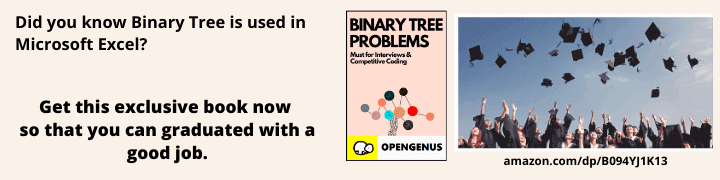
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes | Coding time: 2 minutes
Often, i++ and i=i+1 are considered to be same statements but in Java, both statements work differently internally. We will take a look at:
- The compiler instructions used
- Typecasting along with the operations
- benchmarking the two operations
We eventually see that i=i+1 is always faster than i++.
Compiler Instructions
As both i = i+1 and i++ are different commands, both uses different JVM instructions in bytecode.
i+1 uses iadd instruction
i++ uses iinc instruction
The exact command depends on the type of compiler being used.
Typecasting
In the expression i=i+1, as we are adding 1 an integer, if i is of lower precision such as byte or short, it will give error: incompatible types: possible lossy conversion.
Example (with the error):
class opengenus
{
public static void main (String[] args)
{
short i = 1;
i = i + 1; // gives compilation error
System.out.println(i);
}
}
Output:
error: incompatible types: possible lossy conversion from int to short
i = i + 1;
^
1 error
Correct code with explicit type casting:
class opengenus
{
public static void main (String[] args)
{
short i = 1;
i = (short)(i + 1); // compiles correctly
System.out.println(i);
}
}
Output:
2
This problem does not take place in the expression i++ as typecasting is handled internally by the compiler
Example:
class opengenus
{
public static void main (String[] args)
{
short i = 1;
i++; // compiles correctly
System.out.println(i);
}
}
Output
2
Hence, while replacing i=i+1 by i++, we need to consider the type of i as well.
Question
Depending upon above points, which should be faster?
Benchmark
To measure the performance of the two similar statements, we used the following Java code:
import java.lang.Math;
class benchmark_opengenus
{
public static void main (String[] args)
{
int i=0, limit = 1000000;
long t1_1 = System.nanoTime();
for(int j=0; j<=limit; j++)
{
i++;
}
long t1_2 = System.nanoTime();
long t2_1 = System.nanoTime();
for(int j=0; j<=limit; j++)
{
i=i+1;
}
long t2_2 = System.nanoTime();
System.out.println("i++ took "+(t1_2-t1_1)+ " nanoseconds");
System.out.println("i=i+1 took "+(t2_2-t2_1)+ " nanoseconds");
System.out.println("i=i+1 took "+ String.format("%.2f",(double)(t2_2-t2_1)*100/(t1_2-t1_1))+" % of time taken by i++");
}
}
Output:
i++ took 4041400 nanoseconds
i=i+1 took 2676134 nanoseconds
i=i+1 took 66.22 % of time taken by i++
Hence, we see i = i+1 is nearly 40% faster than i++.