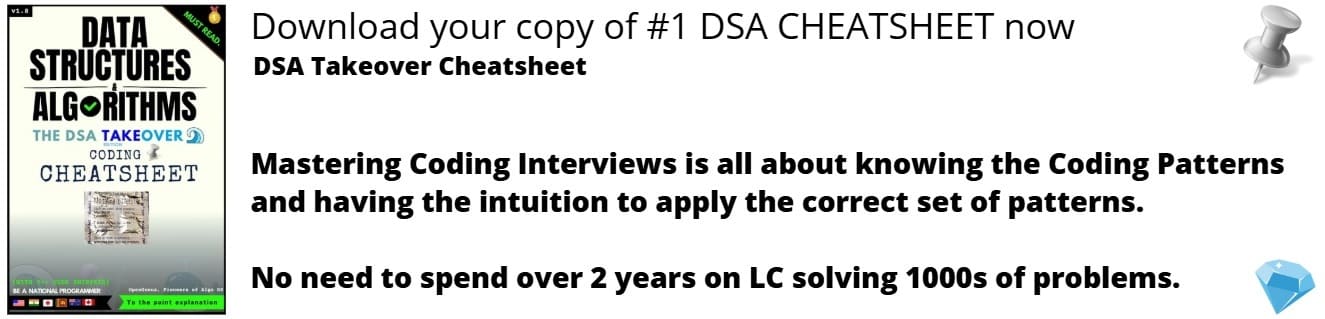
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Introduction
- Code
- Complexity
- Applications
- Questions
- Conclusion
Introduction
-
Python is a versatile programming language that can be used for a wide range of tasks. One of its many applications is creating patterns using code. In this article, we will explore 20 different Python programs that can be used to print various patterns.
-
List of patterns covered :-
- Square Pattern
- Right Triangle Pattern
- Left Triangle Pattern
- Inverted Right Triangle Pattern
- Inverted Left Triangle Pattern
- Pyramid Pattern
- Inverted Pyramid Pattern
- Diamond Pattern
- Hourglass Pattern
- Checkerboard Pattern
- Hollow Square Pattern
- Hollow Right Triangle
- Hollow Pyramid Pattern
- Hollow Diamond Pattern
- Hollow Hourglass Pattern
- Cross Pattern
- Plus Pattern
- Left Arrow Pattern
- Right Arrow Pattern
- Heart Pattern
Code
1. Square Pattern
- To print a square pattern of n x n stars, we can use the following code:
n = 5
for i in range(n):
for j in range(n):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the square. The inner loop prints a star followed by a space n times, while the outer loop moves to the next line after each row is printed.
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
2. Right Triangle Pattern
- To print a right triangle pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(i+1):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the triangle. The inner loop prints a star followed by a space i+1 times, where i is the current row number. The outer loop moves to the next line after each row is printed.
*
* *
* * *
* * * *
* * * * *
3. Left Triangle Pattern
- To print a left triangle pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i+1):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the triangle. The first inner loop prints n-i-1 spaces to align the stars to the left, while the second inner loop prints a star followed by a space i+1 times. The outer loop moves to the next line after each row is printed.
*
* *
* * *
* * * *
* * * * *
4. Inverted Right Triangle Pattern
- To print an inverted right triangle pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(n-i):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the triangle. The inner loop prints a star followed by a space n-i times, where i is the current row number. The outer loop moves to the next line after each row is printed.
* * * * *
* * * *
* * *
* *
*
5. Inverted Left Triangle Pattern
- To print an inverted left triangle pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(i):
print(' ', end=' ')
for k in range(n-i):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the triangle. The first inner loop prints i spaces to align the stars to the left, while the second inner loop prints a star followed by a space n-i times. The outer loop moves to the next line after each row is printed.
* * * * *
* * * *
* * *
* *
*
6. Pyramid Pattern
- To print a pyramid pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the pyramid. The first inner loop prints n-i-1 spaces to center the stars, while the second inner loop prints a star followed by a space i*2+1 times. The outer loop moves to the next line after each row is printed.
*
* * *
* * * * *
* * * * * * *
* * * * * * * * *
7. Inverted Pyramid Pattern
- To print an inverted pyramid pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(i):
print(' ', end=' ')
for k in range((n-i)*2-1):
print('*', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the pyramid. The first inner loop prints i spaces to center the stars, while the second inner loop prints a star followed by a space (n-i)*2-1 times. The outer loop moves to the next line after each row is printed.
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
8. Diamond Pattern
- To print a diamond pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
print('*', end=' ')
print()
for i in range(n-2, -1, -1):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
print('*', end=' ')
print()
This code uses two sets of nested for loops to iterate over the rows and columns of the diamond. The first set of loops prints the top half of the diamond, while the second set prints the bottom half. The inner loops are used to print spaces and stars in the appropriate positions.
*
* * *
* * * * *
* * * * * * *
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
9. Hourglass Pattern
- To print an hourglass pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(i):
print(' ', end=' ')
for k in range((n-i)*2-1):
print('*', end=' ')
print()
for i in range(1, n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
print('*', end=' ')
print()
This code uses two sets of nested for loops to iterate over the rows and columns of the hourglass. The first set of loops prints the top half of the hourglass, while the second set prints the bottom half. The inner loops are used to print spaces and stars in the appropriate positions.
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
* * *
* * * * *
* * * * * * *
* * * * * * * * *
10. Checkerboard Pattern
- To print a checkerboard pattern of n x n squares, we can use the following code:
n = 5
for i in range(n):
for j in range(n):
if (i+j) % 2 == 0:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the checkerboard. The inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
* * *
* *
* * *
* *
* * *
11. Hollow Square Pattern
- To print a hollow square pattern of n x n stars, we can use the following code:
n = 5
for i in range(n):
for j in range(n):
if i == 0 or i == n-1 or j == 0 or j == n-1:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the square. The inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
* * * * *
* *
* *
* *
* * * * *
12. Hollow Right Triangle
- To print a hollow right triangle pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(i+1):
if i == n-1 or j == 0 or j == i:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the triangle. The inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
*
* *
* *
* *
* * * * *
13. Hollow Pyramid Pattern
- To print a hollow pyramid pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
if k == 0 or k == i*2 or i == n-1:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the pyramid. The first inner loop prints n-i-1 spaces to center the stars, while the second inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
*
* *
* *
* *
* * * * * * * * *
14. Hollow Diamond Pattern
- To print a hollow diamond pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
if k == 0 or k == i*2:
print('*', end=' ')
else:
print(' ', end=' ')
print()
for i in range(n-2, -1, -1):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
if k == 0 or k == i*2:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two sets of nested for loops to iterate over the rows and columns of the diamond. The first set of loops prints the top half of the diamond, while the second set prints the bottom half. The inner loops are used to print spaces and stars in the appropriate positions.
*
* *
* *
* *
* *
* *
* *
* *
*
15. Hollow Hourglass Pattern
- To print a hollow hourglass pattern of n rows, we can use the following code:
n = 5
for i in range(n):
for j in range(i):
print(' ', end=' ')
for k in range((n-i)*2-1):
if k == 0 or k == (n-i)*2-2 or i == 0:
print('*', end=' ')
else:
print(' ', end=' ')
print()
for i in range(1, n):
for j in range(n-i-1):
print(' ', end=' ')
for k in range(i*2+1):
if k == 0 or k == i*2:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two sets of nested for loops to iterate over the rows and columns of the hourglass. The first set of loops prints the top half of the hourglass, while the second set prints the bottom half. The inner loops are used to print spaces and stars in the appropriate positions.
* * * * * * * * *
* *
* *
* *
*
* *
* *
* *
* *
16. Cross Pattern
- To print a cross pattern of n x n stars, we can use the following code:
n = 5
for i in range(n):
for j in range(n):
if i == j or j == n-i-1:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the cross. The inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
* *
* *
*
* *
* *
17. Plus Pattern
- To print a plus pattern of n x n stars, we can use the following code:
n = 5
for i in range(n):
for j in range(n):
if i == n//2 or j == n//2:
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the plus. The inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
*
*
* * * * *
*
*
18. Left Arrow Pattern
- To print a leftward arrow pattern of n rows using asterisks, you can use the following code:
n = 7
for i in range(n):
if i <= n//2:
for j in range(n//2-i):
print(' ', end=' ')
for k in range(i+1):
print('*', end=' ')
else:
for j in range(i-n//2):
print(' ', end=' ')
for k in range(n-i):
print('*', end=' ')
print()
This code uses a for loop to iterate over the rows of the arrow pattern. The inner loops use conditional statements to determine whether to print a space or a star based on the current row number. The outer loop moves to the next line after each row is printed.
*
* *
* * *
* * * *
* * *
* *
*
19. Right Arrow Pattern
- To print a rightward arrow pattern of n rows using asterisks, you can use the following code:
n = 7
for i in range(n):
if i <= n//2:
for j in range(n//2):
print(' ', end=' ')
for k in range(i+1):
print('*', end=' ')
else:
for j in range(n//2):
print(' ', end=' ')
for k in range(n-i):
print('*', end=' ')
print()
This code uses a for loop to iterate over the rows of the arrow pattern. The inner loops use conditional statements to determine whether to print a space or a star based on the current row number. The outer loop moves to the next line after each row is printed.
*
* *
* * *
* * * *
* * *
* *
*
20. Heart Pattern
- To print a heart pattern of n rows, we can use the following code:
for row in range(6):
for col in range(7):
if (row == 0 and col % 3 != 0) or (row == 1 and col % 3 == 0) or (row - col == 2) or (row + col == 8):
print('*', end=' ')
else:
print(' ', end=' ')
print()
This code uses two nested for loops to iterate over the rows and columns of the heart pattern. The inner loop uses a conditional statement to determine whether to print a star or a space based on the current row and column numbers. The outer loop moves to the next line after each row is printed.
* * * *
* * *
* *
* *
* *
*
Complexity
-
The time complexity of these programs depends on the size of the pattern being printed.
-
For patterns with n rows and columns, the time complexity is generally *O(n^2) *since we need to iterate over all rows and columns.
Applications
-
These programs can be used for educational purposes to teach programming concepts such as loops and conditional statements.
-
They can also be used to create ASCII art or as part of larger programs that require pattern generation.
Questions
- Can these programs be modified to print patterns using different characters?
- How can we optimize these programs to run faster?
- Can these programs be extended to print more complex patterns?
Conclusion
In this article at OpenGenus, we have explored 20 different Python programs that can be used to print various patterns. These programs demonstrate the versatility and power of Python as a programming language and provide a starting point for further exploration and learning.