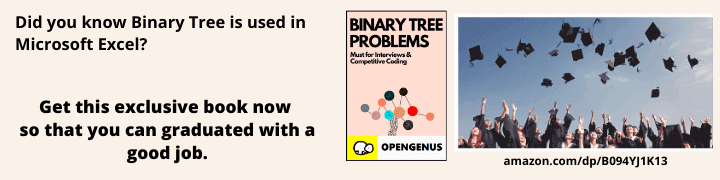
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
In Java, goto is a reserved word but it has not implemented in Java. Hence, we do not have a same goto keyword/ feature as in other languages like C and C++.
Key points for Java:
- Java does not support goto but it is a reserved word
- Java does support labels
- Java does support continue and break which use label
Why goto is not supported?
In software engineering practices, it is strongly advised not to use goto as it increases the code complexity greatly.
In legacy code, it has been seen that the use of goto has made it difficult for new developers for developing new features. For this, Java developers skipped added goto functionality but added it is a reserved keyword so it is possible to added such a feature later if required.
So, currently, it is not possible to use goto but one can develop a external library to implement this feature of goto using another statement such as goto2. This is possible as Java does support limited program flow control using:
- labels
- continue
- break
and the compiler infrastructure supports explicit program flow control.
Currently, there is not such production level library.
If we try to use goto in Java, we will get a compile time error like:
class OpenGenus
{
public static void main (String[] args)
{
loop: System.out.println("1");
if (true) continue loop;
System.out.println("2");
}
}
Compile time error:
prog.java:6: error: illegal start of expression
if (true) goto loop;
^
prog.java:6: error: not a statement
if (true) goto loop;
^
2 errors
As goto is a reserved word, one cannot use it as an identifier. In case, we try to do so, we will get a compile time error like:
class OpenGenus
{
public static void main (String[] args)
{
int goto = 2;
}
}
Compile time error:
prog.java:5: error: not a statement
int goto = 2;
^
prog.java:5: error: ';' expected
int goto = 2;
^
2 errors
Implementing similar feature
We can use break statement to skip some code statements by enclosing code statements in a labeled code block.
Example:
class OpenGenus
{
public static void main (String[] args)
{
loop: {
System.out.println("1");
if (true) break loop;
System.out.println("2");
}
System.out.println("3");
}
}
Output:
1
3
We can use a continue statement in a limited way to deal with loops:
class OpenGenus
{
public static void main (String[] args)
{
loop:
for(int i=0; i< 3; i++)
{
if (i==1)
continue loop;
System.out.println(i);
}
System.out.println("--");
}
}
Output:
0
2
--