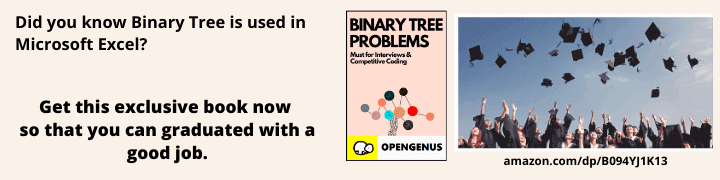
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Eager Loading and Over-eager loading are methods of loading resources to web pages during creation of DOM Nodes.
Contents
- Three Types of Loading
- Eager Loading
- Over-Eager Loading (Preloading)
- MIME Types
- CORS-enabled fetches
- Responsive Preloading
- Scripting Preloads
- Usage
Three Types of Loading
Webpages consist of HTML pages along with several other resources like stylesheets, scripts, images, and video.
Loading these resources can be done synchronously when a page is loaded, asynchronously before a page is loaded, or asynchronously after a page is loaded.
The three types of loading are:
- Eager Loading: is the event where all server resources are loaded after initialization of the DOM.
- Over-eager loading (preloading): is similar to eager loading, except the developer anticipates which resources to load in the future and preloads them.
- Lazy Loading: is the opposite of over-eager loading, and defers loading resources for DOM elements until a condition is met, such as an element entering the viewport requiring the given resource.
This article will focus on implementing over-eager loading, since eager loading is the default behavior of most webpages and frameworks.
Eager Loading
The default behavior of the browser is to eagerly load resources.
When a request for a page is made like example.com/index.html
, the page usually contains links to other resources on the server to load. These resources typically include stylesheets, scripts, and hypermedia.
The page will prioritize creating HTML DOM nodes first, then it will load resources in the order of the <link>
or <script>
tags.
<head>
<link rel="stylesheet" href="Home.module.css"/>
<script type="module" src="index.js"/>
</head>
In the above example, Home.module.css
is loaded first, then index.js
after. Note that by loading, we mean that the HTML tells the browser to send an HTTP request for a specific resource on a remote server to then load onto the client computer. The <link>
,<script>
and any other resource tags do this either after the DOM is created (eager and lazy loading) or before the DOM is created (over-eager loading).
Over-Eager Loading (Preloading)
We can implement over-eager loading by preloading requested resources ahead of time.
HTML allows us to preload these resources natively in <link>
tags or their resource specific tags.
The basic syntax is:
<link rel="preload" href="resource/file/path">
Preloading is advantageous for scripts or stylesheets that contain links to other resources or make fetch requests. This allows the browser to prioritize making web requests to pull the required data before the HTML is rendered.
The following types of data can be preloaded:
- audio: Audio file, as typically used in
<audio>
. - document: An HTML document intended to be embedded by a
<frame>
or<iframe>
. - embed: A resource to be embedded inside an
<embed>
element. - fetch: Resource to be accessed by a fetch or XHR request, such as an ArrayBuffer or JSON file.
- font: Font file.
- image: Image file.
- object: A resource to be embedded inside an
<object>
element. - script: JavaScript file.
- style: CSS stylesheet.
- track: WebVTT file.
- worker: A JavaScript web worker or shared worker.
- video: Video file, as typically used in
<video>
.
MIME Types
<link>
elements can accept type
attributes which can contain the MIME type of the resource you are loading. This is useful when preloading as the browser can check if a resource is supported and ignore it if it isn't.
Below is an example of using type
to preload a supported video/mp4
and possibly unsupported video type video/webm
:
<head>
<meta charset="utf-8">
<title>Video preload example</title>
<link rel="preload" href="sintel-short.mp4" as="video" type="video/mp4">
</head>
<body>
<video controls>
<source src="sintel-short.mp4" type="video/mp4">
<source src="sintel-short.webm" type="video/webm">
<p>Your browser doesn't support HTML5 video. Here is a <a href="sintel-short.mp4">link to the video</a> instead.</p>
</video>
</body>
This enables browsers to swap between resources depending on client side conditions.
CORS-enabled fetchs
CORS enables servers to indicate locations other than the current server to where locations are located. It allows requests to be redirected from one server to another to get the same requested resource.
In these situations, it is important to specify the cross-origin
tag so that servers know to redirect requests in cases where a resource isn't located in the requested server but another.
This often comes up in resources like:
- scripts that use
fetch()
orXMLHttpRequest
- fonts
The basic syntax is:
<link rel="preload" href="resource/file/path" crossorigin>
Responsive Preloading
Using media
tags, we can responsively load images.
<link rel="preload" href="bg-image-narrow.png" as="image" media="(max-width: 600px)">
<link rel="preload" href="bg-image-wide.png" as="image" media="(min-width: 601px)">
<script>
var mediaQueryList = window.matchMedia("(max-width: 600px)");
var header = document.querySelector('header');
if (mediaQueryList.matches) {
header.style.backgroundImage = 'url(bg-image-narrow.png)';
} else {
header.style.backgroundImage = 'url(bg-image-wide.png)';
}
</script>
Scripting Preloads
Preload tags can be scripted into elements like the following:
var preloadLink = document.createElement("link");
preloadLink.href = "myscript.js";
preloadLink.rel = "preload";
preloadLink.as = "script";
document.head.appendChild(preloadLink);
Then the preload can be executed:
var preloadedScript = document.createElement("script");
preloadedScript.src = "myscript.js";
document.body.appendChild(preloadedScript);
Usage
It is recommended that:
- Eager loading be used for small size applications where features can be immediately loaded on start.
- Lazy loading be used for large applications to load features that won't be used immediately on start for faster startup times.
- Over-eager loading be used for features that are used immediately on startup of an application, for example a Login page.
With this article at OpenGenus, you must have the complete idea of Eager Loading and Over-Eager Loading.