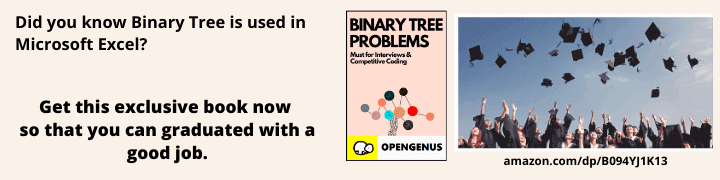
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
Set is a container that is used to store the values which are unique i.e no value in a set can be repeated and no value can be repeated. If you want to edit the value added by you the only way is to remove the wrong element and add the correct one.
The value stored in the set can be in ascending or desending order (with the help of greater function).
By default it is stored in ascending order.
For using set you need to mention either of the header files mentioned :
#include <bits/stdc++>
#include <algorithm>
#include <set>
#include <iterator>
Defining a set
A set is defined as follows:
std::set<datatype, comparison function> a;
- datatype can be a primitive datatype like int or a user defined type
- comparison function defines the sorting order of the datatype and is optional
Intializing set with int data type
The following example helps you to undestand how the set declared by you can be a type of int and give you the value stored in your set.
Code 1
//int set with int values
#include <iostream>
#include <set>
int main()
{
std::set<int> a;
a.insert(1);
a.insert(2);
a.insert(3);
for(auto& str: a) std::cout << str << ' ';
std::cout << '\n';
return 0;
}
Output
1 2 3
Inserting char instead of int
The following example store the value of char inserted with the help of ' ' and the set defined is int so the value printed is the ascii values.
Code 2
//int set with values in ' '
#include <iostream>
#include <set>
int main()
{
std::set<int> a;
a.insert('1');
a.insert('2');
a.insert('3');
for(auto& str: a) std::cout << str << ' ';
std::cout << '\n';
return 0;
}
Output
49 50 51
Intializing set with char data type
In the just above example we saw that when an int set gets a char value it prints the respective value so in the below example we have changed the type of set into char and hence get the desired output.
Code 1
//char set with char values
#include <iostream>
#include <set>
int main()
{
std::set<char> a;
a.insert('1');
a.insert('2');
a.insert('3');
for(auto& str: a) std::cout << str << ' ';
std::cout << '\n';
return 0;
}
Output
1 2 3
When do the vise versa of code 2 in the intializing set with int data type we get no result as char to int conversion is not possible in set.
Code 2
//char set with char values
#include <iostream>
#include <set>
int main()
{
std::set<char> a;
a.insert(1);
a.insert(2);
a.insert(3);
for(auto& str: a) std::cout << str << ' ';
std::cout << '\n';
return 0;
}
Output
//No output
Intializing set with array
Code 1
In the following example we assign the value of static array into a set. Since the array has a duplicate value hence the set only stores only one value.
Hence the output only have one value of each value in array.
#include <set>
#include <utility>
#include <iostream>
using namespace std;
int main()
{
static int const a[] = {3, 1, 4, 1, 5, 9, 2, 6, 5, 4};
set<int> const numbers( begin( a ), end( a ) );
for( auto const v : numbers ) { cout << v<<" "; }
cout << endl;
}
Output
1 2 3 4 5 6 9
Intializing set with string
In the following example we first declare a set with sting data type and store the value in set using " " and then print the value.
#include <iostream>
#include <set>
#include <string>
int main()
{
std::set<std::string> a;
a.insert("1");
a.insert("2");
a.insert("3");
for(auto& str: a) std::cout << str << ' ';
std::cout << '\n';
return 0;
}
Output
1 2 3
Intializing set with user defined function
In the following example with the help of a user defined class we compare the value in set and print them in descending order.
#include <iostream>
#include <set>
#include <string>
class comp
{
public :
template <typename T>
bool operator () (const T &l,const T&r)
{
return l>r;
}
};
int main()
{
std::set<int, comp> news = {1,2,3};
// note provided a custom comparison function
for(auto i : news)
{
std::cout<<i<<" ";
}
return 0;
}
Output
3 2 1