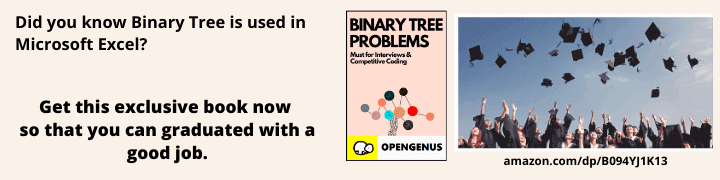
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Generally, Java programs terminate when they reach the end of the program, but there may be times when we need to know how to terminate a program when a certain condition is met or an exception occurs.
Table of contents:
- Ways To Terminate Program in Java
- The exit() Method
- Return Statement
- The Halt Method
Prerequisite: Basics of Java in one post
Let us get started with Different ways to terminate program in Java.
Ways To Terminate Program in Java
Terminating a program means to stop the execution of the program and more specifically, kill the process that was executing the program. This can be done from the terminal executing the program but can also be done from within the Java code.
Different ways to terminate program in Java are:
- Using exit() Method
- Return Keyword
- Halting the JVM itself using the Halt Method
The exit() Method
The System class contains several useful class fields and methods.Among the facilities provided by the System class are standard input, standard output, and error output streams; access to externally defined properties and environment variables; a means of loading files and libraries; and a utility method for quickly copying a portion of an array.One such important method provided by System class is exit().This method terminates the currently running Java Virtual Machine.It takes a status code as a parameter.This method doesn't return any value. Exit can be disabled by the SecurityManager class.
Syntax :
System.exit(int status)
Status values can be used to denote the outcome of the Program
- 0 is used when execution went fine.
- Any other integer value when some error has occurred. Different values can denote different kinds of Errors.
Example:
import java.util.*;
class Example{
public static void main(String[] args)
{
System.out.println("Hello , You've reached the first print statement");
System.exit(0);
System.out.println("Second Println here");
}
}
Output:
Hello , You've reached the first print statement
from the above example we can see that after the exit() method is called , JVM will terminate , the lines beyond this call are not executed.
The System.exit() call internally invokes the Runtime.getRuntime().exit(0).
Return Statement
Return statement is usually used to return a value from a function incase the function returns any value , else it is used to terminate a function if it returns void.Return would cause a program to exit , if it's present inside the main method of a class. If you add more code after return, the compiler will complain about unreachable code.
class Example{
public static void Main(String[] args)
{
if(args.length < 2)
{
System.out.println("Expecting more arguments");
return;
}
for(String s : args)
{
System.out.println(s);
}
}
}
Execution :
java Example hello
Output:
Expecting more arguments
from the above program, we can clearly see that return can be used to exit a program only if it's used inside the main method. What happens if we put some code after return? Let's see.
class Demo{
public static void Main(String args[])
{
System.out.println("Code After Return?");
return;
System.out.println("Cannot print this or even execute");
}
}
when we try to execute this program , we'll get the following error.
Demo.java:6:error: unreachable statement
System.out.println(“Cannot print this or even exeute”);
^
1 error
Thus , Return is only viable as a way to exit when it's inside the Main method.
The Halt Method
Every Java application has a single instance of class Runtime that allows the application to interface with the environment in which the application is running.
This class has various methods to interact with the environment in which the Java virtual Machine is Running. Few uses of this class include:
- Used to get Current Runtime.
- Can be used to find the Free memory in Java Virtual Machine.
- Exit the running Java Virtual Machine by initiating the Shutdown Sequence.
- Can be used to forcibly terminate the currently running Java Virtual Machine.
It is the halt method of this Runtime class that we're interested in. When you call the exit method , the Shutdown sequence is started , Shutdown Hooks or Exit finalizers are called. These Hooks are pieces of code to be executed when the JVM is shutting down. The Halt method forcibly terminates the JVM , hence no hooks are called. Halt method should be used with extreme caution as it abruptly terminates the JVM. Halt is rarely used . Halt can be disabled by the SecurityManager class.
Syntax:
Runtime.getRuntime().halt(int status);
Just like the exit() method , the status can indicate Success or Failure.
class HaltDemo{
public static void Main(String args[])
{
System.out.println("Forcibly Terminating JVM");
Runtime.getRuntime().halt(0);
// Won't Execute as The JVM has terminated.
Syste,.out.println("Are we Stil running");
}
}
Output:
Forcibly Terminating JVM
Thus , Halt should only be used when we know what we're doing.
Conclusion
In most circumstances, a program will terminate itself when it reaches the end, but in unusual circumstances, such as exceptions, a developer must know how to terminate a program. Java provides several ways to terminate a program. These include the above mentioned topics. Developers must be cautious when using these termination methods.
With this article at OpenGenus, you must have the complete knowledge to terminate a program in Java using different techniques.