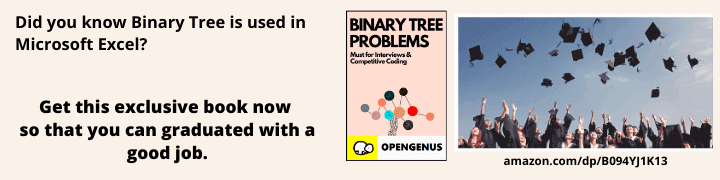
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 5 minutes
In this article, we will cover how hashing is natively supported in C++ through STL (std:hash) and how we can use it to generate hash of any object in C++.
When we use any associative container we unknowingly use an hash function. Basically the key-value pairs that we use in a map, multimap, unordered_map etc are mapped with each other. The basis of mapping comes from the hashcode generation and the hash function.
std::hash is a class in C++ Standard Template Library (STL). It is such a class that can be constructed in a more dafault way which in others words means that any user who intends to use the hash class can constuct the objects without any given initial values and arguments. So by default a hash class is a template class.
We will cover the following sub-topics:
- How to create an object in std::hash?
- Character hashing
- String hashing
- Integer hashing
- Vector hashing
- Hasing and collision
How to create an object in std::hash?
To create a objeect for the hash class we have to follow the following procedure:
hash<class template> object_name;
Member functions
The std::hash class only contains a single member function.
operator(): this returns the hashed value for each object argument that is provided to it.
Now lets directly switch over to different objects that can be used in the hash function to get their corresponding hash values.
Character hashing
#include<bits/stdc++>
using namespace std;
void character_Hashing()
{
char ch = 'b';
hash<char> hash_character;
cout << "\nthe hashed value is: " << hash_character(ch) << endl;
}
int main()
{
character_Hashing();
}
Output:
the hashed value is: 98
String hashing
To hash a string in C++, use the following snippet:
hash<char> hash_string;
hash_string(s); // s is a string
This C++ code example demonstrate how string hashing can be achieved in C++.
#include<bits/stdc++>
using namespace std;
void string_Hashing()
{
string s = "abc";
hash<char> hash_string;
cout << "\nthe hashed value is: " << hash_string(s) << endl;
}
int main()
{
string_Hashing();
}
Output:
the hashed value is: 3665446528845549387
Now for an integer the hash function returns the same value as the number that is given as input.The hash function returns an integer, and the input is an integer, so just returning the input value results in the most unique hash possible for the hash type.
Integer hashing
To hash an integer in C++, use the following snippet:
hash<int> hash_string;
hash_string(n); // n is an integer
This C++ code example demonstrate how integer hashing can be achieved in C++.
#include<bits/stdc++>
using namespace std;
void int_Hashing()
{
int n = 5;
hash<int> hash_int;
cout << "\nthe hashed value is: " << hash_int(n) << endl;
}
int main()
{
int_Hashing();
}
Output:
the hashed value is: 5
Vector hashing
To hash a vector in C++, use the following snippet:
// define the vector
vector<bool>
bol{ true, false,
true, false };
// create the hash function
hash<vector<bool> h_f> ;
// use the hash function
h_f(bol);
This C++ code example demonstrate how vector hashing can be achieved in C++.
#include<bits/stdc++.h>
using namespace std;
void vector_Hashing()
{
vector<bool>
bol{ true, false,
true, false };
hash<vector<bool> h_f> ;
cout << "\n hash value: "<< h_f(bol) << endl;
}
void main()
{
vector_Hashing();
}
output
hash value: 16935082123893034051
Apart from these standard data types, we can also use hash function for many other data types:
- boolean
- signed char
- unsigned char
- short
- unsigned sort
- signed sort
- float
- double
- long double
- long
and others.
So these were some types of data that can be hashed using the std::hash class.
the real use of std::hash comes in a hashtable where different key values are to be hashed to thier values.
All these hashing stuff that the hash functions do is just to find a unique identity all the arguments that need to be hashed and further referred using their hashed values.
Hasing and collision
Now the need of a good hash function is surely a concern because collision can be bad issue in case of a hash function that is not that diverse and robust. So sometimes it is also required we build some user defined hash function so that in collision cases we can handle it properly.
That was almost everything about std::hash. Hope you liked it.
Happy coding :)