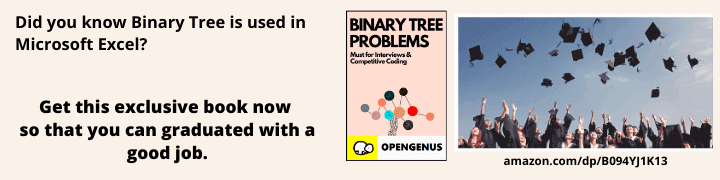
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
There are multiple ways to add two or more strings or a string and an integer in C++. We will explore each method is depth but the easiest approach is to use + operator as it is overloaded for strings in C++. To add integers with string, you will need to use to_string() as well.
The simplest approach will be as follows:
string string_data_3 = string_data_1 + string_data_2;
// OR
string string_data_3 = string_data_1 + to_string(integer_data_1);
We will explore the following techniques of adding strings in C++:
- Add two strings using + operator
- Add two strings using strcat method
- Add two strings using strncat method
- Add integer to string using to_string()
- Add two strings using append()
- Add two strings using format() in C++20
In C++, strings are defined in two different ways:
- Using string datatype in C++. It takes care of null terminating character at the end.
#include <string>
std::string data = "OpenGenus";
- Using character array
char data[] = "OpenGenus\0";
We can add strings depending upon the type of string it is.
Add two strings using + operator
The + operator can be used to add or concatenate two string objects. The important thing to note is that it is overloaded to handle only string objects and does not convert other types like Integer or character array to string. The basic syntax is as follows:
string data1 = "OpenGenus";
string data2 = "Foundation";
string data3 = data1 + data2;
The complete C++ code is as follows:
// Part of OpenGenus IQ
#include <iostream>
#include <string>
using namespace std;
int main()
{
string data1 = "OpenGenus";
string data2 = "Foundation";
string data3 = data1 + data2;
cout << data3;
return 0;
}
Output:
OpenGenusFoundation
One important point to note is that cannot use + on strings defined by quotes directly like:
string data = "OpenGenus" + "Foundation";
This will give the following error:
opengenus.cpp: In function 'int main()':
opengenus.cpp:7:33: error: invalid operands of types 'const char [10]' and 'const char [11]' to binary 'operator+'
string data = "OpenGenus" + "Foundation";
^
This is because the quotes are create an array of characters instead of a string. For this, you need to use the strcat or strncat functions.
Add two strings using strcat
strcat() is a function that concatenates two character arrays. It does not return anything but modifies the first array by attaching the second array to it. The syntax is:
strcat(char_array_1, char_array_2);
It will modify char_array_1 by attaching char_array_2 behind it. Note that both array must be null terminated that is should have null character (\0) as the last character.
Following is the complete C++ code to demonstrate this:
// Part of OpenGenus IQ
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
char data1[] = "OpenGenus\0";
char data2[] = "Foundation\0";
strcat(data1, data2);
cout << data1;
return 0;
}
Output:
OpenGenusFoundation
Add two strings using strncat
strncat() is similar to strcat() except that it will attach only the first N characters of the second string/ character array to the first array. N is provided by the user. The syntax is as follows:
strncat(char_array_1, char_array_2, N);
It will modify char_array_1 by attaching the first N characters of char_array_2 at the end of char_array_1.
Following is the complete C++ code to understand the use of strncat():
// Part of OpenGenus IQ
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
char data1[] = "OpenGenus\0";
char data2[] = "Foundation\0";
strncat(data1, data2, 5);
cout << data1;
return 0;
}
Output:
OpenGenusFound
Add integer to string using to_string()
To add integer to a string, we need to convert the integer to a string. This can be done using the to_string() method of string utility/ header file. Once done, we can use the + operator to add an integer to a string.
Syntax:
string data1 = "OpenGenus";
string data2 = data1 + to_string(1);
Following is the complete C++ code to illustrate the process:
// Part of OpenGenus IQ
#include <iostream>
#include <string>
using namespace std;
int main()
{
string data1 = "OpenGenus";
string data2 = data1 + to_string(1);
cout << data2;
return 0;
}
Output:
OpenGenus1
Add two strings using append()
append() function can be used on strings to attach another string at the end of the original string. It does not modify the original strings but returns the modified string.
Syntax:
string data1 = "OpenGenus";
string data2 = "Foundation";
string data3 = data1.append(data2);
Following is the complete C++ code example:
// Part of OpenGenus IQ
#include <iostream>
#include <string>
using namespace std;
int main()
{
string data1 = "OpenGenus";
string data2 = "Foundation";
string data3 = data1.append(data2);
cout << data3;
return 0;
}
Output:
OpenGenusFoundation
Add two strings using format() in C++20
format() is a new feature available in C++20 and hence, it will not work in earlier versions of C++. It gives flexibility of format strings based on requirements and the syntax is clean and similar to Python. This can be used for several cases including adding strings.
Syntax:
string data1 = "OpenGenus";
string data2 = "Foundation";
string data3 = format("{} {}", data1, data2);
Following is the complete C++ code example:
// Part of OpenGenus IQ
#include <iostream>
#include <string>
using namespace std;
int main()
{
string data1 = "OpenGenus";
string data2 = "Foundation";
string data3 = format("{} {}", data1, data2);
cout << data3;
return 0;
}
Output:
OpenGenus Foundation
With this, you have the complete knowledge of adding or concatenating two or more strings together in C++.
You need not to confused about which method to chose as the simple approach would be to use the + operator and to ensure greater control with clean implementation, you may use the format() method in C++20 and onwards.
If you have any doubts, you may comment down below (👇) and our C++ experts will get back to you and clarify your doubts.