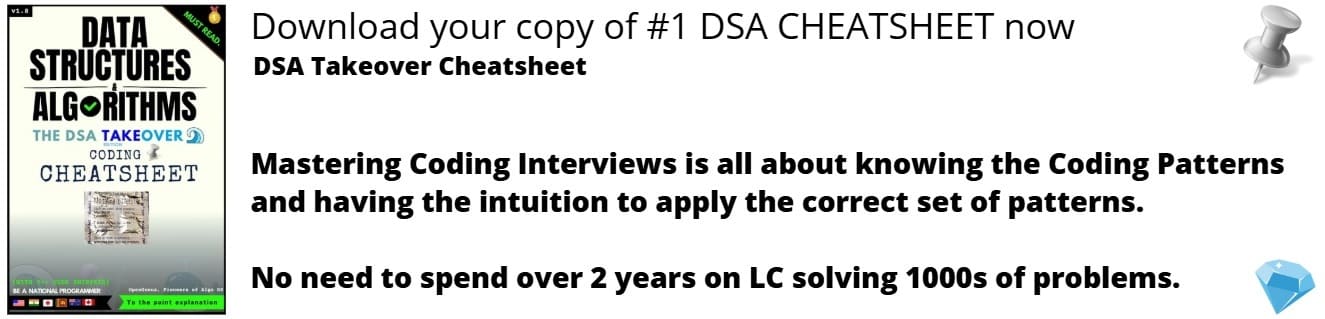
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Over the course of this article, you will be learning how to automate the sending of bulk messages with WhatsApp! A basic way which only uses 2 lines of code and a complete program so you can understand this process. This article is meant for all levels I will be going over two ways to do this.
Table of Contents:
- Setting Up Your System
- Using Pywhatkit
- Using Selenium
- Third Party Applications
- The Import Statements
- Connecting to Chrome
- Connecting to WhatsApp
- Other Files
- Putting it All Together
- Run The Code
1. Setting up Your System
As always, the most important part of any program is the set up and ensuring you have everything required to get started. For this article we will need python, an IDE (I use Pycharm), and PyWhatKit. We will be going over how to install PyWhatKit next, the other two you should search how to videos for installing if not already installed. First we will be making a folder and calling it "bulk_automate_whatsapp" Next we will make our python file called "whatsapp.py" or whatever you would like to name it.
2. Using PyWhatKit
Installing PywhatKit:
I always enjoy doing my installations through the command prompt, but there is another way. If you do get stuck with the command line installation here is the link to there site where they go more in depth click here. Copy the command depending on your system.
Mac or Linux:
pip3 install pywhatkit
Windows:
pip install pywhatkit
The Code
import pywhatkit
pywhatkit.sendwhatmsg('+phone number', "Hello automated world", 14, 20)
The first line imports the PyWhatKit module. The next line calls upon the sendwhatmsg function, which sends the message on WhatsApp. The first argument will be the phone number you would like to send to, then the message, followed by the time in 24 hours, and lastly the minutes of the time you want the message to send at. That is all that is needed, but if you want to learn how to do this and make a more customizable program, keep reading.
3. Using Selenium
Using a third party app that has already created code can be a good way to learn if you look at how that code works. Or if you just want to make the porgram quick and utilize it's functions. If you want to know how to send a bulk message with WhatsApp then we need to make our own program and use functions to make it customizable. In order to do this, we will be using some 3rd party applications like Selenium and ChromeDriverto connect our code to the web.
4. Third party Applications
ChromeDriver
The first 3rd party application we will be using is called ChromeDriver You can download the program here.
On the page choose the appropriate link for your Chrome version. Then you will choose the appropriate link according to your system. If you are on windows the 32bit link works regardless. You will then be downloading a zip file. Once downloaded we will move this zip file and extract it into the folder we have created. If you get stuck here, here is a video of how to download ChromeDriver for windows and for Mac.
Selenium
Selenium is a great program for working with automation and web applications, we will be using it for these exact purposes. The installation process for selenium is simple, you open the command prompt by searching up "cmd" on your computer. Open the command prompt and enter the following command:
pip install selenium
4. The Import Statements
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.keys import Keys
import time
import pyperclip
If the following doesn't make sense yet, come back to this section after so you can see how these lines were used. These are all of the import statements you need for the following program. The first line is needed to bring in the webdriver extension, this allows for our program to open and connect to Chrome. The next statement is required to allow for searching of elements by a certain property. The webDriverWait allows for our program to wait for the webpage to be loaded before moving forward in the code. The next line is for the expected conditions to be met with the WebDriverWait function. It works with that module. The importing of Keys is essentially importing the keyboard to tell the computer which keys to hit. The time module is for buffering just in case. Lastly pyperclip is used to allow for copying and pasting and clipboard type functions.
5. Connecting To Chrome
The first step of any program like this is to connect to your browser so you can control it. Essentially in this program we will be informing the computer where to press, what to input, and how. Just as if we were doing it manually with our mouse and keyboard. So the first step when I want to message someone on my laptop with WhatsApp is to open Chrome. We will be doing this with the following code:
web_browser = webdriver.Chrome()
If you run this code it should open a tab in Chrome. Understanding this part of the code in more depth is out of the scope of this article. All you need to know is we have created an instance of Chrome using the function webdriver.Chrome(). So when we want to connect to Chrome now we use the variable web_browser.
6. Connecting To WhatsApp
The next step in our process is to open WhatsApp and we can do this with the following line of code.
web_browser.get("https://web.whatsapp.com/")
Put all together the code will open a Chrome page of WhatsApp bringing up an issue, the QR code. Unfortunately, there is not much we can do, when we want to use the app, we need to scan and login first. Once we are logged in the next step is to search for the group I want to message. We will be creating a variable that is attached to this search bar. In order to to do this we will be taking the XPath of the search box. The XPath is the code that points to an element in this case it is the search box. To get the XPath of the search box you need to right click on the search box and click inspect. This will bring up the html right click on the highlighted section and select copy then select XPath. Once we have this, we input it into our code like so.
search_xpath = "//*[@id=\"side\"]/div[1]/div/label/div/div[2]"
searchbox = web_browser.find_element(By.XPATH, "")
Now the variable search box is set as the search box on WhatsApp. As stated above we are recreating what it would be like to physically send bulk messages on WhatsApp. You will notice we will be saving certain elements as variables, such as the search box. Keep them so we can manipulate these elements to do what we want. The next element we need to grab is the group in which we search and click to message this group. Try to code out this next part by yourself. If you get stuck the answer is below.
test1_xpath = "//*[@id=\"pane-side\"]/div[1]/div/div/div[1]/div/div/div[2]/div[1]/div[1]/span/span"
group_title = web_browser.find_element(By.XPATH, test1_xpath)
Now group_title is saved with the group we want to click. In this case it is the first group which is test1. We need to grab one more element which is the message bar where we will be putting our message and hitting enter, done like this.
input_xpath = "//*[@id=\"main\"]/footer/div[1]/div/span[2]/div/div[2]/div[1]/div/div[2]"
input_box = web_browser.find_element(By.XPATH, input_xpath)
input_box is set to the message box and we have every element we need to do this project. Sending bulk messages means we have multiple people/groups in which we want to send to. For this I made two files one to hold all the group names I want to send to and one for the message to send. Let's set up these two files with our program.
7. Creating The Other Files
Beginning with "recipients.txt" this is what I made my filename. In this file each line will be a different group or person. For example, my file looks like this:
test1
test2
test3
We save this file and put it in the same directory as the other files created. Next we have our "message.txt" with the same concept, but there is only one message more than one message is out of the scope of this article, however once you have this program set up, that is a great exercise to do to see if you understand this concept. No variable is needed, but the message should be in quotes in the file. My message is "Hello, testing". It does not have to be complex as it is just a test.
8. Reading The Other Files
Next we will be going over how to read in files to our program. Using the With function we are going to open the file gather all the data and save it into an array I called groups. This looks like the following.
with open('recipients.txt', 'r') as f:
groups = [group.strip() for group in f.readlines()]
The first argument is the file you want to open, second how you want to open the file in this case we want to read the file and is what the 'r' represents. The readlines() function tells the program to return everything as a list. After the use of the .strip() function returns a copy of the string without spaces for each element in "recipients.txt". Then we do the same for the "message.txt".
with open('message.txt', 'r') as f:
msg = f.read()
Since it is only one line all we need to do is read in the line and save it to a variable I called "msg".
9. Putting it all together
We will be using a for loop to loop through each element in the "recipients.txt". In each loop we wait for the application to load, clear the search box and paste in our group, wait for buffering, find and click on the group, wait for buffering, find the message box input our message and hit enter. This looks like the following:
for group in groups:
search_xpath = "//*[@id=\"side\"]/div[1]/div/label/div/div[2]"
search_box = WebDriverWait(web_browser, 500).until(
EC.presence_of_element_located((By.XPATH, search_xpath))
)
search_box.clear()
pyperclip.copy(group)
search_box.send_keys(Keys.CONTROL + "v")
time.sleep(2)
test1_xpath = '//*[@id="pane-side"]/div[1]/div/div/div[1]/div/div/div[2]/div[1]/div[1]/span/span'
group_title = web_browser.find_element(By.XPATH, test1_xpath)
group_title.click()
time.sleep(1)
input_xpath = "//*[@id=\"main\"]/footer/div[1]/div/span[2]/div/div[2]/div[1]/div/div[2]"
input_box = web_browser.find_element(By.XPATH, input_xpath)
pyperclip.copy(msg)
input_box.send_keys(Keys.CONTROL + "v")
input_box.send_keys(Keys.ENTER)
time.sleep(2)
If pyperclip is not already installed, you can install the program with the following command.
pip3 install pyperclip
10. Run The Code
You are finally done and ready to run the code! In the terminal run the command to run your code. Now watch your computer come to life and send out as many messages as you want. Congratulations and good luck with your new program and knowledge.
With this article at OpenGenus, you must have the complete idea of how to develop a Python Script to Send Bulk WhatsApp Message.