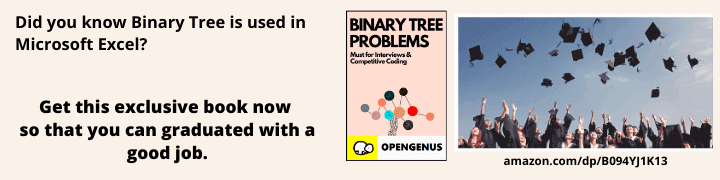
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the idea of Scope Resolution operator :: in C++ which is used widely in C++ implementations to access a class inside another class, in multiple inheritance and many more.
Table of contents:
- Basics of Scope Resolution :: in C++
- Implementation of C++ example using scope resolution
- Different ways to use Scope Resolution :: in C++
- To define a function outside a class
- To access a class’s static variables
- In case of multiple Inheritance
- For namespace
- Refer to a class inside another class
- Applications
Let us get started with Scope Resolution :: in C++ Programming Language.
Basics of Scope Resolution :: in C++
A scope resolution operator '::' is an operator which helps to identify and specify the context to which an identifier refers, particularly by specifying a namespace or one can also say it is used to qualify hidden names so that you can still use them. You can use the unary scope operator if a namespace scope or global scope name is hidden by an explicit declaration of the same name in a block or class.
EXAMPLE-
If you have a global variable of name Apple and a local variable of name Apple, to access global Apple , you'll need to use the scope resolution operator.
Implementation of C++ example using scope resolution
#include<bits/stdc++.h>
using namespace std;
string name="OPENGENUS";
// not decalred in any function known as global
int main()
{
string name; // declared inside a main function known as local
cin >> name;
cout << "\nThe name you entered will be displayed "
<< name << endl;
// Using Scope Resolution :: in C++
cout << "The name which you declared globally will be displayed "
<< ::name << endl;
return 0;
}
OUTPUT:
opengenus
The name you entered will be displayed opengenus
The name which you declared globally will be displayed OPENGENUS
Different ways to use Scope Resolution :: in C++
Scope Resolution :: in C++ can be used as:
- To define a function outside a class
- To access a class’s static variables
- In case of multiple Inheritance
- For namespace
- Refer to a class inside another class
IT CAN ALSO BE USED FOR FOLLWING PURPOSES AS:
1. To define a function outside a class
When we declare a function inside a class, which works on the data members of the class. Now to access that declared function outside the definition of the class for its further definition, implementation or just calling the function , we use scope resolution operator.
scope resolution operator :: is used to define a function outside a class
#include<bits/stdc++.h>
using namespace std;
class Dog
{
public:
// Only declaration of the properties of Dog class
void colour();
int size();
};
// Now here we are given defination to the decalred property colour in the class Dog
void Dog :: colour()
{
cout<<"brown"<<endl;
}
int Dog :: size()
{
cout<<"3"<<endl;
return 0;
}
int main()
{
Dog goofy; // creating an object of the class Dog
goofy.colour(); // here getting the colour of goofy
goofy.size();// size of goofy
return 0;
}
OUTPUT
brown
3
2. To access a class’s static variables
Let's consider a situation where you have the static variable of the name "x=3" and a local variable of the name "x=4" and we do know the fact that the value of static variable never changes, but in a function having a local variable "x=4" where we want to access the static variable with value "x=3" we will use the operator otherwise it would give us a value of local variable i.e "x=4".
#include<bits/stdc++.h>
using namespace std;
class Dog
{
static string name;
public:
static int size;
// what happens here is loacl parameter name value will be displayed
// everytime inside this function despite of the fact that a static variable of it already exsits
// so in this case we can acces the static variable using "::"
void func(string name)
{
// We can access class's static variable
// even if there is a local variable
cout << "Value of static name is " << Dog::name<<endl;
cout << "Value of local name is " << name<<endl;
}
};
// In C++, static members must be explicitly defined
// like this
string Dog::name = "scooby";
int Dog :: size = 4;
int main()
{
Dog d; // creating object of the class
string name;
cin>>name;
d.func(name);
cout <<"Dispalying the size of static variable size = " << Dog::size;
return 0;
}
OUTPUT
goofy
Value of static name is scooby
Value of local name is goofy
Dispalying the size of static variable size = 4
3. In case of multiple Inheritance
Let's suppose we have a parent class and it has two children (two different classes for two children) and now we want to know the gender and age of both so we need two functions displaying age and gender for both the children in their respective classes so to access both the children's age and gender uniquely we would use a scope resolution operator.
// Use of scope resolution operator in multiple inheritance.
#include<bits/stdc++.h>
using namespace std;
class Anime
{
public:
string name;
int episodes;
void getdata(string x, int y) //SAME NAME
{
name = x;
episodes = y;
}
void display()
{
cout << "Anime is" << name <<" with "<<episodes<<"episodes"<< endl;
}
};
class Character
{
public:
string name;
void getdata(string q) // SAME NAME
{
name = q;
}
void display()
{
cout << "Name of Favorite character" <<name<< endl;
}
};
// multiple classes getting inherited by details class
class details: public Anime, public Character
{
public:
void display()
{
Anime::getdata("AOT", 70); // used to getdata of anime class
Anime::display();
Character::getdata("LEVI"); // used to getdata of character class
Character::display();
}
};
int main()
{
details d;
d.display();
return 0;
}
OUTPUT
Anime is AOT with 70 episodes
Name of Favorite character LEVI
4. For namespace
If a class having the same name exists inside two namespaces we can use the namespace name with the scope resolution operator to refer to that class without any conflicts.
// Use of scope resolution operator for namespace.
#include<bits/stdc++.h>
int main(){
// all these std :: was taken care by namespace but not defined so to avoid conflicts.
std::cout << "Hello World" << std::endl;
}
5. Refer to a class inside another class
Let us consider we have food (parent class) where we have different classes inside our parent class (i.e a class inside another class) so in that case, to access a class that is present inside another class we will use scope resolution operator.
#include<bits/stdc++.h>
using namespace std;
class Colours // main class
{
public:
static string x;
class Light // another class inside main class
{
public:
string x;
static string y;
};
class Dark // another cass inside main class
{
public:
string x;
static string y;
};
};
string Colours ::x="Blue";
string Colours::Light::y = "Sky blue"; // value of class light
string Colours::Dark:: y="Dark blue"; // value of class dark
int main()
{
Colours A;
Colours::Light B;
Colours::Dark C;
cout<<A.x<<endl; // displaying values of main class
cout<< B.y<<endl; // displaying value of light class inside main class
cout<<C.y<<endl; // dispalying value of Dark class inside main class
return 0;
}
OUTPUT
Blue
Sky blue
Dark blue
Applications
- To access the value of a global variable inside a function with a local variable of the same name.
- It is also used to define and call a function outside the definition of the class.
- It is possible and usually necessary for the library programmer to define the member functions outside their respective classes.
With this article at OpenGenus, you must have the complete idea of using Scope Resolution :: operator in C++.