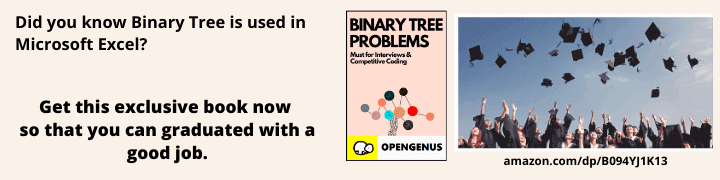
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
Enumeration (or enum) is a user defined data type in C and C++. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain.
C++ allows programmer to define their own function.A user-defined function groups code to perform a specific task and that group of code is given a name(identifier).
When the function is invoked from any part of program, it all executes the codes defined in the body of function.
Synatx :
enum enum_name{constant1, constant2, constant3, ....... };
NOTE : Like in array we have index number here also the constant have index number.
Examples
An example program to demonstrate working of enum in C++
#include<iostream.h>
enum week{Mon, Tue, Wed, Thur, Fri, Sat, Sun};
int main()
{
enum week day; //calling enum
day = Wed;
cout<<day;
return 0;
}
Output :
2
In the above example, we declared âdayâ as the variable and the value of âWedâ is allocated to day, which is 2. So as a result, 2 is printed.
Another example program to demonstrate working of enum in C++
#include<iostream.h>
enum year{Jan, Feb, Mar, Apr, May, Jun, Jul,Aug, Sep, Oct, Nov, Dec};
int main()
{
int i;
for (i=Jan; i<=Dec; i++)
printf("%d ", i);
return 0;
}
Output :
0 1 2 3 4 5 6 7 8 9 10 11
In this example, the for loop will run from i = 0 to i = 11, as initially the value of i is Jan which is 0 and the value of Dec is 11.
Interesting fact about enum
- Two enum names can have same value. For example, in the following C program both âFailedâ and âFreezedâ have same value 0.
#include <iostream.h>
enum State {Work = 1, Fail = 0, Freez = 0};
int main()
{
cout<<Work<<Fail<<Freez;
return 0;
}
Output :
1, 0, 0
- If we do not explicitly assign values to enum names, the compiler by default assigns values starting from 0.
For example, in the following C++ program, sunday gets value 0, monday gets 1, and so on.
#include <iostream.h>
enum day {sunday, monday, tuesday, wednesday, thursday, friday, saturday};
int main()
{
enum day d = thursday;
cout<<"The day number stored in d is :"<<d;
return 0;
}
Output:
The day number stored in d is : 4
- We can assign values to some name in any order. All unassigned names get value as value of previous name plus one.
#include <iostream.h>
enum day {sunday = 1, monday, tuesday = 5,wednesday, thursday = 10, friday, saturday};
int main()
{
cout<<sunday<<monday<<tuesday<<wednesday<<thursday<<friday<<saturday;
return 0;
}
Output :
1 2 5 6 10 11 12
-
The value assigned to enum names must be some integeral constant, i.e., the value must be in range from minimum possible integer value to maximum possible integer value.
-
All enum constants must be unique in their scope. For example, the following program fails in compilation.
enum state {working, failed};
enum result {failed, passed};
int main()
{
return 0;
}
Output :
Compile Error: 'failed' has a previous declaration as 'state failed'