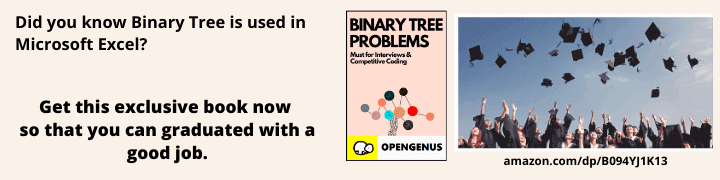
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to create an Array of structure in C and what is the need of it using a complete C code example.
Table of content
- Introduction
- Declaration and Its type
- Need for array of structure
- Example
Introduction
Structures are user defined data types . In general the structures can be defined as different data types under one name and arrays can be defined as similar data type under one name , so basically Array of structures is an Array of data type structure and that structure is defined by users need containing different data types like int,float char etc.
Array of structure helps programmers to achieve their goals of creating an array which can accomodate different data types it is done by creating a structure and adding all data types needed and store this structure as a single data type in an array , we can say that we have created a different data type using structure and stored it in an array.
Declaration and Its type -
Using struct keyword we can create a structure. under structure we can accomodate various different data types and bind them under structure to create a user defined data type .below is its implementation -
struct Employee {
char name[50];
int age;
float salary;
int phone[10];
char address [200];
};
It can be declared in two ways -
1.variable and structure declared combined -
Here variable is declared along with the structure
below is its implementation-
struct Employee {
char name[50];
int age;
float salary;
int phone[10];
char address [200];
}emp;
2. variable and structure declared separately -
Here structure is declared above and variable is declared in main() function.
below is its implementation -
struct Employee {
char name[50];
int age;
float salary;
int phone[10];
char address [200];
};
int main()
{
struct Employee emp;
}
Need for array of structure -
The main advanatage of array of structures is code reusability i.e instead of declaring multiple variables with different names we can use a single array and declare all similar data type structure variables under one variable .
E.g
int a,b,c,d,e,f; // without array variable declaration
int a[6]; // with array variable declaration
//both will store 6 int values
Array also increases readability and efficiency of our code.So array of structure can store multiple structures data under a single variable instead of declaring different structures variables which is confusing and time consuming.i.e.
istead of this -
struct Employee emp1,emp2,emp3,emp4.......emp100;
we will use below method for our program
struct Employee emp[100];
//and than we can access different employees by emp[0],emp[1],etc..
from above example we get a rough idea why we should use array of structures instead using different variables because instead of typing 100 variables name we use one variable array name to do the same task.
below is an structure of employee emp[100] .
Example -
Below is an example of array of structures -
#include<stdio.h>
struct employee
{
char name[50];
char city[30];
int id;
};
int main()
{
struct employee e[2];
for(int i = 0; i < 2; i++ )
{
printf("\nEnter details of employee %d\n", i+1);
printf("Enter name: ");
scanf("%s", e[i].name);
printf("\nEnter id: ");
scanf("%d", &e[i].id);
printf("\nEnter city: ");
scanf("%s", e[i].city);
printf("\n");
}
printf("\n");
printf("Name\tid\t\t\t\tcity\n");
for(int i = 0; i < 2; i++ )
{
printf("%s\t\t%d\t\t\t%s\t\t",
e[i].name, e[i].id, e[i].city);
printf("\n");
}
return 0;
}
Input and output:
Enter details of employee 1
Enter name: joe
Enter id: 78
Enter city: washington
Enter details of employee 2
Enter name: jack
Enter id: 76
Enter city: texas
Name id city
joe 78 washington
jack 76 texas
With this article at OpenGenus, we get a good idea of array of structures and their needs.