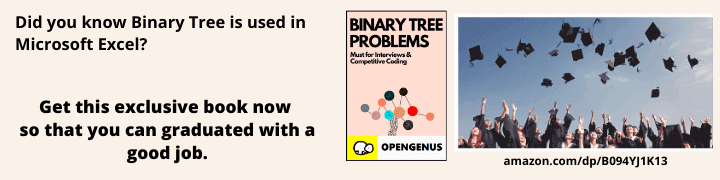
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to learn how to develop an application that performs the CRUD operations in MySQL database using hibernate. Here we will develop a command line application but the same procedure can be used to develop any other type of application like GUI tool for MySQL, webapp etc.
Prerequisites
The learner
- should be familiar with basics of Java
- should have Java installed on their respective machine
- should have MySQL database installed
- should have a modern IDE like Eclipse, IntelliJ Idea etc.
- should have an active internet connection
Steps to create the CRUD app
Step 1: Create a maven project
Step 2: Add the required dependencies
Step 3: Create a model class
Step 4: Create Java Class for hibernate configuration
Step 5: Create a doa layer class to handle CRUD operations
Step 6: Develop CLI to test the application
Note: I am using eclipse IDE over here but any modern IDE of your choice can be used to follow along. I'll provide the steps to perform the task in the eclipse IDE.
Step 1: Create a maven project
To create a maven project in eclipse ide follow the following steps-
- Go to File > New > Project..
- Type maven in the filter box, select Maven Project and click next
- Click next
- In catalog dialog select 'internal' and select 'maven-archetype-quickstart' from the list of archetypes and click next
- Type the values for 'Group Id', 'Artifact Id' and 'Package' and click finish
Wait for the project to be created.
By default the maven associates the project with 'Java 1.5'. So, the eclipse IDE will complain to you for this. To remove the warning given by the eclipse IDE, open up the pom.xml file and paste the following code inside properties element.
<maven.compiler.source>17</maven.compiler.source>
<maven.compiler.target>17</maven.compiler.target>
Since, I have Java 17 installed on my machine I have used 17 here. You use the java version installed on your machine.
Step 2: Add the required dependencies
Add the following dependencies in the pom. xml file under dependencies element.
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-entitymanager</artifactId>
<version>5.6.9.Final</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.29</version>
</dependency>
The first dependency brings all the required jar files that we need for hibernate, including hibernate-core, hibernate-common-annotations and others.
The second dependency brings a java jdbc connector of type-4 driver of MySQL database.
Step 3: Create a model class
Create User.java under com.gaurav.model package
package com.gaurav.model;
import java.util.Date;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long id;
private String firstname;
private String lastname;
@Temporal(TemporalType.DATE)
private Date dob;
public User() {
super();
}
public User(String firstname, String lastname, Date dob) {
super();
this.firstname = firstname;
this.lastname = lastname;
this.dob = dob;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getFirstname() {
return firstname;
}
public void setFirstname(String firstname) {
this.firstname = firstname;
}
public String getLastname() {
return lastname;
}
public void setLastname(String lastname) {
this.lastname = lastname;
}
public Date getDob() {
return dob;
}
public void setDob(Date dob) {
this.dob = dob;
}
@Override
public String toString() {
return "User [id=" + id + ", firstname=" + firstname + ", lastname=" + lastname + ", dob=" + dob + "]";
}
}
Step 4: Create Java Class for hibernate configuration
Create a class HibernateConf.java under com.gaurav.util package.
package com.gaurav.util;
import java.util.Properties;
import org.hibernate.SessionFactory;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.cfg.Configuration;
import org.hibernate.cfg.Environment;
import org.hibernate.service.ServiceRegistry;
import com.gaurav.model.User;
public class HibernateConf {
private static SessionFactory factory;
private HibernateConf() {}
public static SessionFactory getFactory() {
if(factory == null) {
Configuration configuration = new Configuration();
Properties settings = new Properties();
settings.put(Environment.DRIVER, "com.mysql.cj.jdbc.Driver");
settings.put(Environment.URL, "jdbc:mysql://localhost:3306/tutorial");
settings.put(Environment.USER, "root");
settings.put(Environment.PASS, "root");
settings.put(Environment.DIALECT, "org.hibernate.dialect.MySQL8Dialect");
settings.put(Environment.SHOW_SQL, "false");
settings.put(Environment.HBM2DDL_AUTO, "update");
configuration.setProperties(settings);
configuration.addAnnotatedClass(User.class);
ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder()
.applySettings(configuration.getProperties())
.build();
factory = configuration.buildSessionFactory(serviceRegistry);
}
return factory;
}
}
Remember to replace the values of USER and PASS to your database credentials. In my case it is 'root' and 'root' respectively. Create a database tutorial in mysql database or replace tutorial with the name of another database.
Step 5: Create a doa layer class to handle CRUD operations
For the shake of following the standards we first create a contract or an interface that holds the methods to be implemented.
Create UserDao.java interface under com.gaurav.dao package.
package com.gaurav.doa;
import java.util.List;
import com.gaurav.model.User;
public interface UserDao {
void saveUser(User user);
User getUserById(long id);
List<User> getAllUsers();
void updateUser(User user);
void deleteUserById(long id);
}
Now, create an implementation class UserDaoImpl.java under com.gaurav.dao package.
package com.gaurav.doa;
import java.util.List;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import com.gaurav.model.User;
import com.gaurav.util.HibernateConf;
public class UserDaoImpl implements UserDao {
private SessionFactory factory = HibernateConf.getFactory();
@Override
public void saveUser(User user) {
Transaction transaction = null;
try(Session session = factory.openSession()) {
transaction = session.beginTransaction();
session.save(user);
transaction.commit();
} catch (Exception e) {
if(transaction != null)
transaction.rollback();
}
}
@Override
public User getUserById(long id) {
Transaction transaction = null;
User user = null;
try(Session session = factory.openSession()) {
transaction = session.beginTransaction();
user = session.get(User.class, id);
transaction.commit();
} catch (Exception e) {
if(transaction != null)
transaction.rollback();
}
return user;
}
@Override
@SuppressWarnings("unchecked")
public List<User> getAllUsers() {
Transaction transaction = null;
List<User> users = null;
try(Session session = factory.openSession()) {
transaction = session.beginTransaction();
users = session.createQuery("from User").list();
transaction.commit();
} catch (Exception e) {
if(transaction != null)
transaction.rollback();
}
return users;
}
@Override
public void updateUser(User user) {
Transaction transaction = null;
try(Session session = factory.openSession()) {
transaction = session.beginTransaction();
session.saveOrUpdate(user);
transaction.commit();
} catch (Exception e) {
if(transaction != null)
transaction.rollback();
}
}
@Override
public void deleteUserById(long id) {
Transaction transaction = null;
try(Session session = factory.openSession()) {
transaction = session.beginTransaction();
User user = session.get(User.class, id);
session.delete(user);
transaction.commit();
} catch (Exception e) {
if(transaction != null)
transaction.rollback();
}
}
}
The above implementation class is responsible for all the crud operation in the database.
Now, to test the application we are going to develop a commandline application.
Step 6: Develop CLI to test the application
There must be a file App.java under com.gaurav package. Just write the below code in the same file.
package com.gaurav;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.sql.Date;
import com.gaurav.doa.UserDao;
import com.gaurav.doa.UserDaoImpl;
import com.gaurav.model.User;
public class App
{
public static void main( String[] args )
{
UserDao dao = new UserDaoImpl();
BufferedReader input = new BufferedReader(new InputStreamReader(System.in));
while(true) {
try {
System.out.println("==================MENU=================");
System.out.println("1. Create a new user");
System.out.println("2. See a user");
System.out.println("3. See all the users");
System.out.println("4. Update a user information");
System.out.println("5. Delete a user");
System.out.println("6. Exit");
System.out.print("Enter your choice: ");
int choice = Integer.valueOf(input.readLine());
switch(choice) {
case 1:
{
System.out.print("Enter the firstname: ");
String firstname = input.readLine().trim();
System.out.print("Enter the lastname: ");
String lastname = input.readLine().trim();
System.out.println();
System.out.println("Dob format will be yyyy-mm-dd");
System.out.print("Enter the dob: ");
String date = input.readLine().trim();
User user = new User(firstname, lastname, Date.valueOf(date));
System.out.println("\nAdding the user.........");
dao.saveUser(user);
System.out.println("User added successfully!");
break;
}
case 2:
{
System.out.print("Enter the userId: ");
long id = Long.valueOf(input.readLine());
System.out.println(dao.getUserById(id));
break;
}
case 3:
{
dao.getAllUsers().forEach(u -> System.out.println(u));
break;
}
case 4:
{
System.out.println("Enter the userId: ");
long id = Long.valueOf(input.readLine());
User user = dao.getUserById(id);
if(user == null) {
System.out.println("Sorry! The user does not exit.");
break;
}
System.out.println("Leave blank if don't want to change.");
System.out.print("Enter the firstname: ");
String firstname = input.readLine().trim();
if(firstname != "")
user.setFirstname(firstname);
System.out.print("Enter the lastname: ");
String lastname = input.readLine().trim();
if(lastname != "")
user.setLastname(lastname);
System.out.println();
System.out.println("Dob format will be yyyy-mm-dd");
System.out.print("Enter the dob: ");
String date = input.readLine().trim();
if(date != "")
user.setDob(Date.valueOf(date));
System.out.println("\nUpdating the user.........");
dao.updateUser(user);
System.out.println("User updated successfully!");
break;
}
case 5:
{
System.out.println("Enter the userId: ");
long id = Long.valueOf(input.readLine());
System.out.println("Deleting the user.......");
dao.deleteUserById(id);
System.out.println("User deleted successfully!");
break;
}
case 6:
System.exit(0);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
Now, right click in the App.java, go to Run As > and run it as java application. Now you should be able to see the below output:
Now, try performing all the operations in this application.
Happy Coding!🙂🙂🙂