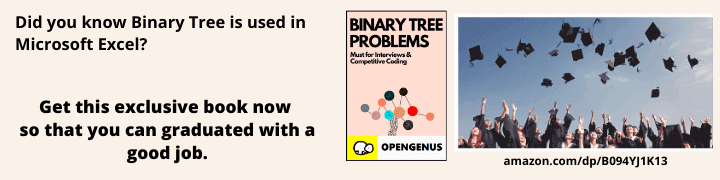
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about strtol in C++ and we will also compare it with other similar functions such as strtoul.
Table of contents:
- strtol in C++
- Example of using 'strtol'
- Example showing strtol() function with different bases
- Comparing strtol with other similar functions
strtol in C++
In C++, the 'strtol' is a STL(Standard Template Library) function that converts a string to a long integer. It is defined in the 'cstdlib' header file.
Following is the general syntax for using 'strtol' :
long strtol(const char* str, char** endptr, int base);
The function takes three arguments. These arguments are described below:
- 'str' is a pointer to the string to be converted (starting of a string).
- 'endptr' is a pointer to a 'char*' varible, where the function will store a pointer to the character following the last character used in the conversion. If 'endptr' is not 'NULL', 'strtol' will store a pointer to the first character not used in the conversion in this variable. It is not used if it is a 'NULL' pointer.
- 'base' is the base of the integer represented in the string. It must be between 2 and 36, or it can be '0'. If the base is '0', it indicates that the base is determined by the string itself (i.e. it can be octal, hexadecimal or decimal).
This function returns a long int value if a valid conversion occurs and 0 is returned if an error occurs.
Example of using 'strtol'
#include <iostream>
#include <cstdlib>
using namespace std;
int main() {
char str[] = "123456";
char* endptr;
long num = strtol(str, &endptr, 10);
if (endptr == str) {
// str is not a valid number
cout<<"Invalid input"<<endl;
} else {
cout<<"The number is: "<<num;
}
return 0;
}
The output of this program will be:
The number is: 123456
Note: strtol can handle strings that contain leading whitespace, negative numbers, and numbers in other bases (e.g., octal or hexadecimal).
Some examples of valid strings that can be converted to long integers using strtol:
Valid strings:
"1234"
" 1234" (with leading whitespace)
"-1234" (negative integer)
"+1234" (positive integer with explicit plus sign)
"9223372036854775807" (largest possible long integer on most systems)
Some examples of invalid strings that cannot be converted to long integers using strtol:
Invalid strings:
"abcd123" (starts with non-numeric characters)
" " (only contains whitespace)
"" (empty string)
"1.234" (contains a decimal point)
"1,234" (contains a comma)
You don't need to specify a base unless you want to parse a number in a different base as strtol is designed to parse integers in base 10 by default.
Example showing strtol() function with different bases:
#include <iostream>
#include <cstdlib>
using namespace std;
int main()
{
char str[] = "127az";
char *endptr;
cout << str << " to Long Int with base-8 = " << strtol(str, &endptr, 8) << endl;
cout << "End String = " << endptr << endl << endl;
cout << str << " to Long Int with base-16 = " << strtol(str, &endptr, 16) << endl;
cout << "End String = " << endptr << endl << endl;
cout << str << " to Long Int with base-36 = " << strtol(str, &endptr, 36) << endl;
cout << "End String = " << endptr << endl << endl;
return 0;
}
The output of this program will be:
127az to Long Int with base-8 = 87
End String = az
127az to Long Int with base-16 = 4730
End String = z
127az to Long Int with base-36 = 1782395
End String =
Note: If strtol encounters an error, it returns the value LONG_MIN or LONG_MAX, depending on the sign of the number, and sets the global variable errno (a preprocessor macro used for error indication) to one of the following values:
- ERANGE: The value of the number in the string is out of range for a long int.
- EINVAL: The base argument is not a valid base for a number in the string or the string is empty or does not contain a number.
After invoking strtol, we should check the value of errno to handle any errors that were returned. If one of the above mentioned values is set for errno, we should respond appropriately to handle the error. This can imply giving the user an error message, tracking the error, or doing something else to reduce the issue.
Comparing strtol with other similar functions:
strtol | strtoll | strtoul | strtoull |
It returns a long int. | It returns a long long int. | It returns an unsigned long int. | It returns an unsigned long long int. |
With this article at OpenGenus, you must have the complete idea of strtol in C++ Programming Language.