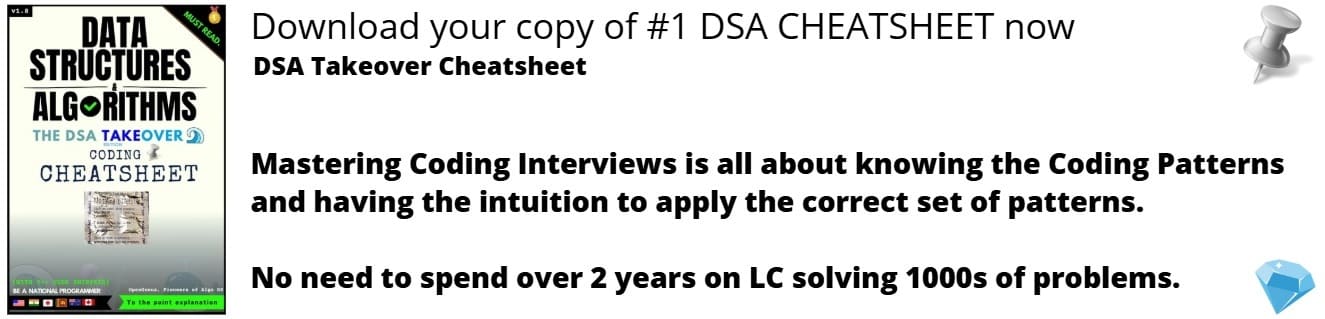
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored Static class variable in Python along with code examples. We have covered different cases like accessing class variable inside and outside class.
Table of Contents:
- what is class?
- what is objects?
- What is Static Variable?
- Creating Static variable in python
- Classification of static variable in python
- Accessing class variable inside the class
- Accessing class variable outside of the class
- Question
To know about Static class variable first we need to know about what is class and object
What is class?
A class is like a blueprint for creating an object.
All classes have a function called init(), which is always executed when the class is being initiated.
What is object?
Objects are the instances of a particular class.
Every other element in Python will be an object of some class
So now we have the idea about the class and objects lets look into Static Class Variable
Static variable
Static variable also known as Class variable are the variables whose single copy is available to all the insatnce of the class whereas in Instance variables are the variables who have individual copy available to every object of the class.
Whenever we declare a variable inside static class it stores a single copy inside heap memory and it is shared with all the object of that class so if modify the variable it will be modified to all the object of the class.
That means if we modify the copy of class variable in an instance, it will effect all the copies in other instance.
here is real life example of static variable ,
Let a Instrument shop be represented by a class Instrument.
All the Customer who buys Instrument from that shop will be provided free course offered for learning instrument .The class may have a static variable whose value is βyesβ for all objects. And class may also have non-static members like Instrumentname and Count.
Example,
class Instrument:
sp='Yes' #class variable (variable can be of any type)
#Outside of constructor inside of class
def __int__(self):
self.model='Guitar' #Instance variable
#variable instance should be inside the constructor
def show_model(self):
print(self.model) #accessing Instance variable
piano=Instrument() #Creation of object
Creating a Static Variable in Python
we can access static variables using the format - "className.staticVariable".
1.Declaring the Variable inside the Class
Unlike other Programming languages python does not have static keyword, instead of that we simply declare a variable inside the class, but it should not be inside any method, and that variable will be known as a static or class variable.
Here is code demonstration for that
# Python program to show that the variables with a value
# assigned in class declaration, are class variables
# Class for different type of Instrument
class Instrument:
courseoffered = 'yes' # Class Variable
def __init__(self,price,tax):
self.Instrumentname = Instrumentname # Instance Variable
self.count = count # Instance Variable
# Objects of CSStudent class
a = Instrument('Guitar', 1)
b = Instrument('piaono', 2)
print(a.courseoffered) # prints "yes"
print(b.courseoffered) # prints "yes"
print(a.Instrumentname) # prints "Guitar"
print(b.Instrumentname) # prints "Piaono"
print(a.count) # prints "1"
print(b.count) # prints "2"
# Class variables can be accessed using class
# name also
print(Instrument.courseoffered) # prints "yes"
# Now if we change the courseoffered for just a it won't be changed for b
a.courseoffered = 'No'
print(a.courseoffered) # prints 'no'
print(b.courseoffered) # prints 'yes'
# To change the courseoffered for all instances of the class we can change it
# directly from the class
instrument.courseoffered = 'yes'
print(a.courseoffered) # prints 'yes'
print(b.courseoffered) # prints 'no'
2. Using hasattr() method
hassttr() method is an inbuilt function in python which is used to check whether the object has the static class variable created or not . It returns True if the object has the static class variable created otherwise it will returns False.
Classfication of a Static Variable in Python:
1.class variable should be created inside the class but outside of method
2.static variable or class variable can be accessed inside the class as well as oustide but not directly with the instance
3.All objects of the class share the same copy of the static variable.
Accessing Static class Variables in Python
- Within Class Method:
- Outside the class
With Class Method:
To access class variable, we need class method with cls as first parameter then we can access variable using cls.variable name
For accessing class variable we use decorator @classmethod
Here is code demonstration for that
class Instrument:
fp='yes' # class variable
def __init__(self):
self.model='Guitar'
def show_model(self):
print(self.model)
@classMethod #class Method
def is_fp(cls):
cls.fp # accessing class variable inside the class
choir.Instrument()
2.Outside class
we can access the class or static variable using classname.variable_name
class Instrument:
fp='yes' #class variable
@classmethod #class method
def show(cls):
cls.fp # accessing class variable inside class method
guitar= Instrument()
Instrument.fp # accessing class variable outside class
Question
A variable that is defined inside a method and belongs only to the current instance of a class is known as?
A. Inheritance
B. Instance variable
C. Function overloading
D. Instantiation
View Answer
Ans : B
Explanation: Instance variable : A variable that is defined inside a method and belongs only to the current instance of a class.
With this article at OpenGenus, you must have the complete idea of static class variable in Python.