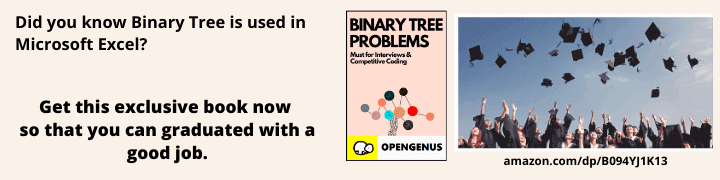
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
C++ is a very commonly used programming language which is used by many users who are active in "Competitive programming" and if they want to learn "Data structures and Algorithms" concepts and want to master in technical interviews for their dream job.
As C++ language is widely used nowadays but while writing its code you have to include some header files and a line "using namespace std;".But do you know including this particular line in a C++ code or a program is not considered a good practice? Let's see why and how this particular line is bad.
C++ contains a standard library that includes common functionality you use in building your applications like containers, algorithms, data structures etc. If names used by these functionality were out in the open, for example, if they defined a random class, suppose they defined a "stack" class globally, you'd never be able to use the same name again without conflicts. So they created a "namespace, std" to contain this change. The "using namespace" statement or line just means that in the particular scope it is present, make all the things under the "std namespace" available without having to use prefix "std::" before each of them.Pulling in the entire "std namespace" into the global namespace is not good as it defeats the purpose of namespaces and can lead to name collisions. This situation is called "namespace pollution".
The statement "using namespace std;" is generally considered bad practice. The alternative to this statement is to specify the namespace to which the identifier belongs using the scope operator(::) each time we declare a type. Although the statement saves us from typing std:: whenever we wish to access a class or type defined in the std namespace, it imports the entirety of the std namespace into the current namespace of the program. Let us see some examples that why using this line is not considered a good practice.
Now if someone wants to use output function in C++ "cout" from std namespace,so what will be the code?Let's see."//" this means adding comments in our code as it makes the code more readable and efficient for others to understand.
//first we include relevant header files
#include <iostream>
using namespace std; //this is the line we have to use in our code to execute it
cout<<"HELLO!"; //this will print hello in the output terminal
Now for instance after some time we wish to use another another sample or version of cout that is implemented in another library suppose we call it as "hello.h".We have to include that header file or library also. You will clearly understand after seeing this example.
//include header files
# include <hello.h>
# include <iostream>
using namespace std;
cout<<"HELLO!";
Now you have observered that there is an ambivalence or uncertainty, to which library does cout point to? The compiler may detect this and unable to compile the program or a given code. In the worst case, the program may still compile but call the wrong function, because we have not specified to which namespace the identifier belonged. So now you all have a bit of clear understanding of "why using namespace std;" is considered a bad practice. Now let's see further.
Namespaces were introduced into C++ to resolve identifier name issues,problems or conflicts. This ensured that two objects can have the same name and yet be treated in a different manner as if they belonged to different namespaces. You must have observed and noticed how the exact opposite has occurred in the above explained example. Instead of resolving a name conflict, we actually create a naming conflict.
When we include or import a namespace we are essentially pulling all type of definitions into the current scope. The std namespace is very large and big. It has hundreds of predefined identifiers, so it is quite possible that a developer or a user may overlook or ignore the fact that there is another definition of their intended object in the std library. Being not aware of this they may proceed to specify their own implementation and expect it to be used in later parts of the program. Thus there would exist two definitions for the same type in the current namespace. This is not allowed in C++, and even if the program compiles or executes there is no way of knowing which definition is being used where.The solution to the problem is to explicitly specify to which namespace our identifier belongs to using the scope operator "::". Now let's see an example of how to resolve this problem using this particular operator.
//include header files
#include <hello>
#include <iostream>
std::cout<<"Something to display"; // Using cout of std library
hello::cout<<"Something to display"; // Using cout of hello library
But having to type "std::" each every time we define a type is monotonous. It also makes our code look very inefficient and lengthy with lots of type definitions and makes it very difficult for the reader to judge or read the code. The source code that is messed up with complicated,complex and long type definitions is very difficult to read. This is something developers or users seek to avoid or ignore because code maintainability is a very important factor and they consider this thing very keenly. There are a few ways to resolve this dilemma or issue by specifying exact namespace without messing up the code with std keywords. Now we will some methods through which we can easily resolve this issue.
- Using "typedefs": Typedefs can easily resolve this issue as they can save us from writing long type definitions. Now just see this below mentioned example,it is just the modification of the previously used example where we are using the two libraries.
//include header files
#include <hello>
#include <iostream>
typedef std::cout cout_std;
typedef hello::cout cout_hello;
cout_std<<"HELLO!";
cout_hello<<"HELLO!";
Now seeing this example we can observe that now the program is looking a bit understandable.
- "Try to import namespace in limited scope not in global scope": If we will import namespace inside functions or in limited scope, the namespace definitions get imported into a local scope, and we at least know where possible errors may originate if they do arise. You get clear understanding after seeing this example code.
//include header files
#include <iostream>
void hello()
{
using namespace std; //use inside any function
// Now proceed with function
}
With this article at OpenGenus, I am sure you all have a clear view of why using namespace std; in a code is not a good practice. While developing a code or any applications it is quite necessary for the developer to be aware of all the issues that may arise in their code and know the solutions to resolve them so that their code will be easy, efficient, unambiguous, readable and error free.