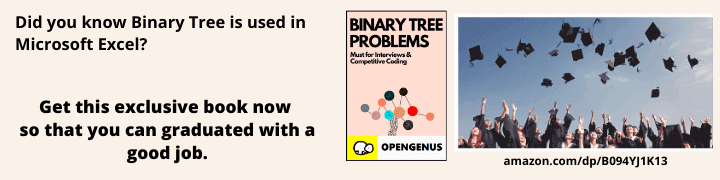
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the approach to create a Python script to create GitHub repository. In this, we have used GitHub REST API.
Table of contents:
- Introduction to GitHub
- What is Git?
- What is a GitHub repository
- How to Create a Github pull request
- What is an API?
- Applications of Github
Introduction to GitHub
GitHub is a web-based platform that provides version control and collaboration tools for software development. It was founded in 2008 and is now owned by Microsoft.
GitHub allows developers to host and review code, manage projects, and build software. It also provides a range of tools for collaboration, including the ability to track and discuss issues, review code changes, and work with other developers on shared projects.
One of the main features of GitHub is its use of Git, a version control system that allows developers to track changes to their code and collaborate with others on projects. Git allows developers to create "branches" of code, which can be modified and merged back into the main project codebase. This allows for flexible and efficient collaboration, as developers can work on their own copies of the code without affecting the main project.
GitHub also provides a range of integrations with other tools and services, such as continuous integration platforms, bug tracking systems, and project management tools. This makes it an essential tool for many software development teams.
In addition to its code hosting and collaboration features, GitHub also provides a range of tools for community building, including the ability to create and participate in open source projects, follow other developers and organizations, and contribute to discussions and forums.
What is Git?
Git is a version control system that allows you to track changes to your files and collaborate with others on a project. It is a distributed version control system, which means that each copy of a repository is a complete copy of the entire project, including the full history of all changes made to the project.
What are its uses ?
-
Git stores your files and their changes as a series of snapshots, called commits, in a repository. Each commit contains a description of the changes made and a reference to the previous commit. This allows you to go back in time and see what your project looked like at any point in its history.
-
It also allows you to work on multiple versions of your project concurrently using branches. A branch is a copy of your project that you can work on without affecting the main version of your project. You can create as many branches as you need and switch between them easily. When you are ready, you can merge your changes back into the main branch.
-
Git is widely used by developers and teams to collaborate on software projects, as well as by individuals to manage their own files and projects. It is an open-source tool, which means that the source code is freely available and can be modified and distributed by anyone.
GitHub is a popular hosting service for Git repositories that provides additional features for collaboration and code review.
What is a GitHub repository :
A GitHub repository is a storage location where you can store your code, files, and documents. It is a central place where you can collaborate with other people on a project and track changes to your files over time. You can use a repository to store and manage your code, track changes you make to your code, and share your code with others.
How do you create a GitHub repository?
-
To create a repository on GitHub, you will need to create a free account on the website. Then, you can create a new repository by clicking the "New" button on your profile page. You can choose to make your repository public, which means that anyone can view and contribute to your code, or you can make it private, which means that only you and the people you invite can access the repository.
-
Once you have created your repository, you can add files to it by clicking the "Add files" button and selecting the files you want to include. You can then commit your changes, which saves them to the repository, and push your changes to the repository, which makes them available to others.
How to Create a Github pull request :
To create a new repository on GitHub with code and images using Python, you will need to use the GitHub REST API and the requests library.
Here are the steps you can follow:
-
Make sure you have a GitHub account and create a personal access token with the necessary permissions to create a repository and push code to it.
-
Install the requests library using pip install requests. Import the requests library and create a new variable to store your personal access token. Set up the base URL for the GitHub API and create a dictionary to store the data for your new repository.
-
Use the requests.post() function to send a POST request to the API endpoint to create a new repository. Pass in the base URL, your data dictionary, and your personal access token as arguments. If the repository was successfully created, the API will return a response with a status code of 201. You can check the status code of the response using the response.status_code attribute.
If the repository was not created, the API will return a response with a different status code. You can handle these errors by checking the status code and taking appropriate action.
-
Once you have created the repository, you can use the requests.put() function to push code and images to the repository. To do this, you will need to use the requests.put() function to send a PUT request to the API endpoint for creating or updating a file in the repository. Pass in the base URL, the file path, the file content, and your personal access token as arguments.
If the file was successfully added to the repository, the API will return a response with a status code of 201. You can check the status code of the response using the response.status_code attribute.
-
If the file was not added to the repository, the API will return a response with a different status code. You can handle these errors by checking the status code and taking appropriate action.
This is what a sample code looks like :
import requests
# Set up your personal access token and the base URL for the GitHub API
personal_access_token = "your_personal_access_token"
api_base_url = "https://api.github.com"
# Create a dictionary to store the data for your new repository
data = {
"name": "my-new-repository",
"description": "This is my new repository",
"private": False
}
# Send a POST request to the API endpoint to create a new repository
response = requests.post(f"{api_base_url}/user/repos", json=data, headers={
"Authorization": f"Bearer {personal_access_token}"
})
# Check the status code of the response
if response.status_code == 201:
print("Successfully created repository")
else:
print("Failed to create repository")
# Set the file path and content for the file you want to add to the repository
file_path = "path/to/file.txt"
file_content = "This is the content of the file"
# Send a PUT request to the API endpoint for creating
# or updating a file in the repository
response = requests.put(f"{api_base_url}/repos/{owner}/{repo}/contents/{file
What is an API? :
An API, or Application Programming Interface, is a set of rules, protocols, and tools for building software and applications. It specifies how software components should interact with each other, and it allows developers to access and use the functionality of other software components in their own applications.
APIs are often provided by web-based services, such as social media platforms, online payment systems, and e-commerce sites. These APIs allow developers to access and use the functionality of these services in their own applications, such as integrating a payment gateway into an e-commerce site or displaying social media posts on a blog.
How do API's Work ?
-
When an API is provided by a web-based service, it usually exposes specific functionality or data to external developers through a series of endpoints. An endpoint is a specific URL or address that represents a specific piece of functionality or data provided by the API. For example, an API for a social media platform might have an endpoint for retrieving user information, and another endpoint for posting updates to a user's timeline.
-
To access an API, developers typically send a request to one of these endpoints using a specific set of rules and protocols, such as HTTP or HTTPS. The request will include specific parameters or data, depending on the endpoint being accessed and the functionality being requested.
-
The API will then process the request and return a response to the developer's application. The response will typically include the requested data or the result of the requested operation, such as the user information or the status of a timeline update.
APIs are an important part of modern software development, as they allow developers to access and use the functionality of other software components in their own applications, and they enable the integration of different systems and services.
Applications of Github :
Some common applications of GitHub include:
-
Version control: GitHub allows developers to track changes to their code and collaborate with others on projects using Git, a version control system. This allows developers to create branches of code, modify and review code changes, and merge changes back into the main project codebase.
-
Collaboration: GitHub provides a range of tools for collaboration, including the ability to track and discuss issues, review code changes, and work with other developers on shared projects.
-
Open source development: GitHub is widely used for open source development, as it allows developers to easily share and collaborate on projects with others.
-
Project management: GitHub provides tools for project management, including the ability to assign tasks, track progress, and set deadlines.
-
Community building: GitHub provides a range of tools for community building, including the ability to create and participate in open source projects, follow other developers and organizations, and contribute to discussions and forums.
Overall, GitHub is an essential tool for many software development teams and a key resource for developers looking to collaborate and build software together.
In this article in OpenGenus, we learnt what an API is, what GitHub is, and how to create a succesfull pull request in Github.