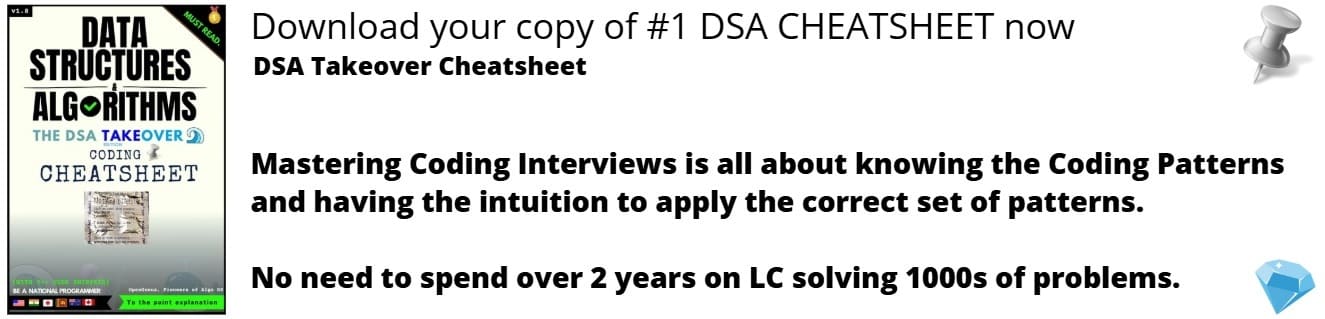
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about pop_back() method of Vector class included in std::vector library. Vectors can be considered as dynamic arrays on the basis of its usage. The elements are added and deleted dynamically changing the the size of the vector at runtime which allows users to prefer the vectors over arrays when they want to control the memory or size of the array at runtime. You need to include <vector>
library for its usage.
vector::pop_back()
Deletes the last element from the vector
This method in vector allows to remove the last element and destroy the memory allocated to the last element of the vector reducing the size of the vector by one.
- Expected Parameters: None
- Return Value: None
- Syntax:
vector_name.pop_back();
Examples:
In the below example, we will try to take a look at the basic usage of the vectors where we will simply output the vector before and after the pop_back() method.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vec = {10,20,30,40,50};
// Outputting the Elements of the vector before pop_back()
for(auto i: vec){
cout<<i<<" ";
}
cout<<endl;
vec.pop_back();
// Outputting the Elements of the vector after pop_back()
for(auto i: vec){
cout<<i<<" ";
}
cout<<endl;
return 0;
}
Output:
Elements before pop_back()
Output: 10 20 30 40 50
Elements after pop_back()
Output: 10 20 30 40
In the next example we will see the similar usage where we will try to delete all the elements of the vector untill it's empty.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vec = {100, 200, 300, 400, 500, 600, 700, 800, 900, 1000};
// Using iterator to print the elements of the vector
for(auto it = vec.begin(), it!=vec.end();it++){
cout<< *it<< " ";
}
cout<<endl;
// Output the size of the vector
cout<< vec.size()<<endl;
// Deleting all the elements of the vectors untill its size is 0
while(!vec.empty()){
vec.pop_back();
}
// Output the size of the vector
cout<<vec.size()<<endl;
return 0;
}
Output:
Output:
100 200 300 400 500 600 700 800 900 1000
10
0
Conclusion:
Vector is very handy data structure especiall then when the user is required to allocate and dellocate the elements dynamically with ease.