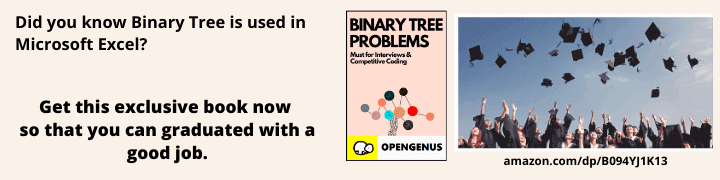
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
C++ uses the concept of streams to perform I/O operations. A stream is a sequence of bytes in which character sequences are 'flown into' or 'flow out of'. Streams operate as the intermediate between the program and the I/O devices thus helping the programmers to achieve device independent I/O operations. The two types of streams in C++ are:
- input stream
- output stream
Headerfiles available in C++ for Input/Output Operations :
- iostream - iostream stands for standard input-output stream. This header file contains definitions to objects like cin, cout, cerr etc.
- iomanip - iomanip stands for input output manipulators. The methods declared in this files are used for manipulating streams. This file contains definitions of setw, setprecision etc.
- fstream - This header file describes the file stream. This header file is used to handle the data being read from a file as input or data being written into the file as output.
The objects being discussed in this article are cin and cout. They are the most commonly used objects for taking inputs and printing outputs.
Standard output stream (cout)
cout is an object of the class ostream. The standard output device is the display screen. The characters are inserted into the output stream. The insertion operator (<<) is used along with cout to insert values into the stream so that the output device (monitor) can use them.
Usage
#include<iostream>
using namespace std;
int main()
{
int Hello=10;
cout<<"Hello"; //This prints the text "Hello" on the display screen.
cout<<Hello ; // Prints the contents of the variable 'Hello'(10) on the screen.
cout<<10; //Prints number 10 on the screen
}
Multiple insertion operations (<<) can be chained in a single statement:
cout<<"Welcome"<<"to"<<"OpenGenus!";
Output
Welcome to OpenGenus!
Chaining multiple insertions is useful to mix literals and variables in a single statement:
cout<<"My name is "<<name<<" and I am "<<age<<"years old";
Assuming the variable name is initialised with 'Alice' and age with 25, the output of the previous statement would be :
My name is Alice and I am 25 years old
The cout operator does not add linebreaks add the end of an output. To add a linebreak, the newline character ('\n') has to be used.
cout<<"This is sentence 1.\n";
cout<<"This is sentence 2.";
Output
This is sentence 1.
This is sentence 2.
The same thing can also be achieved using endl manipulator instead of the new-line character.
cout<<"This is sentence 1."<<endl;
cout<<"This is sentence 2.";
This produces the same output as above.
Note - While both the newline character and endl produce the same output, the key difference between the two is that endl causes a flushing of the output buffer every time it is called, whereas '\n' does not.
Output Formatting :
Formatting the output is an important part of any application, to improve the user interface and enhance the readability.
Formatting in C++ is done through the use of I/O manipulators. Most of these manipulators are available in iostream and iomanip.
The standard manipulators are :-
-
endl - Introduces a line break on the output stream similar to '\n'. The example was seen above .
-
setw() - The setw() manipulator sets the width of the field assigned for the output. It takes the size of the field (in number of characters) as argument.
Example :
#include<iostream.h>
#include<iomanip.h>
void main()
{
cout<<setw(6)<<"X";
}
Output
_ _ _ _ _X
When chaining multiple insertion operations, the setw() manipulator does not stick from one operation to the next. To set the width for each operation, setw() has to be repeated.
Example :
cout<<setw(3)<<1<<"\n"<<setw(3)<<2<<"\n"<<setw(3)<<3;
Output :
_ _1
_ _2
_ _3
- setprecision() - The setprecision() manipulator sets the total number of digits to be displayed when floating-point numbers are printed.
Example :
cout<<setprecision(5)<<22.0/7;
Output
3.1415
The setprecision() manipulator can also be used to set the number of decimal places to be displayed. To do this, you have to set an ios flag.
cout.setf(ios::fixed);
cout<<setprecision(5)<<22.0/7;
Output
3.14159
Note - Unlike setw() , all the subsequent couts after setprecision() retain the precision set with the last setprecision(). That means setprecision() is "sticky".
- Additional IOS flags :
flag | meaning |
---|---|
left | left-justify the output |
right | right-justify the output |
showpoint | displays decimal point and trailing zeros for all |
all floating point numbers, even if decimal places | |
are not required | |
showpos | display a plus sign before positive values |
scientific | display floating point numbers in scientific notation |
* Standard input stream (cin) :
cin is an object of the class istream . The standard input device is they keyboard. When the user enters characters via the keyboard, the keycode is placed in the input stream. The extraction operator ( >> ) is used with cin to extract values from the stream.
Usage
#include <iostream>
using namespace std;
void main ()
{
int i;
cout << "Please enter an integer value: ";
cin >> i;
cout << "The value you entered is " << i;
}
Output
Please enter an integer value: 12
The value you entered is 12
Like the insertion operator, extraction operator can also be chained in a single statement.
cin >> x >> y;
This is the equivalent of
cin>>x;
cin>>y;
In both cases, the user must provide two values separated by any blank separator (space, tab or newline).
Using cin with strings
cin can be used to read strings the same way it is used with other fundamental data types. However, cin always considers any white space character as terminating the value being extracted. So extracting a string with cin means extracting only a word and not a sentence.
To overcome this, a function called getline() can be used that takes the stream (cin) as first argument, and the string variable as second.
Example :
void main()
{
string myname;
cout <<"Enter your name: ";
getline (cin, myname);
cout<<"Welcome "<<myname;
}
Output
Enter your name: Alice Cooper
Welcome Alice Cooper
Another way to use getline with cin :
cin.getline(char buffer, int length) - Reads a stream of characters into the string buffer. It stops when it has read length-1 characters or when it finds an end-of-line character ('\n').
Example :
void main()
{
char address[20];
cout << "Address: ";
cin.getline(address, 20);
cout<<"You live in "<<address;
}
Output
Address: Baker Street, UK
You live in Baker Street, UK
Apart from getline(), cin can also be used with other member functions. Some of them include :
funtion | meaning |
---|---|
cin.get(char &ch) | Reads an input character and store it in ch |
cin.read(char buffer, int n) | Reads n bytes from the stream into the buffer |
cin.ignore(int n): | Ignores the next n characters from the stream |
cin.eof(): | Returns a nonzero value if end of file is reached |
With this article at OpenGenus, you must have the complete idea of Cin and Cout in C++. Enjoy.