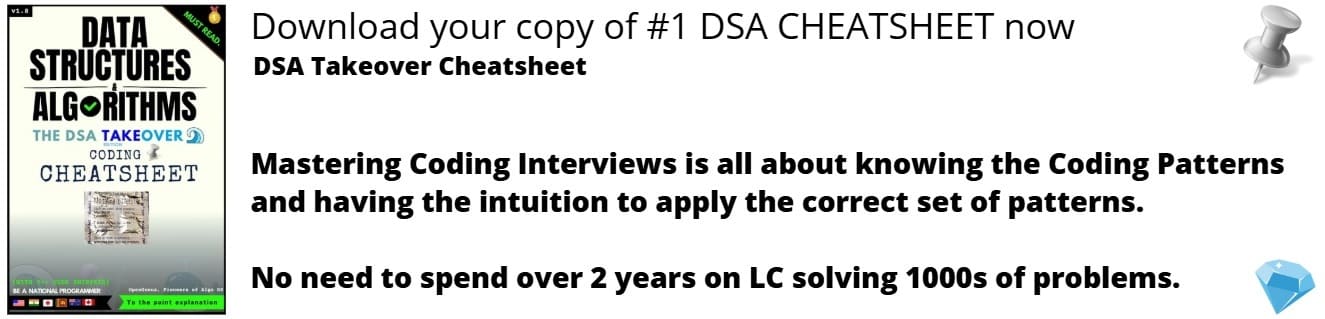
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will be creating a simple "Note Taking App in Python" with a Basic GUI and Database Integration.
Table of Contents:
- Introduction
- Setting Up the Development Environment
- Creating the Main Window
- Adding a Text Box for Note Input
- Implementing the Save Button and Database Integration
- Adding a Button to View Saved Notes
- Running the Application
- Conclusion
Introduction:
Building graphical user interfaces (GUIs) is one of the many uses for the flexible programming language Python. In this article, we'll look at how to build simple Python note-taking application with a simple graphical user interface (GUI) and database integration. Users of our application will be able to record notes, save them to a database, and browse their recorded notes. You will have a working application that combines GUI functionality with database administration by the conclusion of this article.
This app uses the SQLite database and the functions for interacting with it. The database stores the notes, and the model provides methods to save new notes and retrieve existing notes.
Setting Up the Development Environment:
Setting up our development environment is the first step. If you haven't already, install Python and make sure you have the required packages, including Tkinter for the graphical user interface and a database library like SQLite or MySQL.
pip install tk
Creating the Main Window:
Import the necessary modules, then use the Tkinter library to build the app's main window. Set the geometry and window title.
import tkinter as tk
# Create the main window
window = tk.Tk()
window.title("Note Taking App")
window.geometry("400x300")
Adding a Text Box for Note Input:
Create a text box where users can input their notes. Use the Tkinter Text
widget to pack it into the main window.
# Create a text box
note_entry = tk.Text(window)
note_entry.pack()
Implementing the Save Button and Database Integration:
Integrate a database to hold the notes and include a button that enables users to save their notes. Create a function that extracts the text box's contents, connects to the database, and stores the note there.
import sqlite3
# Create a save button and database integration
def save_note():
note = note_entry.get("1.0", tk.END)
conn = sqlite3.connect("notes.db")
cursor = conn.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS notes (id INTEGER PRIMARY KEY AUTOINCREMENT, content TEXT)")
cursor.execute("INSERT INTO notes (content) VALUES (?)", (note,))
conn.commit()
conn.close()
save_button = tk.Button(window, text="Save Note", command=save_note)
save_button.pack()
To handle database activities, the'sqlite3' library is imported in the code above. TheSave_note()
method creates a connection to the SQLite database and obtains the note's content from the text field. If the "notes" table doesn't already exist, we build it before inserting the note into the database using a SQL query. Finally, we close the database connection and commit the modifications.
Adding a Button to View Saved Notes:
Add a button that searches the database for saved notes and displays them. Create a function that retrieves the notes, opens a new window to present them in, and displays the notes using the Text
widget.
# Adding a button to view saved notes
def view_notes():
conn = sqlite3.connect("notes.db")
cursor = conn.cursor()
cursor.execute("SELECT * FROM notes")
notes = cursor.fetchall()
conn.close()
view_window = tk.Toplevel(window)
view_window.title("View Notes")
view_text = tk.Text(view_window)
for note in notes:
view_text.insert(tk.END, note[1] + "\n")
view_text.pack()
view_button = tk.Button(window, text="View Notes", command=view_notes)
view_button.pack()
The view_note()
method, which uses a SQL query to fetch all the notes from the database, is defined in the code above. Using the Toplevel
class, we build a new window and use the 'Text' widget to fill it with the obtained notes.
Running the Application:
Calling the mainloop()
function adds the code necessary to run the program. The Tkinter event loop is executed via this method, enabling user interaction and updating the GUI.
# Run the application
window.mainloop()
Conclusion:
This article shows how to mix GUI features with database administration, offering new opportunities for creating more complex applications. Users have the option of entering their notes, saving them to a database, and seeing those notes later. The Tkinter toolkit for Python and its database connectivity packages offer a strong framework for building flexible applications.
FULL IMPLEMENTATION
import tkinter as tk
import sqlite3
# Create the main window
window = tk.Tk()
window.title("Note Taking App")
window.geometry("400x300")
# Create a text box
note_entry = tk.Text(window)
note_entry.pack()
# Create a save button and database integration
def save_note():
note = note_entry.get("1.0", tk.END)
conn = sqlite3.connect("notes.db")
cursor = conn.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS notes (id INTEGER PRIMARY KEY AUTOINCREMENT, content TEXT)")
cursor.execute("INSERT INTO notes (content) VALUES (?)", (note,))
conn.commit()
conn.close()
save_button = tk.Button(window, text="Save Note", command=save_note)
save_button.pack()
# Adding a button to view saved notes
def view_notes():
conn = sqlite3.connect("notes.db")
cursor = conn.cursor()
cursor.execute("SELECT * FROM notes")
notes = cursor.fetchall()
conn.close()
view_window = tk.Toplevel(window)
view_window.title("View Notes")
view_text = tk.Text(view_window)
for note in notes:
view_text.insert(tk.END, note[1] + "\n")
view_text.pack()
view_button = tk.Button(window, text="View Notes", command=view_notes)
view_button.pack()
# Run the application
window.mainloop()