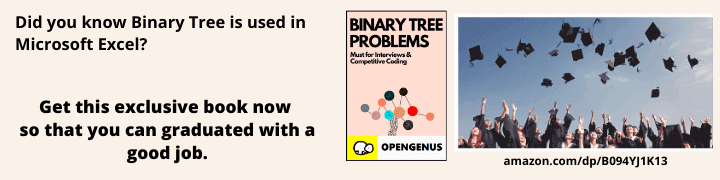
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will learn about 3 different ways to delete element from set in C++ STL. This will involve using methods like erase and find.
A set is a container which contains unique elements in a sorted order.
There are different ways to delete element from set in C++. Some of them are mentioned below:
- Method 1: Using the erase() function to delete a single element
- Method 2: Using the erase() function to delete a range of elements
- Method 3: Using the find() function and the erase() function
Method 1: Using the erase() function to delete a single element
- If we have to delete a single element from the given set, pass that element as a parameter to the erase() function.
Following is the complete C++ code example demonstrating how to delete a range of elements in a set using erase():
// Part of iq.opengenus.org
#include <iostream>
#include <set>
using namespace std;
int main() {
set<int> s = {11, 12, 13, 14, 15, 16, 17, 18};
// Print the elements of the set
for (auto it : s) {
cout << it << " ";
}
cout << endl;
// Delete element '12' from the set
s.erase(12);
// Print the remaining elements of the set
for (auto it : s) {
cout << it << " ";
}
cout << endl;
return 0;
}
Output:
//initial elements in the set
11 12 13 14 15 16 17 18
//after deleting a single element
11 13 14 15 16 17 18
//after deleting starting 3 elements from the set
15 16 17 18
Method 2: Using the erase() function to delete a range of elements
- To delete a range of elements, we have to pass the range as a parameter.
Following is the code snippet:
auto it1 = s.begin();
auto it2 = s.begin();
//incrementing it2 3 times
it2++;
it2++;
it2++;
// To delete starting 3 elements from the set
s.erase(it1, it2);
Following is the complete C++ code example demonstrating how to delete a range of elements in a set using erase():
// Part of iq.opengenus.org
#include <iostream>
#include <set>
using namespace std;
int main() {
set<int> s = {11, 12, 13, 14, 15, 16, 17, 18};
// Print the elements of the set
for (auto it : s) {
cout << it << " ";
}
cout << endl;
auto it1 = s.begin();
auto it2 = s.begin();
//incrementing it2 3 times
it2++;
it2++;
it2++;
// To delete starting 3 elements from the set
s.erase(it1, it2);
// Print the remaining elements of the set
for (auto it : s) {
cout << it << " ";
}
cout << endl;
return 0;
}
Method 3: Using the find() function and the erase() function
We can use the find() method to search for the element which we have to delete, and then we can use the erase() method to remove it.
Following code snippet explains the idea:
auto it = s.find(15);
s.erase(it);
Following is the complete C++ code example demonstrating how to delete elements in a set using find() and erase():
#include <iostream>
#include <set>
using namespace std;
int main() {
set<int> s = {11, 12, 13, 14, 15, 16, 17, 18};
// Print the elements of the set
for (auto it : s) {
cout << it << " ";
}
cout << endl;
// find element 15 in the set
auto it = s.find(15);
// delete element 15 from the set if it exists
if (it != s.end()) {
s.erase(it);
}
// Print the remaining elements of the set
for (auto it : s) {
cout << it << " ";
}
cout << endl;
return 0;
}
Output:
//initial elements in the set
11 12 13 14 15 16 17 18
//after deletion of the element 15 from the set
11 12 13 14 16 17 18
With this article at OpenGenus, you must have the complete idea of how to delete elements in a set in C++ STL.