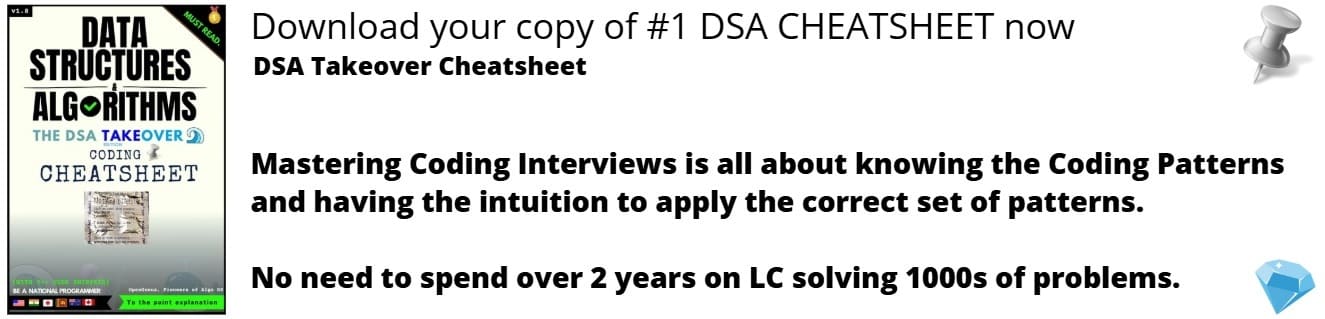
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered how to pass a vector to a function as function agrument in C++ with variants like 1D vector, 2D vector, 3D vector, global vector and global vector by value. We have also, covered how to return a vector from a function.
Table of contents:
- Passing a 1D vector to the Function
- Passing a 2D Vector to the function
- Passing a 3D vector to the Function
- Passing a global vector to the function
- Passing a global vector by value
- How to return a Vector from a function?
Passing a 1D vector to the Function
In C++ arrays are passed to a function with the help of a pointer, which points to the starting element of the array. Hence any changes made to the array in the function are reflected in the original array.
Pass by Value
However, when passing a vector to function as an argument the case is not the same. Here by default a copy of the original vector is created and is used in the function. Hence any changes made to the vector in the function do not reflect upon in the original vector.
This can be shown by the example given below:-
CPP Code
#include <bits/stdc++.h>
using namespace std;
// creating a function to pass the vector by value to the function
void func( vector <int> duplicate) { // the original vector is copied into the vector duplicate
// let us reduce every even number in the vector by 1
int l = duplicate.size(); // storing the size of vector duplicate in l
// reducing every even number in duplicate by 1
for(int i = 0; i < l; i++){
if(duplicate[i]%2 == 0)
duplicate[i]--;
}
// displaying the modified vector
cout<<"Modified vector in the function-->"<<endl;
for(int i = 0;i < l;i++)
cout<<duplicate[i]<<" ";
cout<<endl;
}
int main(){
vector <int> original = {1,2,3,4,5,6,7,8,10}; // creating the original vector
func( original ); // calling the function func
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++)
cout<<original[i]<<" ";
return 0;
}
Output:-
Modified vector in the function--> 1 1 3 3 5 5 7 7 9 Original vector is--> 1 2 3 4 5 6 7 8 10
Pass by Reference
When we pass a vector by reference, it does not pass a copy of the original vector rather the function operates on the original vector. Hence the changes made to the vector in the function are also permanent or are reflected in the original vector.
Also, passing by refrence does not take up space and time since it does not create a new copy, so it's faster.
To pass a vector by reference use the & operator with the function argument of vector.
Syntax:-
void func( vector <int> &duplicate);
Let us take a look at the code below to understand this-
CPP Code
#include <bits/stdc++.h>
using namespace std;
// creating a function to pass the vector by reference to the function
void func( vector <int> &duplicate) { // the original vector is passed by reference
// let us reduce every even number in the vector by 1
int l = duplicate.size(); // storing the size of vector duplicate in l
// reducing every even number in duplicate by 1
for(int i = 0; i < l; i++){
if(duplicate[i]%2 == 0)
duplicate[i]--;
}
// displaying the modified vector
cout<<"Modified vector in the function-->"<<endl;
for(int i = 0;i < l;i++)
cout<<duplicate[i]<<" ";
cout<<endl;
}
int main(){
vector <int> original = {1,2,3,4,5,6,7,8,10}; // creating the original vector
func( original ); // calling the function func
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++)
cout<<original[i]<<" ";
return 0;
}
Output:-
Modified vector in the function--> 1 1 3 3 5 5 7 7 9 Original vector is--> 1 1 3 3 5 5 7 7 9
In the code above we have passed the original vector to the function func by reference using the & operator, and after modifying the vector in the funciton we can see that the changes made are also reflected in the original vector.
Need of Passing by reference
- To modify the original vector.
- To make the code faster in certain scenarios. For example when the function does not modify the vector it is better to pass by reference so as to make the code faster.
Passing a 2D Vector to the function
A 2D vector is a vector of vectors. It can contain any number of vectors in it. Like vectors, 2D vector is a dynamic version of the 2D arrays. Here the size of each vector in the 2d vector can be dynamic and different, whereas in 2D array this is not possible.
A 2D vector can be passed to the function in the same way as 1D vector, using both:
- Pass By Value
- Pass By Reference
CPP Code
#include <bits/stdc++.h>
using namespace std;
// creating a function to pass 2D vector by value to the function
void byValue(vector <vector <int> > duplicate) { // passing a 2D vector by value
// changing every value of 2D vector to -1
int row = duplicate.size(); // number of rows in the 2D vector or number of 1D vector
for(int i = 0; i<row; i++){
for(int j = 0; j<duplicate[i].size();j++)
duplicate[i][j] = -1;
}
// displaying the modified 2D vector
cout<<"Modified 2D Vector in the function by value-->"<<endl;
for(int i = 0;i<row; i++){
for(int j=0;j<duplicate[i].size(); j++)
cout<<duplicate[i][j]<<" ";
cout<<endl;
}
}
// creating a function to pass 2D vector by reference
void byRef(vector <vector <int> > &duplicate) { // passing a 2D vector by reference
// changing every value of 2D vector to -1
int row = duplicate.size(); // number of rows in the 2D vector or number of 1D vector
for(int i = 0; i<row; i++){
for(int j = 0; j<duplicate[i].size();j++)
duplicate[i][j] = -1;
}
// displaying the modified 2D vector
cout<<"Modified 2D Vector in the function by reference-->"<<endl;
for(int i = 0;i<row; i++){
for(int j=0;j<duplicate[i].size(); j++)
cout<<duplicate[i][j]<<" ";
cout<<endl;
}
}
int main(){
vector <vector <int> > original = {{1,2,3},{4,5,6},{7,8,10}}; // creating the original vector
byValue( original ); // calling the function byValue
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++){
for(int j=0;j<original[i].size();j++)
cout<<original[i][j]<<" ";
cout<<endl;
}
cout<<endl;
byRef( original ); // calling the function byRef
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++){
for(int j=0;j<original[i].size();j++)
cout<<original[i][j]<<" ";
cout<<endl;
}
return 0;
}
Output
Modified 2D Vector in the function by value--> -1 -1 -1 -1 -1 -1 -1 -1 -1 Original vector is--> 1 2 3 4 5 6 7 8 10 Modified 2D Vector in the function by reference--> -1 -1 -1 -1 -1 -1 -1 -1 -1 Original vector is--> -1 -1 -1 -1 -1 -1 -1 -1 -1
The above code shows how to pass a 2D vector by both value and reference. Here the processing of the vector is the same as in 1D vector. In the above code we first pass the vector by value and then by reference. Passing by value doesn't modify the original vector and works on the copy of the original vector. Whereas when passing by reference changes are reflected in the original vector as well.
\Passing a 3D vector to the Function
3D vector is a vector of 2D vectors. Here each vector/row of 3D vector is a 2D vector. This 3D vector is also dynamic and can contain any number of elements in it.
A 3D vector can be passed to the function in the same way as 1D vector, using both:
- Pass By Value
- Pass By Reference
CPP Code
#include <bits/stdc++.h>
using namespace std;
// creating a function to pass 3D vector by value to the function
void byValue(vector <vector <vector <int> > > duplicate) { // passing a 3D vector by value
// changing every value of 3D vector to -1
int row = duplicate.size(); // number of rows in the 3D vector or number of 2D vector
for(int i = 0; i<row; i++){
for(int j = 0; j<duplicate[i].size();j++){
for(int k=0;k<duplicate[i][j].size();k++)
duplicate[i][j][k]=-1;
}
}
// displaying the modified 3D vector
cout<<"Modified 3D Vector in the function by value-->"<<endl;
for(int i = 0;i<row; i++){
cout<<i+1<<"-> 2D row of the 3D vector-->"<<endl;
for(int j=0;j<duplicate[i].size(); j++){
for(int k=0;k<duplicate[i][j].size();k++)
cout<<duplicate[i][j][k]<<" ";
cout<<endl;
}
cout<<endl;
}
}
// creating a function to pass 3D vector by reference
void byRef(vector <vector <vector <int> > > &duplicate) { // passing a 3D vector by reference
// changing every value of 3D vector to -1
int row = duplicate.size(); // number of rows in the 3D vector or number of 2D vector
for(int i = 0; i<row; i++){
for(int j = 0; j<duplicate[i].size();j++){
for(int k=0;k<duplicate[i][j].size();k++)
duplicate[i][j][k]=-1;
}
}
// displaying the modified 3D vector
cout<<"Modified 3D Vector in the function by reference-->"<<endl;
for(int i = 0;i<row; i++){
cout<<i+1<<"-> 2D row of the 3D vector-->"<<endl;
for(int j=0;j<duplicate[i].size(); j++){
for(int k=0;k<duplicate[i][j].size();k++)
cout<<duplicate[i][j][k]<<" ";
cout<<endl;
}
cout<<endl;
}
}
int main(){
vector <vector < vector <int> > > original = {{{1,2,3},{4,5,6},{7,8,10}},{{11,12,13},{14,15,16},{17,28,29}}}; // creating the original vector
byValue( original ); // calling the function byValue
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++){
cout<<i+1<<"-> 2D row of the 3D vector-->"<<endl;
for(int j=0;j<original[i].size();j++){
for(int k=0;k<original[i][j].size();k++)
cout<<original[i][j][k]<<" ";
cout<<endl;
}
cout<<endl;
}
cout<<endl;
byRef( original ); // calling the function byRef
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++){
cout<<i+1<<"-> 2D row of the 3D vector-->"<<endl;
for(int j=0;j<original[i].size();j++){
for(int k=0;k<original[i][j].size();k++)
cout<<original[i][j][k]<<" ";
cout<<endl;
}
cout<<endl;
}
cout<<endl;
return 0;
}
Output:-
Modified 3D Vector in the function by value--> 1-> 2D row of the 3D vector--> -1 -1 -1 -1 -1 -1 -1 -1 -1 2-> 2D row of the 3D vector--> -1 -1 -1 -1 -1 -1 -1 -1 -1 Original vector is--> 1-> 2D row of the 3D vector--> 1 2 3 4 5 6 7 8 10 2-> 2D row of the 3D vector--> 11 12 13 14 15 16 17 28 29 Modified 3D Vector in the function by reference--> 1-> 2D row of the 3D vector--> -1 -1 -1 -1 -1 -1 -1 -1 -1 2-> 2D row of the 3D vector--> -1 -1 -1 -1 -1 -1 -1 -1 -1 Original vector is--> 1-> 2D row of the 3D vector--> -1 -1 -1 -1 -1 -1 -1 -1 -1 2-> 2D row of the 3D vector--> -1 -1 -1 -1 -1 -1 -1 -1 -1
Looking at the above code we can see that when passed to the function the 3D vector behaves in the same way as that of the 1D and 2D vectors. When passed to the function byValue, the changes are made to the copy of the vector and when passed to the function to the function byRef, changes made to the vector are reflected in the original vector.
Passing a global vector to the function
A global vector is a vector which is declared outside the main function and is available to all the functions of the program. A gloabal vector can be accessed by any function and hence the changes made to the vector are made to the original vector and we do not need to pass the vector to the function. This means that when used in this way the original vector is used instead of its copy.
To understand this, let us take a look at the following example:-
CPP Code
#include <bits/stdc++.h>
using namespace std;
vector <int> global; // declaring a global vector
void func(){ // we do not need to pass the global vector to a function if we want to
// make changes to the original global vector
// let us add a few elements to the gloabl vector
for(int i=1; i<10; i++)
global.push_back(i);
// displaying the modified vector in the function
cout<<"Modified vector inside the function-->"<<endl;
for(int i=0;i<global.size();i++)
cout<<global[i]<<" ";
}
int main(){
// invoking the funtion func
func();
// displaying the original global vector
cout<<endl<<"The original global vector is-->"<<endl;
for(int i=0;i<global.size();i++)
cout<<global[i]<<" ";
return 0;
}
Output:-
Modified vector inside the function--> 1 2 3 4 5 6 7 8 9 The original global vector is--> 1 2 3 4 5 6 7 8 9
In the above program we can see that when the elements are added to the global vector they are added to the original global vector, hence they are shown when we display the original vector in the main function.
Passing a global vector by value
We can also pass a global vector to a function by value, if we do not intend to cause any modification in the gloabl vector. This can be shown by the code below:-
CPP Code
#include <bits/stdc++.h>
using namespace std;
vector <int> global; // declaring a global vector
void func( vector <int> duplicate){ // passing the global vector by value
// we will make changes to the duplicate vector
// let us change every element to twice its value
for(int i=0;i<duplicate.size();i++)
duplicate[i] *= 2;
// displaying the modified vector in the function
cout<<"Modified vector inside the function-->"<<endl;
for(int i=0;i<duplicate.size();i++)
cout<<duplicate[i]<<" ";
}
int main(){
// initializing the global vector
for(int i=1; i<10;i++)
global.push_back(i);
// invoking the funtion func
func(global);
// displaying the original global vector
cout<<endl<<"The original global vector is-->"<<endl;
for(int i=0;i<global.size();i++)
cout<<global[i]<<" ";
return 0;
}
Output:-
Modified vector inside the function--> 2 4 6 8 10 12 14 16 18 The original global vector is--> 1 2 3 4 5 6 7 8 9
We can see in the output of the above code, that the changes made to the vector inside the function are not reflected in the global vector.
How to return a Vector from a function?
Returning a vector form a function is a very simple task. For this the return type of the function is the same as the vector, for example:-
If we wish to return a vector of int then, we will have to declare the function in this way:-
vector <int> func();
Let us take a look at how to implement it in the code:-
CPP Code
#include <bits/stdc++.h>
using namespace std;
vector <int> func(){ // declaring a function of type vector <int>
vector <int> send; // declaring the vector to be returned to the main function
// adding elements to the vector send
for(int i=1;i<=10;i++)
send.push_back(i);
// displaying the newly created vector
cout<<"Vector created in the function-->"<<endl;
for(int i=0;i<send.size();i++)
cout<<send[i]<<" ";
return send; // returning the vector send to the main funciton
}
int main(){
vector <int> recieve; // declaring a vector in which we will recieve the vector returned by the function
recieve = func(); // storing the returned vector in recieve
// displaying the recieved vector
cout<<endl<<"Vector recieved in the main function-->"<<endl;
for(int i=0;i<recieve.size();i++)
cout<<recieve[i]<<" ";
return 0;
}
Output:-
Vector created in the function--> 1 2 3 4 5 6 7 8 9 10 Vector recieved in the main function--> 1 2 3 4 5 6 7 8 9 10
In the above code we created a vector named as send in the function and then returned it to the main function, where it is then stored in the recieve vector.
With this article at OpenGenus, you must have the complete idea of Passing a vector to a function in C++ and returning a vector from a function in C++.