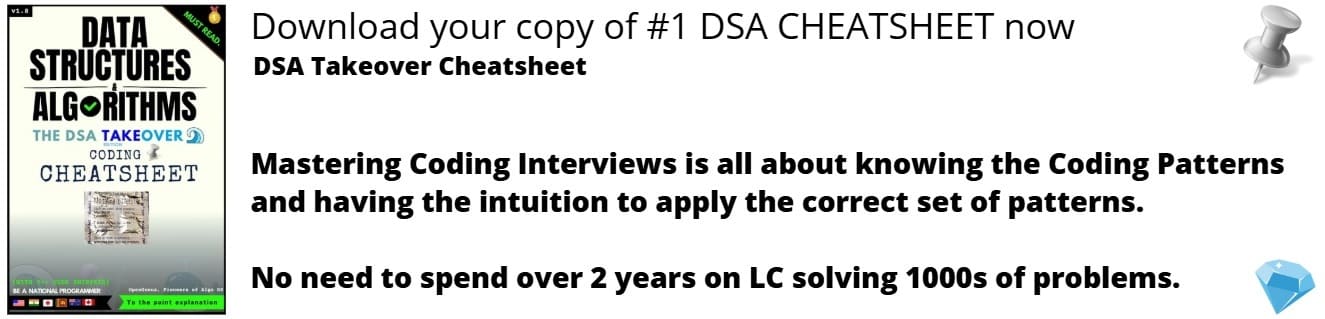
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will be exploring the System Requirements, Architecture, Low-level design, database design, and examples of School Management Software.
Table of Contents
- School Management System
- System Requirements
- Functional Requirements
- Non-functional Requirements
- Software Requirements
- Hardware Requirements
- System Design
- Architecture of the System
- Subsystem Decomposition
- Low-Level Design
- Database Design
- Examples of School Management Software
School Management System
School management systems are software designed to help schools to manage their academic and administrative activities seamlessly. It provides a complete set of features including managing Admissions and Fees activities, Staff management, Curriculum and Timetable management, and Performance reports.
System Requirements
The system we will be designing will provide the most important features that every school management system must offer. However, do note that a school management system can have other sub-systems for library management, sports, etc.
Functional Requirements
At the basic, every school management system must provide features like,
- Allow adding and removing teachers/staff details
- Allow adding and removing student details
- Manage payment of student fees and salary for faculty
- Get reports of student activities and faculty performance
- Allow adding of marks and attendance by faculty members
A better understanding of the functional requirements can be gained from the use-case diagram below.
Non - Functional Requirements
Security:
Only authorized users must be able to access the system and view and modify the data.
User Friendly:
The system should provide an interactive user-friendly interface that is easily understandable for all users.
Dependability:
The system should provide consistent performance with easy tracking of records and updating of records.
Maintainability:
The system should be easily maintainable and adding and removing new features must be very easy.
Software Requirements
Platform: Windows Server/ Linux
Language: Java/ C++/ Python/ any multithreading capable OOP based language
Database : Relational DBMS like MySQL/ PostgreSQL
Front-end Frameworks : Angular/ React/ Vue
Hardware Requirements
Processor: Intel Xeon X3 or better
Ram: 16 GB or greater
Disk Space: 1 TB or more
Network Connection: 15 Mbps or faster
System Design
Now that we have understood the functional and non-functional requirements of our system, let's take a look at the system architecture, decomposition, and database design.
Architecture of the System
Our school management system follows a simple 3 tier client/server architecture. The client can use web browsers to access the system through the local area network of the school or anywhere using the internet using the HTTPS protocol.
The middle tier which includes the server presents the website to the user and controls the business logic. It controls the interactions between the application and the user. The most common web server is Apache.
The data tier maintains the application's data such as student data, teacher data, timetable data, etc. It stores these data in a relational database management system (RDBMS) like MySQL. The client tier interacts with the server to make requests and retrieve data from the database. It then displays to the user the data retrieved from the server.
Subsystem Decomposition
Decomposing the system into smaller units called subsystems will help reduce the complexity of the system. Subsystems are just packages holding related classes. Our school management system is also decomposed into subsystems as follows. The major subsystems are 'Enrollment', 'Authentication', 'Assessment', 'Timetable', 'Attendance' and 'Report' systems.
The Enrollment subsystem registers a student or faculty offline. It allows recording the detailed information of the person including personal and emergency details. Users are classified into roles.
The Authentication subsystem authenticates a user to grant access based on the role of the user.
The Attendance system facilitates the recording of attendance of the student and faculty and also reports to the parents of the students.
The Timetable subsystem generates a timetable, which involves allocating a time slot to a subject teacher for a class of students.
The Assessment system deals with the exams and marks of the students for each subject.
The Report system handles generating of report cards which can be viewed by both the teachers and parents.
Low-Level Design
The following code shows some of the classes involved in School Management System Software.
class Person {
String name;
Integer age;
String sex;
String address;
Double phone;
}
class User extends Person {
Integer uid;
String email;
String role;
String password;
public void login(mail, passwd);
public void logout();
}
class Admin extends User {
public void addCourse(id, details);
public String removeCourse(id);
public void addFaculty(id, name, details);
public void modifyFaculty(id, field ,newValue);
public void addStudent(id, name, details);
public void modifyStudent(id, field ,newValue);
public void manageAttendance();
}
class Student extends User {
String grade;
Character section;
public void applyLeave(date);
public void checkMarks();
public void payFees(amount);
public void checkAttendance();
public void checkTimeTable();
public void raiseIssue();
}
class Faculty extends User {
String subjects[];
String grade;
public void addMarks(studentId, subjectId, assesmentId, marks);
public void addAttendance(studentId, isPresent, date);
public void addTimetable();
public void generateReports(studentId);
}
class Subject {
Integer sid;
String name;
public void displaySubject();
}
class Classroom {
Integer grade;
Character section;
ArrayList<Student> students;
ArrayList<Faculty> teachers;
public Pair<Integer,Character> getClassroomDetails();
public ArrayList<Students> getStudents();
public ArrayList<Faculty> getFaculty();
}
class Mark {
Subject s;
Student n;
Integer mark;
}
class Exam {
Integer Id;
Name String;
Mark m;
public Integer getmark(Student);
}
class Issues {
Integer issueID;
String type;
String description;
public void resoveIssue();
}
The Person class is the base class for all the users. The User class adds the necessary information for authenticating a user. The Admin class extends from the User class and has methods to add and remove courses, students, and staff members and also manage attendance.
The Student class and Faculty class also inherit from the User class. The Student class stores the grade and section information in addition to the inherited fields. A student can check their marks, timetable, attendance, apply for leave, pay fees and raise issues. The Faculty class also has information about the subjects that the faculty teaches. The faculty can add marks, attendance, timetable, and generate report cards for a student.
The Subject class contains information about a subject. The Issues raised by students are handled by the Issue class. resolveIssue() method can be called once the issue has been resolved. In addition to the above classes, we can also have separate classes for Transactions, Timetable, etc.
Database Design
A school management system software needs to store data about the students, faculty, assessments, attendance, etc. Therefore, we have identified the major tables that will be implemented on the selected RDBMS.
The above database diagram shows the schema for the school management software database.
The Student and Teacher tables store data of the students and faculty respectively. Generally, there are three types of relationships in a relational database system. These are one-to-one, one-to-many, and many-to-many relationships. The system under consideration has one-to-many and many-to-many relationships.
The Classroom tables stores information such as the section and grade. Each teacher is associated with one or many classrooms. Each classroom also has a Timetable associated with it. The Subject table stores the details about a specific subject and each classroom is associated with many subjects. The Classroom_Student table acts as a relation between the Student and Classroom tables and reduces anomalies.
The Exam table stores the details about a specific examination and has a one-to-many relationship with the Results table which stores the marks for a particular subject and a particular student for an exam.
Examples of School Management Software
Now we will have a brief look at some of the popular school management software available.
1. Fedena
Fedena is a school management software that is free and open source. It is used by thousands of educational institutions worldwide for all administration, management, and learning-related activities. It has more than 50 feature-rich modules for online classes, paperless admission, timetable, parent-teacher collaboration, etc. It is built using Ruby on Rails and is one of the most affordable school management software out there. The pricing starts at $459 per year.
2. Gradelink
Gradelink is a popular cloud-based school management software. It has a simple and easy-to-use interface. In addition to providing features such as attendance reporting, enrollment, and tracking marks it also provides easy integration with Google Classroom, Microsoft Office 365, and other software. Gradelink pricing starts at $103 per month for 0-50 students.
3. Campus 365
Campus 365 is an all-in-one school enterprise resource planning (ERP) software. It has features like paperless admission, exams and grade book, fees management, timetable, attendance, parents collaboration, and even an integrated library management module. It features a user-friendly interface and also RFID-based attendance.
4. Teachmint
Teachmint is a leading school management software providing features such as integrated ERP for schools, fee management, automatic attendance, academics management, etc. It has tools for parents to keep track of their children's performance and teachers to overcome global barriers to provide better education. It has a free basic plan and paid plans start from $2 per user for a year.