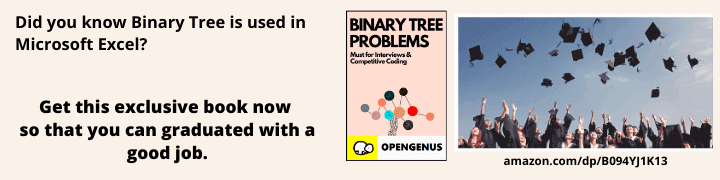
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the Different Preprocessor Directives in C / C++ along with C++ code examples. Preprocessor Directives are processed at compile time and is an important programming technique in large codebase.
Table of contents:
- Introduction to Preprocessor Directives
- File Inclusion Directive
- Macro Substitution Directive
- Conditional Compilation Directive
Let us get started with Preprocessor Directives.
Introduction to Preprocessor Directives
A Preprocessor in C is a program that analyses or processes the source code file that is to be used in the program before it is given to the compiler.
The following diagram represents the operation of a preprocessor preprocessor-1
Preprocessor Directive are codes in C with special commands, these codes are not C language rather are special and specific instructions to the preprocessor.
The syntax of a preprocessor:
It begins with a '#' and does not end with a semicolon(;)
There are different types of Preprocessor Directives in C:
- File Inclusion Directive
- Macro Substitution Directive
- Simple Macro
- Argumented Macro
- Nested Macros
- Conditional Compilation Directive
- if directives
- elif directives
- ifdef directives
- ifndef directives
- else directives
- endif directives
1) File Inclusion Directive
"#include" is the file inclusion Preprocessor Directive.
Syntax:
#include<filename>
It commands the compiler to include contents of the specified files under the enclosed header file written in the directive
2) Macro Substitution Directive
Macro Substitution is a process where an identifier is replaced by a predefined string or a value. "#define" is the Macro Substitution directive in C.
There are three types of macros in C:
a) Simple Macro
This type of macro substitutes the value of a defined symbol in the C program, whenever the symbol appears the macro would replace the symbol with the value assigned to it. This is mainly used in programs to define constants that appear repeatedly in the program but with no change in value.
Example:
#define PI 3.14
b) Argumented Macro
This macro is called argumented macro since it looks like a function. It represents small code snippet, each time the macro name is encountered its arguments are replaced with the actual argument of the program.
Example:
#HALFOF(x)x/2
c) Nested Macros :
A macro can be used within another macro this is called nesting of macros.
Example:
#define CUBE(x)SQR(x)*(x)
3) Conditional Compilation Directive
This type of preprocessors in C conditionally suppress the compilation portion of the source code.
there are six types of Conditional Compilation Directive
i) if directives
The #if directive allows conditional compilation, the preprocessor evaluates an expression provided with #if directive to determine if the subsequent code should be included in the compilation process.
Syntax:
#if conditional_expression
/* Example using #if directive*/
#include <stdio.h>
#define WINDOWS 1
int main()
{
printf("The Website is a great ");
#if WINDOWS
printf("Windows ");
#endif
printf("resource.\n");
return 0;
}
ii) elif directives
The #elif directive provides an alternate section action when used with if,ifdef or , ifndef directives, it includes the C source code that follows the #elif statement when the condition of the preceding #if, #ifdef or #ifndef directive evaluates to false ad the #elif conditions evaluates to be true
Syntax:
#elif conditional_expression
/* Example using #elif directive*/
#include <stdio.h>
#define YEARS_OLD 12
int main()
{
#if YEARS_OLD <= 10
printf("The Website is a great resource.\n");
#elif YEARS_OLD > 10
printf("Website is over %d years old.\n", YEARS_OLD);
#endif
return 0;
}
iii) ifdef directives
The ifdef directive determines of the provided macro exists before including the subsequent C code in the compilation process
Syntax:
#ifdef macro_definition
/* Example using #ifdef directive*/
#include <stdio.h>
#define YEARS_OLD 10
int main()
{
#ifdef YEARS_OLD
printf("The Website is over %d years old.\n", YEARS_OLD);
#endif
printf("The Website is a great resource.\n");
return 0;
}
iv) ifndef directives
The ifndef directive determines if the provided macro does not exist before including the subsequent C program code in the compilation process
Syntax:
#ifndef macro_definition
/* Example using #ifndef directive*/
#include <stdio.h>
#define YEARS_OLD 12
#ifndef YEARS_OLD
#define YEARS_OLD 10
#endif
int main()
{
printf("The Website is over %d years old.\n", YEARS_OLD);
return 0;
}
v) else directives
The #else directive provides an alternate action when used with the if, ifndef, or ifdef directives, the preprocessor will include the C program code that follows the #else statement when the condition for the #if, #ifndef or the #ifdef directives evaluate to be false.
Syntax:
#else
/* Example using #else directive */
#include <stdio.h>
#define YEARS_OLD 12
int main()
{
#if YEARS_OLD < 10
printf("The Website is a great resource.\n");
#else
printf("The Website is over %d years old.\n", YEARS_OLD);
#endif
return 0;
}
vi) endif directives
The endif directives is used to close off if, ifdef, ifndef, elif, else directives, if and when the the #endif directive is encountered, the preprocessing of the opening directive is completed.
Syntax:
#endif
/* Example using #endif directive */
#include <stdio.h>
#define WINDOWS 1
int main()
{
printf("The Website is a great ");
#if WINDOWS
printf("Windows ");
#endif
printf("resource.\n");
return 0;
}
With this article at OpenGenus, you must have the complete knowledge on Preprocessor Directives in C / C++.