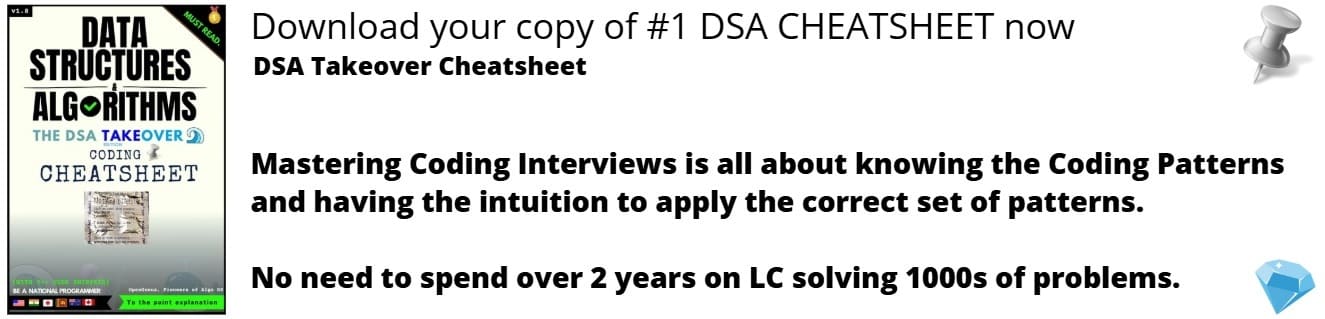
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Flask is a web framework that is widely used for building web applications in Python due to its simplicity, flexibility, and easy-to-use API, making it a popular choice for web developers. The application structure of Flask is considered important as it enables the code to be organized by developers and aids in the creation of scalable and maintainable web applications. Code readability, maintainability, and the ease of incorporating new features into the application are improved by a well-structured Flask application. Therefore, understanding and implementing an appropriate Flask application structure is deemed key to building successful Flask applications. We have covered this in depth in this article at OpenGenus.
Table of contents:
- Basic Outline of Flask Application
- Best Practices to develop Flask Applications
Basic Outline of Flask Application:
The basic structure of a Flask application is composed of several files and directories that work together to create a functioning web application.
The following elements comprise the basic structure of a Flask application and are as follows:
- Main application file: This file sets up the Flask app object, which is responsible for processing incoming requests and generating responses. This file is typically named app.py or application.py and is located in the root directory of the application. In this example, we have named our project flask_app and the main file as app.py.
- Templates: Templates are used to generate dynamic content in Flask applications and are typically stored in a subdirectory named templates within the application directory.
- Static files: Static files such as images and CSS files are commonly used in Flask applications to add functionality and styling. These files are usually stored in a subdirectory named static within the application directory.
- Other files: Other files may be necessary to maintain its structure, such as configuration files or files used to establish connections to databases. Additional files such as readme files and requirements files may also be included in the application directory.
These files are based on the complexity of the application you are building.
Main Application File:
The main application file is the heart of a Flask application. This file typically contains the following components:
- Importing modules: Import any relevant Python modules and Flask extensions that may be required by the application.
- Creating the app instance: Create the Flask app object and configure it as required for the application's use.
- Defining application routes: Specify the routes for the application, which determine how incoming requests are handled and what responses are returned to the user.
- Handling errors: Implementing error handlers to handle any errors or exceptions that may arise during the execution of the application.
Below is the simple example of Flask application, it will print "Hello, World!" on the web.
from flask import Flask # imports Flask from module named flask
app = Flask(__name__) # creation of new instance of flask class
@app.route("/") # it is a decorator which is used to define what URL will
# trigger the function 'hello_world' which is defined under
# it.
def hello_world(): # this function will be called when user visits the URL.
return "<h1>Hello, World!</h1>"
if __name__=="__main__": # '__name__ defines the current module, if it is the main module of application then it will run else not
app.run(host='127.0.0.1', port=8000, debug=True) # this will start application, it defines the IP address of host on which it will run, and other parameters also.
Templates:
In Flask applications, templates are used to display dynamic content to users. These templates are typically created using HTML, and they can include Jinja2 code to incorporate dynamic data into the HTML. Flask applications store their templates in the "templates" subdirectory within the main application directory. To render a template in a Flask application, developers can use the "render_template()" function, which accepts the name of the template file as an input
In the given example, you can see that templates sudirectory contains two files 'base.html' and 'index.html'.
Static Files:
To enhance the appearance and functionality of Flask applications, developers often use static files like images and CSS files. These files are usually located in the "static" subdirectory within the application directory. To reference a static file within a Flask application, the url_for() function can be utilized, which generates a URL for the static file.
We have two files 'main.css' and 'style.css' in the Static subdirectory as shown in the given example.
Other Files:
Other files that the flask application might use are listed as follows:
- Modules: In Flask applications, it is typical to interact with databases or data stores. To represent the data in these stores, developers create Python classes known as modules. These modules provide an interface to interact with the data, enabling the creation, reading, updating, and deletion of data as required.
- Forms: Web applications frequently need to gather data from users, and Flask-WTF is a prominent extension that streamlines the process of building forms in Flask. By defining a form as a Python class, it is simple to produce HTML form elements, validate user input, and manage form submissions.
- Configuration: Flask applications usually require various settings to be configured, such as the connection string for the database or the secret key used for encryption. These settings can be saved in a configuration file, making them easy to manage and update as required.
- Blueprints: As Flask applications expand in size and complexity, it can be challenging to maintain all of the routes and views in a single file. Blueprints provide a solution by allowing you to organize your routes and views into modular components that can be registered with the main application, making it easier to manage your code and reuse code across multiple applications.
- Extensions: Flask offers a diverse range of extensions that provide additional functionality for your application. Using extensions can save you time and allow you to focus on developing the core features of your application.
In the given example, it shows a subdirectory '.vscode' having extension file as extensions.json, configuration file as settings.json and blueprint file as tasks.json.
Best Practices to develop Flask Applications:
Given are the some best practices for organizing and structuring Flask applications:
-
Adopting a modular approach: To make the application more manageable, break it down into smaller, modular components. Each module should have its own routes and templates.
-
Utilizing Flask Blueprints: Using Flask Blueprints is a great way to make the application more scalable and modular. By breaking down the application into modular components, you can manage it more efficiently.
-
Separating logic and views: To improve the code's readability and maintainability, it's a good idea to separate the logic and views of the application into separate files.
-
Storing application-specific settings in a configuration file: To make the application more configurable, it's essential to store application-specific settings in a configuration file. This file can contain information such as database connection details.
-
Using a version control system: To keep track of the changes made to the application's codebase, use a version control system such as Git. This system makes it easier to manage the codebase, track changes, and collaborate with other developers.
With this article at OpenGenus, you must have the complete idea of Structure of a Flask application.