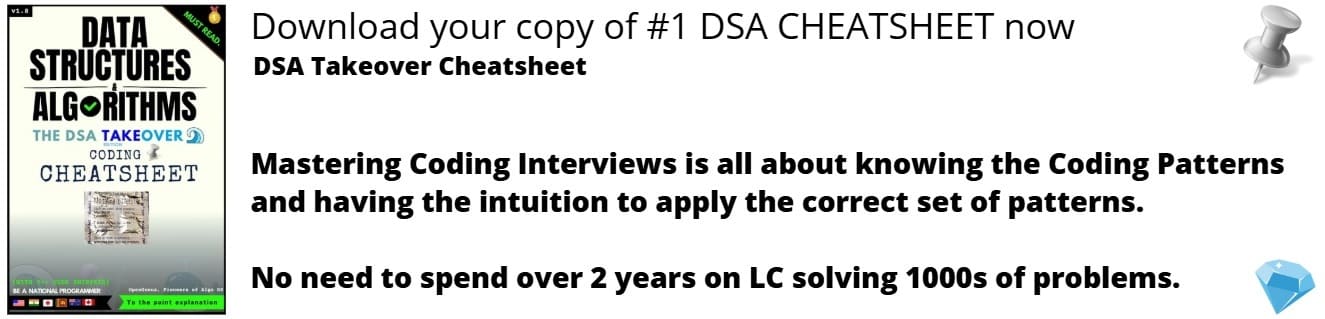
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will take a look at the key features a library management system needs to offer, its high-level, low-level design, database design, and some of the already existing library management software.
Table of Contents
- Library Management System
- System Requirements
- Functional Requirements
- Non - Functional Requirements
- Software Requirements
- High-Level Design
- For a small setting
- For a large setting
- Low-Level Design and Classes
- Database Design of Library Management System
- Leading Library Management Systems
Library Management System
A Library Management System is software that provides the ability to find books, manage books, track borrowed books, managing fines and bills all in one place. It helps the librarian manage the books and books borrowed by members and automates most of the library activities. It increases efficiency and reduces the cost needed for maintaining a library and saves time and effort for both the user and the librarian.
System Requirements
The key requirements that need to be offered by the library management system can be classified into functional and non-functional requirements.
Functional Requriements
- Allow the librarian to add and remove new members.
- Allow the user to search for books based on title, publication date, author, etc., and find their location in the library.
- Users can request, reserve, or renew a book.
- Librarian can add and manage the books.
- The system should notify the user and librarian about the overdue books.
- The system calculates the fine for overdue books on their return.
A more detailed list of key features that need to be supported by the system is given in the use case diagram.
There are 3 actors in the use case diagram. The User, The Librarian, and the System.
User - The user can log in, view the catalog, search for books, checkout, reserve, renew and return a book.
Librarian - The librarian registers new users, adds and maintains the books, collects fines for overdue books, and issues books to users who need them.
System - The system is the library management system itself. It keeps track of the borrowed books and sends notifications to the user and librarian about the overdue books.
Non - Functional Requirements
Usability
Usability is the main non-functional requirement for a library management system. The UI should be simple enough for everyone to understand and get the relevant information without any special training. Different languages can be provided based on the requirements.
Accuracy
Accuracy is another important non-functional requirement for the library management system. The data stored about the books and the fines calculated should be correct, consistent, and reliable.
Availability
The System should be available for the duration when the library operates and must be recovered within an hour or less if it fails. The system should respond to the requests within two seconds or less.
Maintainability
The software should be easily maintainable and adding new features and making changes to the software must be as simple as possible. In addition to this, the software must also be portable.
Software Requirements
- A server running Windows Server/Linux OS
- A multi-threading capable backend language like Java
- Front-end frameworks like Angular/React/Vue for the client
- Relational DBMS like MySQL, PostgreSQL, etc
- Containers and orchestration services like Kubernetes (for a large setting like a national library).
High Level Design
For a Small Setting (School/College):
In a school or college, The users are usually students and the faculty of the institution. Hence, the traffic is usually pretty low with an estimated 5,000 concurrent users at the peak. A group of servers each with 16GB of RAM and a capable processor should be able to handle the load. Each server may need to handle several requests at a time. The requests can be queued and a reply can be sent to the user while the request is being processed.
The user can access the system through a client site or app with a registered account. The librarian can access the admin dashboard and interface using the admin account.
Handling the authentication is done by a separate service. The authentication service has direct access to the accounts on the database. The notification service checks the database for any overdue books and alerts the user and the librarian. It can also be used by the server to notify about book reservations and cancellations.
Since the data stored are mostly relational, like user accounts, details on books, etc., a relational database like MySQL can be used. The database servers can be set up in master-slave configuration for backup and availability.
For a Large Setting (State/National Library):
These libraries are huge and are available to the general public. Anyone can visit these libraries. However, they may contain many important artifacts and rarely allow users to borrow books. The number of concurrent users can range from 100,000 for a state library to 10 Million for a national library at its peak.
These systems can also have separate user and admin interfaces for browsing books and managing users and books respectively.
The servers may be distributed across different regions in the country with load balancers to distribute the traffic. Proxy servers may be used to cache the requests and reduce the number of disk reads. Services such as authentication and notification may be deployed as separate containers. This allows scaling much more easily and simplifies the addition of new services.
The database can also be distributed with the regional data such as books of languages spoken in a region being stored in that region. Many government libraries preserve physical books in digital form and allow anyone to read them online without borrowing.
Low-Level Design and Classes
Now, let us take a look at a little bit lower-level design of the library management system by exploring the various classes and methods involved.
class Person{
String firstName;
String lastName;
String gender;
String Address;
Double phoneNo;
}
class Member extends Person{
int userId;
String email;
String password;
public void login(String email,String password);
public void logout();
}
class User extends Member{
List <Books> booksBorrowed;
Search searchBook;
public void payFine(int amount);
}
class Librarian extends Member{
String role;
BookIssueService issueService;
public void addBook(Book book);
public Book deleteBook(String book_id);
public Book updateBook(Book book);
}
class Library{
String name;
String Address;
List <Book> books;
}
The Person class is the base class and contains fields for the basic information such as name, address, etc. The Member class inherits the person class and adds fields such as a unique user id, login email, and password and also methods to login and logout. Both the User and Librarian classes inherit the Member class. The user can search for a book and pay a fine. The Librarian can issue books, add, delete or update books in the library. The Library class contains the name, address of the library along with the list of available books.
class Book{
int bookId;
String title;
String author;
String category;
Date pubDate;
Location location;
}
class Location{
int shelfNo;
int floorNo;
public List<int> getLocation();
}
class Search{
public List<Book> geBookByTitle(String title);
public List<Book> geBookByAuthor(String author);
public List<Book> geBookByCateogry(String bookType);
public List<Book> geBookByPublicationDate(Date publicationDate);
}
class BookIssueService {
public double calculateFine(int days,User user,Book book);
public BookReservationDetail reserveBook(Book book, User user);
public BookIssueDetail issueBook(Book book, User user);
public BookIssueDetail renewBook(Book book, User user);
public void returnBook(Book book, User user);
}
class BookLending {
Book book;
Date startDate;
User user;
}
class BookReservationDetail extends BookLending {
ReservationStatus reservationStatus;
}
class BookIssueDetail extends BookLending {
Date dueDate;
}
The Book class contains information about the book like its title, author, publication date, etc. The Location class is used to represent where the book is present in the library.
The Search class allows the user to search for books using various methods like author, title, category, publication date. The BookIssueService class has methods to handle reserve books, issue books, renew a book, and return a book. It also calculates the fine for overdue books.
Database Design in Library Management System
The above image shows one possible database schema for the library management system.
The users table stores the user details such as a unique user id, name, contact, and login information. The admin table references the user id as a foreign key and stores the role of the admin such as Librarian, Assistant, etc.
The details about the books are stored in the books table. It includes the book title, author, publisher, date of publication, category, etc. The category table contains more details about each of the categories that may help the reader. The shelf table contains the location of the book in the library which may include the shelf number and floor number.
The borrowing table contains the information about the book borrowed such as the id of the user who borrowed it, the date borrowed, the due date, etc. This information can be used to calculate the fine for overdue books. The records table stores every return made so that it can be referenced when needed. It also stores the fine amount paid if applicable.
Leading Library Management Systems
Leading Library Management Systems are:
- Destiny Library Manager
- CodeAchi
- Alexandria
- Koha
Destiny Library Manager
Follett's Destiny Library management software is one of the most used library management software in schools. Destiny library manager is a complete library management software that can be accessed from anywhere. It has a super-simple interface and allows librarians to keep a real-time track of the library's inventory. Follett offers the Destiny suite, including Destiny Library Manager, a library management system that also includes Destiny Analytics collections management and analytics, and Destiny Discover resource search tool.
CodeAchi
CodeAchi is a full-featured library management software with many options. It allows for searching of books through author, title, shelf, ISBN, and many other criteria. It supports barcode scanning and automatically calculates fines and notifies the borrower through mail and SMS. It allows librarians to export the borrowed books' data into excel and CSV files. Librarians can customize what data needs to be stored about the books and borrowers. It is a great choice for a university or a public library.
Alexandria
Alexandria is a browser-based library management software that is very popular around the world in both community and school libraries. This online Library Management system offers OPAC, Periodicals Management, Fee Collection, School Libraries, Serials Management in one place. Librarians can fine-tune the reports to exactly what they need. They also have fantastic customer service although it is paid.
Koha
Koha is a free and open-source library management software. Koha in Māori means gift or donation. Koha provides a web-based interface with customized report generation, search, social sharing, RSS feeds, online circulation, etc. In addition, Koha also supports a variety of languages. It uses a SQL database with MySQL or MariaDB being preferred. One disadvantage of Koha is that the software is not user-friendly compared to other software and may require training for the library staff to use it.
With this article at OpenGenus, you must have a strong idea of System Design of Library Management System.