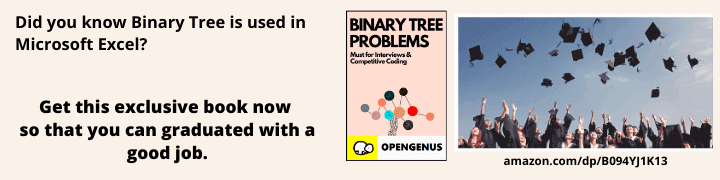
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In C++, endl and /n are used to break lines or move the cursor to a new line. Both endl and \n may seem to be similar but has distinct differences which we have explored in this article in depth.
endl
endl stands for end line. endl is a predefined object of ostream class . it is a manipulator used to insert a new line and flush the output buffer.
cout<<endl;
Example 1:
#include<iostream>
using namesapce std;
void main(){
cout<<"HELLO"<<endl<<"WORLD";
}
Output:
HELLO
WORLD
In the above example, HELLO is displayed first and then using endl we terminate the first line and then WORLD is displayed in the second line.
\n
\n is a newline character in c++. it is also used for inserting a new line.
cout<<"\n";
Example 2:
#include<iostream>
using namespace std;
void main(){
cout<<"I\nAM\nA\nPROGRAMMER";
}
output:
I
AM
A
PROGRAMMER
In the above c++ code we use escape sequence \n or the newline character to terminate the line therfore I ,AM, A, PROGRAMMER all four are displayed in different lines.
To insert two lines one after the other we can use two \n characters.
Example 3:
#include<iostream>
using namespace std;
void main(){
cout<<"Open\n\nGenus";
}
output:
Open
Genus
In this example, we use two newline characters to insert two newlines.
Difference between endl and \n
both endl and \n do the same thing that is inserts a new line however there are some differences between them:
- endl is used to insert a new line and flush the stream but \n only inserts a new line.If we don't want the buffer to be flushed frequently, we use '\n'.
- we can write \n in double quotations as in example 2 and 3 but we cannot write endl in double quotations.
- endl is slow but “/n” is fast for new line creation as it requires less processing.
endl and the idea of Flushing
endl when written to an output stream adds a new line and flushes the buffer.By flushing the buffer, we ensure that the user will see the output written to the stream immediately.
When we use cout, the operand used after the output operator ( << ) are stored in a buffer and are not displayed until it comes across endl cin, which causes the buffer to be flushed, in the sense, display/output the contents of the buffer onto the stdin.
so when we place endl in an output stream, it is equivalent to placing a new-line character in the stream, and then calling flush().
Consider the two examples below:
#include <iostream>
using namespace std;
int main()
{
for (int i = 1; i <= 5; ++i)
{
cout << i <<"/n";
}
cout << endl;
return 0;
This c++ code will output the numbers 1 2 3 4 5 (all in different lines) at once.
#include <iostream>
using namespace std;
int main()
{
for (int i = 1; i <= 5; ++i)
{
cout << i << endl;
}
return 0;
}
This c++ code will print the numbers 1 2 3 4 5 (all in different lines) one by one.
by the above two examples we understand that endl in addtion to inserting a new line flushes the stream but /n only inserts a new line.
Why we should avoid using endl?
endl not only inserts a new line but also flushes the stream each time.
flushing is an expensive operation thus decreasing the overall performance in case of large number of i/o tasks.
We really dont need to flush the buffers after few lines of code as it gets automatically flushed when the buffer is full.
therefore using ‘\n’ also called the new line character is more efficient as it does not flush the buffer like endl.