understanding of customer behavior. However, they might be prone to overfitting, especially with limited data. Deep learning models, such as neural networks, present an opportunity to analyze complex patterns within extensive datasets, potentially leading to more accurate predictions. Moreover, integrating Natural Language Processing (NLP) techniques could enable the analysis of customer feedback and sentiments, offering valuable insights into service quality and areas for improvement.
One key advantage of our approach is the ability to save and reuse the trained model for future predictions. By employing the joblib library, our model can be serialized and stored as 'tip_prediction_model.pkl'. This ensures that the restaurant staff can easily load the pre-trained model, enabling real-time tip predictions without the need for retraining.
Conclusion
By understanding and implementing machine learning techniques, restaurants can enhance customer experiences and optimize their services. Above is just one way of solving the problem, one may use various other machine learning techniques to tackle the challenge of predicting tips in restaurants. For instance, decision tree algorithms can capture complex interactions between different variables, offering a more nuanced understanding of customer behavior.
Incorporating deep learning models, such as neural networks, could provide the capability to analyze intricate patterns within vast datasets, leading to more accurate predictions. Similarly, using Natural Language Processing (NLP) techniques could lead to the analysis of customer feedback and sentiments, providing valuable insights into service quality and areas for improvement.