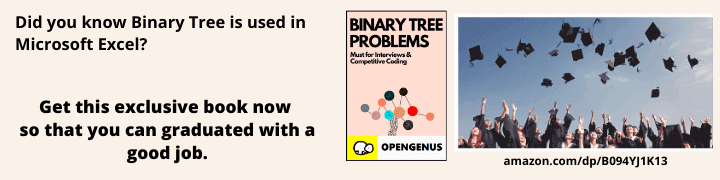
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 15 minutes
You all must be having basic idea of node.js if not I'd recommend you to read these article's first Introduction to Node.js and Working with Routing in Node.js.
In this article we'll be dealing with the files & perform basic operations such as Creating, Reading, Updating, Deleting & Renaming.One of the most basic APIs that Node provides is for the file system; With this API, we'll be coving all these operation's in this article.
⭐ Introduction
File System is a built-in Node.js module and can be imported into the project by including this line of code —
var fs = require('fs')
OR
var file = require('file-system');
file.readFile === fs.readFile // true
All the methods in File-system module have synchronous as well as asynchronous forms. Asynchronous methods take first parameter of the callback function as an error and the last parameter as the completion callback. Let’s see through an example -
The following example demonstrates reading existing Test.txt asynchronously.
var fs = require('fs'); //including file-system module
fs.readFile('Test.txt', function (err, data) {
if (err) throw err;
console.log(data);
//print the content of Test.txt file on console.
});
In the above example Test.txt file is read asynchronously and then callback function are executed when read operation is completed. The read operation either throws an error or completes successfully. The err parameter contains error information if any and the data parameter contains the content of the specified file.
Use fs.readFileSync() method to read file synchronously as shown below.
var fs = require('fs');
var data = fs.readFileSync('test.txt', 'utf8');
console.log(data);
Open your node terminal/command line interface, change the directory to the folder where the file is saved and run
node filename.js
node filename // or you can just write filename; it'll work same
You just read a file in Node.js.🎉
Now let's Create a File using Node.js.
⭐ Creating a new file
we can use fs.writeFile() method to write data to a file and If file already exist's then it overwrites the existing content otherwise it creates a new file and writes data into it OR we can use fs.open() method to create a new file.
let's go through both.
Syntax of fs.writeFile() is:
fs.writeFile(filename, data[, options], callback)
-
filename: name of the file including full path as a string.
-
Data: content to be written in the file.
-
options: options parameter can be an object or string which can include encoding, mode and flag.Default encoding and flag is utf8 and "r".Other flags that could be used here include -
1. r : Opens the file for reading.
2. r+ : Opens the file for reading and writing.
3. rs : Opens the file for reading in synchronous mode.
4. w : Opens the file for writing.
5. a : Opens the file for appending.
6. a+ : Opens the file for reading & appending
4. callback: A function with two parameters err and file descriptor.this function is called once write operation is completed.
var fs = require('fs');
fs.writeFile('test.txt', 'This is test file!', function (err) {
if (err) throw err;
console.log('File created');
});
In the above shown example, we’re using fs.writeFile() method to create a new file. The first argument it takes is name of the file which is test.txt file here. In second argument, we are adding contents to our file which we are creating.The function will throw error on console if any in our program otherwise you will see 'File created' on your console.
Remember, At the starting I told you that we can create file using fs.open().let's try it.
var fs = require('fs');
fs.open('test.txt','w',function(err) {
if(err) throw err;
console.log('File created!');
});
In the above example, we’re using fs.open() method to create a new file where first argument it takes is name of the file. In second argument, a flag has to be defined. If the flag is w for writing, the specified file is opened for writing.
So,Now let’s edit our created file & add a few lines into it before proceeding to check next operations.
This is test file!
we're learning about Node.js File System.
⭐ Updating a file
To update content of a file we use .appendFile() method.
Syntax of fs.appendFile() is:
fs.appendFile(filename,data,callback)
Now,let's see how we can update an existing file and append data to it.
var fs = require('fs');
fs.appendFile('test.txt','This line is our 3rd line.',(err) =>{
if (err) throw err;
console.log('Data is added in existing file');
});
we are using fs.appendFile() method to append data to the end of existing file.The result of above example will be:
This is test file!
we're learning about Node.js File System.
This line is our 3rd line.
Many of you might be thinking that why should we use .appendFile() when we have .writeFile(). So, how is .append() different from .writeFile() ? Well, in case of .appendFile(), we add content at end of the existing data. However, in case of .writeFile(), the existing content gets replaced with the one specified in its second argument.
⭐ Renaming a file
To rename a file we use .rename() method.
Syntax of fs.rename() is:
fs.rename(oldfilename, newFilename, callback)
Now let's see an example:
var fs = require('fs');
fs.rename('test.txt','newtest.txt',(err) =>{
if(err) throw err;
console.log('File successfully renamed!');
});
In the above example, we are renaming a file using .rename() method. The first argument it takes is name of the target file & as second argument, it takes the new name. This function successfully changes the filename from test.txt to newtest.txt.
⭐ Deleting a file
To delete a file we use .unlink() method.
Syntax of fs.unlink() is:
fs.unlink(filename, callback)
Now let's see an example:
var fs = require('fs');
fs.unlink('test.txt', (err) =>{
if(err) throw err;
console.log('File deleted successfully!');
});
In this example, we are deleting a file using .unlink() function. After the execution of this function, the specified file i.e test.txt is successfully deleted.
So, that’s all about the file system module & its basic operations. I hope I’ve made things simpler for you to understand.