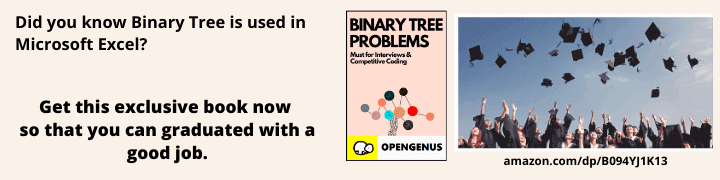
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
The continue statement is a program flow control statement in Java. It is used to skip the current loop iteration and switch to the next loop iteration.
Instead of forcing termination like break, it forces the next iteration of the loop to take place, skipping any code in between. Continue can be seen as a restricted version of goto statement.
Syntax
The syntax of using continue statement in Java is as follows:
<label>;
// some loop like for loop
...
continue <label>;
- label is optional
- if label is not present, continue will transfer control to the next loop iteration of the inner most loop
- if label is present just before a loop, continue will transfer control to the next loop iteration of the concerned loop
Working of continue
Consider the following simple example of using continue without any label:
class OpenGenus
{
public static void main (String[] args)
{
System.out.println("a");
for(int i=1; i<4; i++)
{
if(i==2)
continue;
System.out.println(i);
}
}
}
Output:
a
1
3
Note that wehn i == 2 is encountered, the control goes to the next iteration that is i=3 and 2 is never printed.
Understanding label with continue statement
Consider this example of 2 nested loops each with its own label ("loop1", "loop2") and a label "beforeloop" which is not just before a loop. In the initial version, the continue statement is with no specified label:
class OpenGenus
{
public static void main (String[] args)
{
beforeloop:
System.out.println("a");
loop1:
for(int i=1; i<3; i++)
{
loop2:
for(int j=1; j<3; j++)
{
System.out.println(i+"."+j);
if(i==2 || j==2)
continue;
}
}
}
}
Output:
a
1.1
1.2
2.1
2.2
If we specify the label of the inner most loop with continue statement, it will be same as no label.
continue loop2;
Complete code:
class OpenGenus
{
public static void main (String[] args)
{
beforeloop:
System.out.println("a");
loop1:
for(int i=1; i<3; i++)
{
loop2:
for(int j=1; j<3; j++)
{
System.out.println(i+"."+j);
if(i==2 || j==2)
continue loop2;
}
}
}
}
Output:
a
1.1
1.2
2.1
2.2
In this example, we will set the label to loop1 and in this case, the control will go back to the first loop and the last iteration (i=2 and j=2) will be skipped completely.
continue loop1;
Complete code:
class OpenGenus
{
public static void main (String[] args)
{
beforeloop:
System.out.println("a");
loop1:
for(int i=1; i<3; i++)
{
loop2:
for(int j=1; j<3; j++)
{
System.out.println(i+"."+j);
if(i==2 || j==2)
continue loop1;
}
}
}
}
Output:
a
1.1
1.2
2.1
If we try to use a label not associated with a label, we will get a compilation error denoting that the label has not been found.
continue beforeloop;
Complete code:
class OpenGenus
{
public static void main (String[] args)
{
beforeloop:
System.out.println("a");
loop1:
for(int i=1; i<3; i++)
{
loop2:
for(int j=1; j<3; j++)
{
System.out.println(i+"."+j);
if(i==2 || j==2)
continue beforeloop;
}
}
}
}
Error:
prog.java:15: error: undefined label: beforeloop
continue beforeloop;
^
1 error
We will get the same error if the label is not for a loop which includes the concerned coontinue statement.
Comparison of continue with break, goto
- Break
break control statement transfers the control to the statement just after the immediate loop and terminates the loop immediately.
On the other hand, continue statement does not terminate the loop but only skips the current iteration with the remaining code statements and goes to the next iteration.
Consider the following code example with 2 loops differing only in one statement using continue in one and break in another:
class OpenGenus
{
public static void main (String[] args)
{
System.out.println("With Break");
for(int i=1; i<4; i++)
{
System.out.println(i);
if(i==2)
break;
}
System.out.println("With Continue");
for(int i=1; i<4; i++)
{
System.out.println(i);
if(i==2)
continue;
}
}
}
Output:
With Break
1
2
With Continue
1
2
3
- goto
Continue can be seen as a special case of goto. With goto, we can transfer program control to any part of the code but in case of continue, it is limited to the concerned loops.