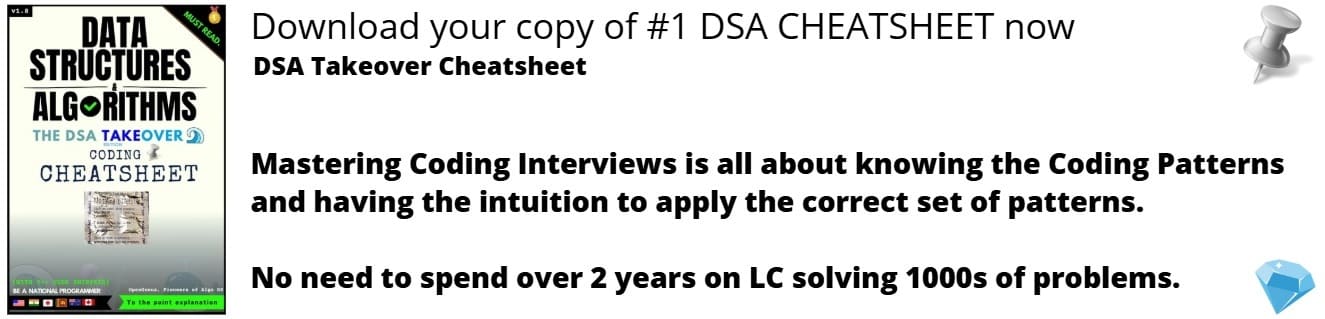
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In software development, creating maintainable, flexible, and scalable code is of utmost importance. As applications grow in complexity, managing code becomes a challenging task. To address these challenges, the SOLID principles were introduced as a set of guidelines for designing object-oriented systems that are easy to understand, maintain, and extend. In this article at OpenGenus, we will dive deep into SOLID principles and illustrate them with practical code examples.
SOLID is an acronym that represents five key principles:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
1. Single Responsibility Principle (SRP):
The Single Responsibility Principle states that a class should have only one reason to change. In other words, a class should have only one responsibility or concern. By adhering to this principle, we keep classes focused and avoid coupling unrelated functionality within them. This leads to better maintainability and allows changes to one aspect of a system without affecting other parts.
Code Example:
Consider a simple class Customer
that holds basic information about a customer:
class Customer:
def __init__(self, name, email):
self.name = name
self.email = email
def get_customer_info(self):
return f"Name: {self.name}, Email: {self.email}"
def send_email(self, subject, message):
# Code to send an email to the customer
pass
In the above example, the Customer
class has two responsibilities: managing customer information and sending emails. To adhere to the SRP, we should separate these concerns into distinct classes:
class Customer:
def __init__(self, name, email):
self.name = name
self.email = email
def get_customer_info(self):
return f"Name: {self.name}, Email: {self.email}"
class EmailService:
def send_email(self, recipient, subject, message):
# Code to send an email to the recipient
pass
Now, the Customer
class is responsible only for customer information, while the EmailService
class handles email-related functionality. This makes the code more maintainable and follows the Single Responsibility Principle.
2. Open/Closed Principle (OCP):
The Open/Closed Principle emphasizes that classes should be open for extension but closed for modification. This means that we should design classes in a way that new functionality can be added without altering their existing code. This leads to less risk of introducing bugs in the existing codebase and promotes code reuse.
Code Example:
Let's consider a Shape
class that calculates the area of different shapes:
class Shape:
def __init__(self, type):
self.type = type
def calculate_area(self):
if self.type == "circle":
return self.calculate_circle_area()
elif self.type == "rectangle":
return self.calculate_rectangle_area()
def calculate_circle_area(self):
# Code to calculate the area of a circle
pass
def calculate_rectangle_area(self):
# Code to calculate the area of a rectangle
pass
In the above example, the Shape
class violates the OCP since every time we want to add a new shape, we need to modify the existing class to accommodate the new calculation logic. To adhere to the OCP, we can use inheritance and create separate classes for each shape:
class Shape:
def __init__(self):
pass
def calculate_area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def calculate_area(self):
return 3.14 * self.radius ** 2
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def calculate_area(self):
return self.width * self.height
Now, we can add new shapes by creating new classes that inherit from the Shape
class, without modifying the existing code. This adheres to the Open/Closed Principle.
3. Liskov Substitution Principle (LSP):
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In simpler terms, a derived class should be able to replace its base class without altering the behavior of the program.
Code Example:
Consider a Bird
class hierarchy:
class Bird:
def fly(self):
pass
class Sparrow(Bird):
def fly(self):
return "Sparrow flying"
class Penguin(Bird):
def fly(self):
return "Penguin can't fly"
In this example, the Penguin
class violates the Liskov Substitution Principle because it alters the behavior of the base class. To adhere to LSP, we can split the Bird
class into two separate classes:
class FlyingBird:
def fly(self):
pass
class Bird(FlyingBird):
pass
class Sparrow(Bird):
def fly(self):
return "Sparrow flying"
class Penguin(FlyingBird):
pass
Now, the Penguin
class doesn't interfere with the fly()
method, and we've separated the flying behavior into the FlyingBird
class, which allows us to adhere to the Liskov Substitution Principle.
4. Interface Segregation Principle (ISP):
The Interface Segregation Principle suggests that clients should not be forced to depend on interfaces they do not use. In other words, it's better to have multiple specific interfaces rather than a single large interface. This ensures that implementing classes are only required to provide the methods they actually need.
Code Example:
Consider a large interface Printer
that has several methods:
class Printer:
def print(self):
pass
def scan(self):
pass
def fax(self):
pass
In this example, if a class only needs the print()
functionality, it is still forced to implement the scan()
and fax()
methods, which may lead to empty or unnecessary implementations. To adhere to ISP, we can split the interface into smaller, more specific interfaces:
class Printer:
def print(self):
pass
class Scanner:
def scan(self):
pass
class FaxMachine:
def fax(self):
pass
Now, classes can implement only the interfaces they need, promoting cleaner and more focused code that follows the Interface Segregation Principle.
5. Dependency Inversion Principle (DIP):
The Dependency Inversion Principle suggests that high-level modules should not depend on low-level modules; instead, both should depend on abstractions. Abstractions should not depend on details; details should depend on abstractions. This principle encourages the use of dependency injection and inversion of control to decouple components, making the system more flexible and easier to maintain.
Code Example:
Consider a NotificationService
class that sends notifications:
class NotificationService:
def __init__(self):
self.email_service = EmailService()
def send_notification(self, message):
self.email_service.send_email("recipient@example.com", "Notification", message)
In this example, the NotificationService
directly depends on the EmailService
implementation, violating the DIP. To adhere to the DIP, we can use dependency injection to inject the dependency through the constructor:
class NotificationService:
def __init__(self, email_service):
self.email_service = email_service
def send_notification(self, recipient, message):
self.email_service.send_email(recipient, "Notification", message)
Now, the NotificationService
depends on an abstraction, represented by the EmailService
interface, rather than the concrete implementation. This promotes better decoupling and adherence to the Dependency Inversion Principle.
Conclusion:
The SOLID principles serve as a fundamental guide for building maintainable, scalable, and efficient object-oriented software systems. By applying the Single Responsibility Principle, Open/Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle, developers can create code that is easier to understand, test, and extend. It is crucial to keep these principles in mind during the design and implementation phases of software development to ensure the longevity and success of our projects.
Remember that while adhering to SOLID principles, we should not over-engineer or over-complicate the code. The goal is to find the right balance between adhering to the principles and keeping the codebase maintainable and efficient.