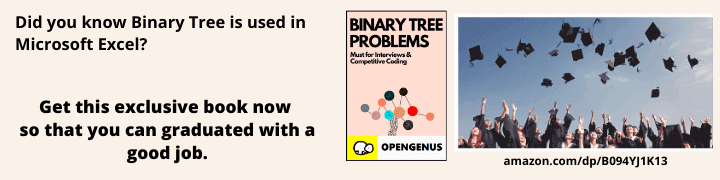
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Content
- Introduction
- Causes
- Limitation of resources
- Bad synchonization mechanisms
- Resource contention
- Poorly Designed Algorithms
- Operating System Limitations
- Effects
- Reduced Throughput
- Increased latency
- CPU Utilization
- Deadlocks
- Resource Starvation
- Scalability issues
- Solutions
- Fine grained locking
- Lock-free algorithms
- Reader-writer locks
- Spinlocks
- Lock Stripping
- Avoiding unnecessary locking
- Non-preemptive scheduling
- Changing Thread priorities
Imagine a canal with few ships and small boats. Ships are much bigger and heavier so it will take some time for them to cross the canal while the smaller boats are much less heavier and faster so they can cross faster. But if ships will cross the canal, boats need to wait as the pathway will be occupied by the ships for some time. This is a real life example of Lock Convoy Problem.
This may sound similar to DeadLock situation but this problem is different than Lock Convoy Problem. In DeadLock problem, two or more threads are blocked for a resource and waiting for each other to release it in order to proceed. But at the end resource lock isn't released by either so it results in system freeze and its unable to make any progress at all.
While, on the other hand in Lock Convoy Problem, multiple threads are waiting to acquire a lock on a shared resource which is being held by another thread. So they need to wait for the busy thread to release the lock. Meanwhile more threads gets added to the waiting forming a convoy. But unlike Deadlock situation, eventually thread gets released and progress is made. But each threads requires waiting depending the thread currently holding the lock on resource. This leads to degraded problem and increases latency problems.
Another real life example can be multiple cars and vehicles passing through a narrow tunnel around same time. This will lead to congestion and delay . Eventually they will be blocked for some time until vehicles pass through the tunnel.
The Lock Convoy Problem typically occurs in multi-threaded and distributed systems where multiple processes are running concurrently and need to access the same resource at the same time. The resource can anything from files,folders to huge databases and core system components.
Causes
Limitation of Resources
Limitation of resources can cause the lock convoy problem.If the resources are limited, the processes might try to access the same resource such as database,file,documents etc. so that might lead to lock convoy problem. Because now the processes need to wait for the current process to release the lock so that will lead to delay and degraded performance. Total waiting time will keep on increasing with the upcoming resource access requests which will make a convoy of requests.
Bad Synchonization Mechanisms
Usually synchonization mechanisms are implemented to ensure that not more than one process access a resource at the same time which may lead to deadlock or lock convoy problem. But it depends on the implementation of the mechanism. If the implementation is faulty, it will lead to lock convoy, deadlock and other locking problems.
Resource Contention
Resource contention occurs when there are too many process running and competing for the same shared resources and resources are not enough for each process, this will make processes compete with each other for resources leading to lock convoy problem.
Poorly Designed Algorithms
Poorly Designed Algorithms also affect the sharing and assignment of resources and can cause lock convoy problem if a resource needs to be access frequently. For example if an algorithm needs to needs to update the database record at every few intervals, and if other processes will also try to update it, that will lead to live lock convoy problem and may lead to inconcurrent updation of database.
Operating System Limitations
Operating system also matters in case of resource allotment and sharing. The operating system may have a restriction for concurrent processes or threads that can run on the system simultaneously. If these limits are exceeded, it will also lead to lock convoy problem.
Effects
This problem can cause significant delays and reduce the overall efficiency of the system. Here are some of the effects of the lock convoy problem in computers:
Reduced throughput
When multiple processes are waiting for access to a shared resource, they may be forced to wait in a queue until the resource becomes available. This can cause significant delays and reduce the overall performance of the system.
Increased latency
The longer a thread or process has to wait for a lock, the higher its latency will be. This can lead to slower response times and reduced user satisfaction. Processes will need to wait too much to get a lock on resource leading to degraded performance.
CPU utilization
When threads are waiting for locks, they may continue to consume CPU resources even though they are not making progress. This can lead to wasted CPU cycles and reduced overall system performance.
Deadlocks
A deadlock occurs when each computer process waits for a resource that is being assigned to another process. In this case, none of the processes get performed since the resource they require is being held by another process that is simultaneously waiting for another resource to be released. In some cases, the lock convoy problem can lead to deadlocks, where two or more processes are waiting for each other to release resources before they can proceed. This can cause the entire system to become unresponsive and require a reboot.
Resource starvation
If one process is holding onto a shared resource for an extended period of time, other processes may be unable to access it and suffer from resource starvation. This can lead to reduced performance and even crashes in some cases.
Scalability issues
The lock convoy problem becomes more severe as the number of threads or processes increases. This can limit the scalability of the system and prevent it from handling larger workloads. As soon the system will be scaled, issue will get worse and convoy will become even longer.
Solutions
Fine-grained locking
One way to mitigate the lock convoy problem is to use fine-grained locking, which involves breaking down a large lock into smaller locks that can be acquired independently by different threads. This approach reduces contention and allows for more parallelism.
Lock-free algorithms
Lock-free algorithms address this problem by using techniques such as atomic operations, compare-and-swap (CAS), and memory barriers to ensure that multiple threads can access shared data structures without interfering with each other. By eliminating the need for locks, lock-free algorithms can improve performance and scalability in multi-threaded applications.
However, designing and implementing lock-free algorithms can be challenging, as they require careful consideration of issues such as memory consistency, race conditions, and thread synchronization.
Reader-writer locks
Reader-writer locks allow multiple threads to read a shared resource simultaneously but only one thread can write at a time. Reader-writer locks can help reduce contention and improve performance in situations where there are many more reads than writes. However, they may not be suitable for all scenarios and may require careful tuning and testing to ensure optimal performance.
Spinlocks
Spinlocks are another solution that involves busy waiting instead of blocking when a thread attempts to acquire a lock that is already held by another thread. While this approach can improve performance in some cases, it can also lead to increased CPU usage and contention if not used carefully.
Lock striping
Lock stripping involves breaking down a single large lock into smaller locks that are more granular and specific to different parts of the system. This allows multiple threads or processes to access different parts of the system simultaneously without having to wait for each other, improving overall performance and reducing contention for locks.
Avoiding unnecessary locking
Finally, it's important to avoid unnecessary locking whenever possible by minimizing the amount of code that requires exclusive access to shared resources and using immutable data structures whenever possible.
Non-Preemptive scheduling
Non-preemptive scheduling means that once a process or thread is given access to a shared resource, it retains that access until the process or thread voluntarily releases it. By doing so, other threads or processes do not have to wait for the lock to be released and can continue with their tasks without delays. Non-preemptive scheduling can be advantageous in situations where there are a few long-running processes, and the likelihood of contention is relatively low. However, this approach does have its limits, and it does not work well in scenarios where there are many short-lived processes or where the resource is locked for an extended period.
Changing thread priority
One solution to lock convoy problem is to change the priority of threads. By assigning different priority to threads, the operating system ensures only the thread which requires the resource first gets access only. This is advantageous in situations that involves lots of threads and some important thread can get stuck in waiting in lock convoy problem. However, it is important to note that changing the priority of threads may not always be effective in solving the Lock Convoy Problem, and it can lead to other issues. For instance, when a high-priority thread is given access to a shared resource, it may not release the resource for a long time, causing lower-priority threads to wait excessively, thus affecting the overall system performance.
In conclusion of this article at OpenGenus, there are several solutions available for mitigating the lock convoy problem in computer systems, including fine-grained locking, lock-free algorithms, reader-writer locks, spinlocks, lock striping, and avoiding unnecessary locking. The best solution depends on the specific scenario and requirements of the system being developed or optimized.