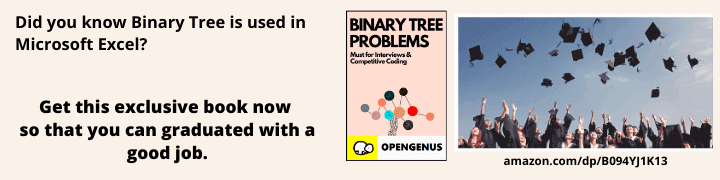
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 15 minutes
In this tutorial at OpenGenus, we will learn how to create or remove/ delete files and directories in Node.JS (JavaScript). We will use the filesystem module (fs) to achieve this.
Accessing file system is a common task but not with Javascript since it was meant to run in a browser. Although Html5 comes with a file system API but it is not fully supported in every browser yet. But installation of nodejs comes with a module which can help us to overcome our problem that is 'fs' or filesystem.
FileSystem module
Filesystem or 'fs' module acts as a wrapper or container to perform various files operations.Here we will see how to create and delete file/directories in synchronous and asynchrounous modes.You can further read about the module in file system.
How to access 'fs' or filesystem module in your program?
To include a module in a program one can use require keyword as shown below:
var fs = require('fs');
One more thing variable or constant should be of the same name as that of the module.So,with this line of code we can access all the built-in functions in 'fs' module.
Create and Delete files in your Javacript/NodeJS program
Since there are two modes synchronous and asynchrounous in which we can create and files so we will see them respectively.
But before that why don't we learn what do we mean by synchronous and asynchronous mode and it's impact on the process.
When you execute something or a code synchronously,you wait till it finishes before you go to the next task. When you execute something asynchronously you can move on to the next task before it finishes.
Now, it is basically means it that you can run multiple process at a same time without any problem in asynchronous mode but in synchronous only one process is executed at a time.
Now when we talk about synchronous and asynchronous mode in 'fs' module we mean that in synchronous mode first file/folder is created before any other task is executed whereas in asynchronous mode both the process creating of file/ folder or writing data or callback functions can execute simultaneously which we will see further down below.
Synchronous
1.Create a file Synchronously
Syntax:
fs.writeFileSync(file, data[,options])
- file: Name of the file
- data: String,array etc
Example:
fs.writeFileSync('mytext.txt',"hello world 3000!");
2.Delete file Synchronously.
Syntax:
fs.unlinkSync(path)
- path: Name of the file or location
fs.unlinkSync('mytext.txt');
Asynchronous
The only difference between Sync and Async is that in Async callback function is used.
1.Create a file Asynchronously.
Syntax:
fs.writeFile(file, data[,options], callback)
- file: Name of the file
- data: String,array etc
- callback: function
- err: error
Example:
fs.writeFile('myText.txt',"Hello NodeJS",(err) => {
if(err) throw err;
console.log("File has been saved!");
});
2.Delete file Asynchronously
Syntax:
fs.unlink(path, callback)
- path: Name of the file or location
- callback: function
- err: error
fs.unlink('myText.txt',(err) => {
if(err) throw err;
console.log('myText.txt was deleted');
});
Create and Delete Directories in your Javacript/ NodeJS program
Similar to Files directories or folders are created and deleted in synchronous and asynchronous modes respectively.
Synchronous
1. Create a directory Synchronously
Syntax:
fs.mkdirSync(path[, options])
- path: Name of the file or location
Example:
fs.mkdirSync('node');
2.Delete directory Synchronously.
Syntax:
fs.unlinkSync(path)
- path: Name of the file or location
fs.rmdirSync('node');
Asynchronous
1.Create a directory Asynchronously
Syntax:
fs.mkdir(path[, options], callback)
- path: Name of the file or location
- callback: function
- err: error
Example:
fs.mkdir('node',function(){
fs.writeFileSync('./node/myText.txt',"Hello World!!");
});
Here in the callback function we are creating a file right after the directory is created.
2. Delete directory Asynchronously
Syntax:
fs.unlink(path, callback)
- path: Name of the file or location
- callback: function
- err: error
fs.unlink('./node/myText.txt',function(){
fs.rmdir('node');
});
As it can be seen when we created the directory 'node' we also created a textfile 'myText.txt' so now to delete 'node' we first have to delete the 'myText.txt' then delete the directory.
Question
Which among the following is the syntax to create directory 'Text' in NodeJS in Synchronous mode?
With this article at OpenGenus, you have the complete idea of deleting files and directories in JavaScript/ Node.JS. Enjoy.