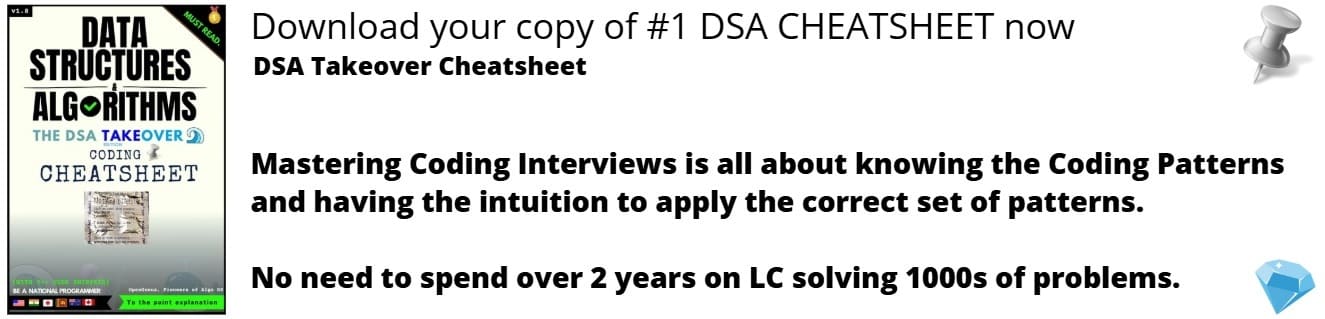
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Engineer and author Martin Fowler said, "Any fool can write code that a computer can understand. Good programmers write code that humans can understand." Coding best practices are a set of informal rules that the software development community employs to help improve software quality. Here, are a few common practices that will help make your code more readable and maintainable.
Best Coding Practices are:
- Keep your code simple and efficient
- Write test cases
- Perform static code analysis
- Adhere to style guides and naming conventions
- Use meaningful names
- DRY (Don't Repeat Yourself) principle
- Write portable code
- Make your code scalable
- Save your work!
We will explore these points deeper now.
Keep your code simple and efficient
Complicated logic should be kept to a minimum since it may not make sense to another programmer or even you in the future! "Simplicity" is not merely the number of lines of code, but of logical and conceptual structure as well; making code shorter may make it less or more complex. For example, consider these code snippets.
if (hours < 24 && minutes < 60 && seconds < 60)
return true;
else
return false;
and
return hours < 24 && minutes < 60 && seconds < 60;
The first approach whilst consuming more screen space would be easily understood by even a novice programmer. The second approach minimizes the size but increase the complexity and may cause problems due to operator precedence.
Write test cases
The number of lines, complexity of the logic implemented, etc won't matter if your program doesn't work so test your code! Make sure that your code is able to give the correct output and can handle errors and exceptions. Verify that your program works for edge cases such as 0s, empty strings/lists, nulls, etc. The use of automated testing tools can help validate builds & releases and make code more supportable.
Perform static code analysis
Static code analysis is a method of debugging code before executing it. This type of analysis addresses weaknesses (detection of overflow, syntax violations, etc) in the source code that might lead to vulnerabilities. Automated code analysis tools such as Embold, SonarQube, etc, provide a huge advantage in speed, depth & accuracy over manual code reviews.
Adhere to style guides and naming conventions
Follow the style guide provided by your organization or programming language which includes details abut indentation, placing of braces and spaces, naming of identifiers (for instance, using lower camel case for variable names) and much more.
In case no guide is provided, ensure that the style you adopt is consistent across all your source code files, to ensure better readability for other users. Platforms like AStyle (Artistic Style), Uncrustify, etc offer code formatting & beautification services across various programming languages.
Also, ensure that naming conventions are followed. For e.g. the 'pandas' package in Python can be imported as 'panda', 'pds', etc, but it is typically imported as 'pd'. Importing it by another name may cause confusion and require more effort from others reading your code.
Use meaningful names
The need for comments may actually be reduced if variables, methods, classes, etc are named properly instead of giving obvious comments. Use descriptive, meaningful names that are able to specify the purpose of the function or variable. For example,
// Here, we calculate the area using length & breadth
double a = l * b;
The above code can be rewritten as:
double area = length * breadth;
No need for comments, a programmer will be able to understand the aim of the code intuitively! The same can be repeated for functions as well. All IDEs also include auto-complete as an inbuilt function, so it takes away the effort of typing out and remembering long identifier names. Also avoid using a single identifier for multiple purposes.
If comments are required, ensure that they are clear and precise in documenting the task of the code.
DRY (Don't Repeat Yourself) principle
Automate repetitive tasks whenever necessary. If you find that a particular code (say, to accept user input) is being repeated in your script, convert it into a function (for e.g. acceptUserInput()) and ensure that all function calls to obtain input from the user is being directed to that it. This enhances the reusability of the code and make it easier to test and find bugs in the program. On a similar note, if there are a large amount of methods providing similar functionality, it could be better to create a separate class to handle it. This ensures better cohesion between the methods of a class and is in accordance with the Single-responsibility principle.
Write portable code
Your code should be able to run accurately and in the same manner across different environments; different processors, operating systems, compilers etc. An example of non-portable code is when a C++ pointer is assumed to be of 4 bytes. However, this is only true for 32-bit systems as the size of a pointer is 8 bytes in a 64-bit system.
Make your code scalable
Your program should be written in a way that allows your application to be scaled both vertically (scale up) and horizontally (scale out) efficiently. This can be achieved by having low resource requirements, mitigating bottlenecks by distributing workloads and computing in parallel, using decentralized databases and more.
Save your work!
Save and backup your code regularly to ensure that your hard work is not lost! Version control softwares like Git allow you to maintain and track changes to your code over time. Verify that commit messages, file names, etc are able to accurately summarize the changes made and is in the required format when contributing to any repository.
Hope you found this article at OpenGenus useful! Although these coding practices are not concrete rules, anyone working with your code (including yourself!) will appreciate the effort, and might even learn from your example :)