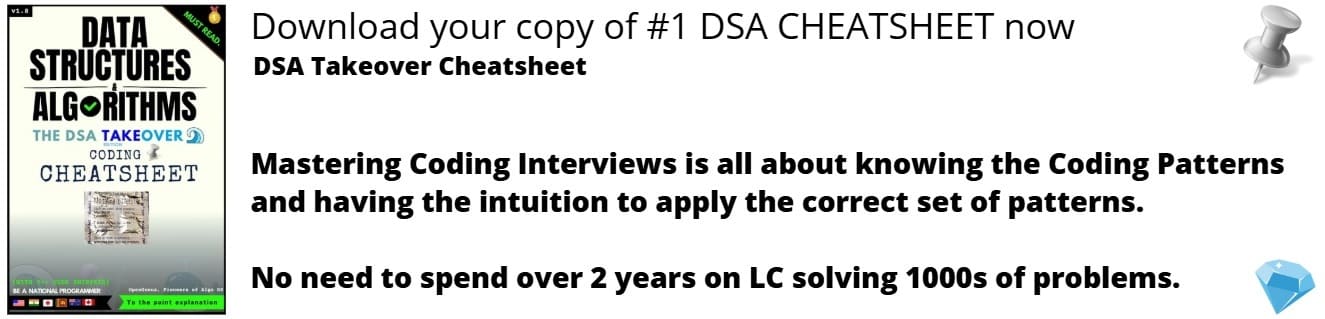
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained with code examples how to detect the key presses Ctrl + C and Ctrl + V using JavaScript on a webpage. The two keypresses are mainly used for Copy and Paste.
TABLE OF CONTENTS:
- Ctrl+C
- Ctrl+V
- How to detect copy paste commands Ctrl+V, Ctrl+C using JavaScript
Ctrl+C
This is sometimes called Control+C, ^c, or C-c. Ctrl+C is a keyboard shortcut that is used to copy highlighted text or other object like an image to the clipboard in a browser or illustrative user environment.
To initiate the command, press and hold Ctrl key and whild holding the key then press C on the keyboard.
Without highlighting the intended text, files, or other objects, nothing will be copied. Therefore, if you use the paste command, it displays what was stored previously in the clipboard, that is only if the copied text or object was highlighted before copying.
On Apple computers(Laptops and Desktops), the keyboard shortcut to copy is Command +C
Ctrl+V
This is sometimes called Control+V and C-v, ^v. Ctrl+V is a keyboard shortcut used to paste text or other object like an image from the clipboard.
To initiate the command, press and hold Ctrl key and whild holding the key then press V on the keyboard. Pressing Ctrl+V function will paste the contents of the clipboard into any editable field. You could paste text from another program into a text box in a form..
On Apple computers(Laptops and Desktops), the keyboard shortcut to copy is Command+V
How to detect copy paste commands Ctrl+V, Ctrl+C using JavaScript
To detect the copy paste commands using Javascript(JS), we use the ctrl property of the keydown event. It returns a “boolean” value to tell if “ctrl” is pressed or not when the key event got triggered.
Syntax:
event.ctrlKey
Return Value:
true: When “ctrl” was initiated.
false: When “ctrl” was not intiated.
Below is a breakdown of the codes. In the example below I will using a combination of HTML, CSS and Javascript codes.
- HTML code:“index.html”
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie-edge">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<textarea cols="30" row="5"
placeholder="Enter Text">
</textarea>
<textarea cols="30" row="5"
placeholder="Paste Text">
</textarea>
</div>
<script src="script.js"></script>
</body>
</html>
2.CSS code: “style.css”
.container{
display: flex;
flex-direction: column;
align-items: center;
}
textarea{
margin-top: 20px;
}
3.Javascript code: “script.js”
document.body.addEventListener("keydown", function (ev) {
// function to check the detection
ev = ev || window.event; // Event object 'ev'
var key = ev.which || ev.keyCode; // Detecting keyCode
// Detecting Ctrl
var ctrl = ev.ctrlKey ? ev.ctrlKey : ((key === 17)
? true : false);
// If key pressed is V and if ctrl is true.
if (key == 86 && ctrl) {
// print in console.
console.log("Ctrl+V is pressed.");
}
else if (key == 67 && ctrl) {
// If key pressed is C and if ctrl is true.
// print in console.
console.log("Ctrl+C is pressed.");
}
}, false);
- Output
When Ctrl+C command was implemented:
When Ctrl+V command was implemented:
You will see a detected result with an alert box when you press the keyboard shortcut Ctrl+C.
You will see a detected result with an alert box when you press the keyboard shortcut Ctrl+V.
With this article at OpenGenus, you must have the complete idea of how to detect Ctrl+C and Ctrl+V using JavaScript.