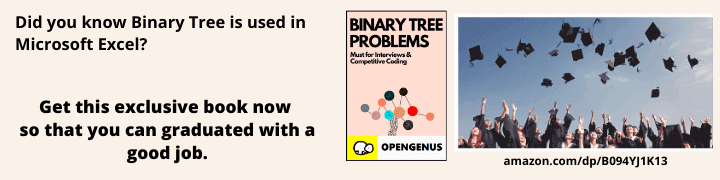
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Template class in C++ is a feature that allows a programmer to write generic classes and functions which is useful as the same class/ function can handle data of multiple data types.
First, we need to know that C++ supports the use of Generic classes and Funtions. So what is this generic programming and how do we use it?
Generic Classes
Generic programming helps us to code and write our programs in such a way that they are independent of data type, yes independent of data type. Usually in programming we hadle different kinds of data with different data type and very often we might need to write the same lines of code each and every time because of change in data type although the functionality or aim of the program is same. Hence we can use the generic classes that act as templates and support any kind of data type.
Example class :
Consider a simple program in C++ like this one,
#include <iostream>
using namespace std;
//class
class Arithmetic
{ //data members
private:
int a;
int b;
public: //member functions
//contructor
Arithmetic(int a, int b)
{
this - > a = a;
this - > b = b;
}
//function
int add()
{
int c;
c = a + b;
return c;
}
};
int main()
{
Arithmetic a(10, 5);
cout << a.add();
return 0;
}
All we are trying to do is just add two numbers, now this is very easy and works fine in the same way we can write functions to perform all kinds of arthematic operations but what if we want to add two decimal numbers i.e of data type float or double or anything, yes we will have to re-write the same logic again from the beginning. But wait what if we make this class a template class in such a way that it can apply the same logic to different types of input irrespective of their data types.
What is a Template Class
In simple terms as indicated by the name it acts as template, and as we have discussed above about generic classes/functions, templates is a feature in C++ using which we can implement generic functions i.e use the same programming logic even if there is a change in the data-type. Template classes ultimate goal or purpose is to make us code and use the same classes and functions to work effectively in the same way on different data types.
Why use a Template Class
We might have come across many scenarios while programming, where we different function and classes with the same logic but just beacuse of change in data-type say int / float we have to re-write the exact some code again and call differnt functions according to their data-type. This adds a lot of compute time, run-time, memory and for us to think, type and ,implement it. Instead the whole process is solved just by implementing templates. Saving a lot of time, energy and making the whole programming process a lot more efficient.
Disadvantages of not using template classes:
- Increased compile time, run time
- More lines of code and memory resources
- Same code logic is repeated for different data-types
Important syntax rules for Templates in C++ :
template <class T>
should be used before class declaration or while defiing member function outside the class.- All the variables should be declared as data type
T
- Even the return type of a funtion should be of type
T
- While initializing class objects in the main function it should be in the following format.
class_name <data_type> object_name(data_members);
Example :
Arithmetic<int>a(10,5);
And when we want to use another data type just mention the data type
Example :
Arithmetic<float>b(2.5,1.3);
Using Template Class
#include<iostream>
using namespace std;
//before class we need to specify that it's a template class
template<class T>
class Arithmetic
{
private:
/*notice the data type of data member of class they are of type T */
T a;
T b;
public:
//same again data types of type T
Arithmetic(T a,T b);
T add();
};
/*whenever we use/define template functions, we need to mention the templat <class T>*/
template<class T>
Arithmetic<T>::Arithmetic(T a,T b)
{
this->a=a;
this->b=b;
}
//even the return type sould of type template T
template<class T>
T Arithmetic<T>::add()
{
T c;
c=a+b;
return c;
}
int main()
{
/*while intializing object of class we need to mention the type*/
Arithmetic<int>a(10,5);
cout<<a.add()<<endl;
//same is re-used for float type or any other data-type
Arithmetic<float>b(2.5,1.3);
cout<<b.add();
}
Now the above program does the same as previous one but using the concept of template class we can re-use the same code to process any kind of data-type.
Advantages of Templates in C++
- Code repetition is reduced as same code is re-used
- This results in smaller compile and execution times
- Implements the concepts of Polymorphism(reusability)
- Good design and structure changes can be made easily with less number of errors and confusion
- Saves time, safe and secure code is obtained easily
- Increases performance which helps us in building powerful libraries
Thoughts
Using templates in C++ will give us mutiple advantages saving time, memory and lines of code at the same time they should be used only when needed and the code should always be easy to read and understand the logic.
With this article at OpenGenus, you must have the complete idea of template classes in C++. Enjoy.