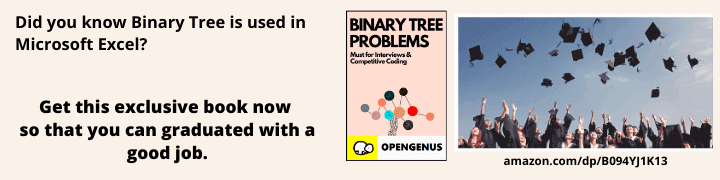
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, I have explained the String Pool in Java and how to implement it in Java programming. I have covered basic concepts of this topic.Also, I have explain the diagram.
- First of all we should know about String. So String is a special class in java and we can create String object using new operator as well as providing values in double quotes.
For example, "opengenus" and "Hello everyone" are both strings - String Pool in a Java is a storage area in Java heap(area of memory used for run-time operations).
- Pool is known as the common area.
- It is a common area to store constants.
- It is a concept where in distinct Strings have a single memory copy.
String greet1= "hello";
String greet2= "hello";
Above two variable will point to same memory location.
When greet1 is declared and assigned a value, JVM checks if the string exists in the commom pool. Since it is not there, adds it to pool.
When greet2 is declared and assigned same value, a value, JVM sees that the string exists in pool so does not create a separate location in memory.
String greet3= "Hlo";
Above variable will point to a separate memory location in pool since it is a separate string.
String greet4= new String("hello");
String class is a very commonly used class.Pooling helps save memory.
It is possible with String since they are immutable(means unchanging over time or unable to be changed).
String Pool in Java: Flow Diagram
In the above daigram,the String s1 and String s3 have same value of string constant , so they are pointing to the same String pool. Similarly , String s2 has different value than String s1 and String s3 , so it is located to diffent location in the String pool.
So, for s1==s2 , it will return false and for s1==s3 , it will return true.
Java Program for the above String Pool image:
public class StringPool {
public static void main(String[] args)
{
String s1= "Apple";
String s2= "Mango";
String s3= "Apple";
System.out.println("s1==s2 :"+ (s1==s2));
System.out.println("s1==s3 :"+ (s1==s3));
}
}
Output:-
s1==s2 : false
s1==s3 : true
When we use the new operator, we force String class to create a new String object in heap space. We can use intern()
method to put it into pool or refer to another String object from the String pool having the same value.
How many Strings are created in String Pool?
We should know about this question.For example, how many strings are getting created in the below statement:
String str= new String("hello");
In the above Statement , either 1 or 2 string will be created. If there is already a string literal "hello" in the pool, then only one string "str" will be created in the pool. If there is no string literal "hello" in the pool, then it will be first created in the pool and then in the heap space, so a total of 2 string objects will be created.
Why String is immutable in Java?
String Pool is possible only because String is immutable in Java.This way Java Runtime saves a lot of heap space because different String variable can refer to the same String variable in the pool.If String would not have been immutable, then String interning would not have been possible because if any variable would have changed the value,it would have been reflected in the other variables too.
Since String is immutable, it is safe for multithreading(process of executing multiple threads/sub-processes simultaneously).A single String instance can be shared across different threads.This avoids the use of synchronization(operation or activity of two or more things at the same time) for thread safety.Strings are implicitly thread safe.
Intern() function:
String interning is a method of storing only one copy of each distinct String value, which must be immutable. When we apply this function, it will assign same memory to the same string values.
For eg:-if a name "Jenn" appears 100 times, by interning we ensure that only one "Jennie" is actually allocated memory.By this, we can reduce the memory of our program.
When this method is executed then it checks whether the String equals to the String Object in the pool or not.If it is there, then string from the pool is returned.Otherwise, this String object is added to the pool.
Java Program to intern a string with String.intern() method
public class Str{
public static void main(String[] args)
{
String str1=new String("open genus"); //String object in heap
String str2= "open genus"; //String literal in pool
String str3= "open genus"; //String literal in pool
String str4= str1.intern(); //String object interned to literal
System.out.println(str1 == str2);
System.out.println(str2 == str3);
System.out.println(str2 == str4);
}
}
Output:
false
true
true
- String literal is a sequence of characters which is used by programmers to display string object or text to user.If there already exists same String value in String pool, then it will refer to that string and no new string object will be created.
- String object is used to create a new string with new() operator.It will take more time to execute than String literal because it will create a new string everytime it is executed.
What is equals method and == operator in Java:
- Both equals() method and the == operator is used to compare two objects in Java.
- == is an operator and equals() is method.
- We use == operator to check memory location or address of two objects are same or not.
- We use equals() method to compare the state or contents of two objects.This method is defined inside java.lang.Object class.
Advantages of String pool in Java
- The main advantage of the String pool is to reduce memory usage.
- String pool is basically an area in Heap memory that holds references to string objects.This is done to reuse the references to same string literals in the program.
- This also used to make String immutable.