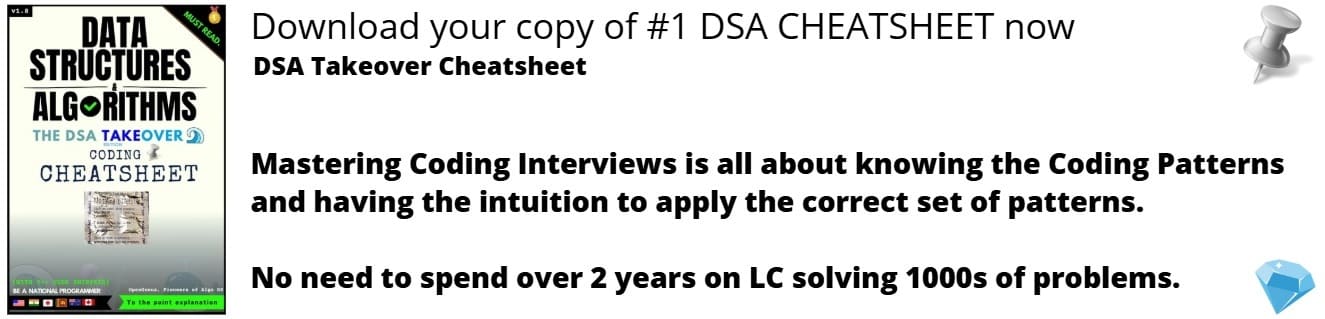
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Vector::size() in C++ STL is used to get the size of a vector container that is the number of elements it has. We have compared it with empty() and capacity() functions as well which are related.
In short, it is called as follows:
int size = vector_name.size()
In c++ STL, there are two types of containers:
- Sequential Containers
- Container Adaptors
Vector is a sequential container that stores objects and data. vector can be seen as dynamic arrays, beacuse vectors are dynamic there is no constraint on the size of a vector.As an element is inserted or deleted vector has the capacity to resize itself accordingly.
size()
size function is used to return the number of elements in a given vector or in other words it returns the size of the vector.
Parameters passed
No parameters are passed in the size function.
Return Values
size() returns the integer value containing.
Syntax
vector_name.size()
Complexity
time complexity: Big O(1)
Example
Here is an example of use of the size function.
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vect;
vect.push_back(10);
vect.push_back(1);
vect.push_back(3);
vect.push_back(9);
vect.push_back(18);
vect.push_back(2);
vect.push_back(14);
cout<<vect.size();
return 0;
}
Output:
7
Explanation of the code
The above code explains the working of the size() function. The push_back() function adds the elements to the vector one be one , 7 elements in total and the size() function is used to check the size of the vector that comes out to be 7 as shown in the output.
Comparison with other functions
size() vs capacity()
The capacity() is the memory space that a vector is using currently.Capacity may or may not be equal to the vector size. It is always equal to or greater than the size of the vector.
The main difference between the size() and capacity() is that the size is exaclty the number of elements in a given vector whereas the capacity is maximum number of elements a vector can have without the reallocation of the memory. When this limit is reached then the capacity is expanded automatically.
Example
#include <iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vect;
for (int i = 1; i < 6; i++)
{
vect.push_back(i);
}
cout << "The size of vector is " << vect.size();
cout << "\nThe maximum capacity is " << vect.capacity();
return 0;
}
Output:
The size of vector is 5
The maximum capacity is 8
Explanation of the code
The above codes illustrates the working of size() and capacity() function. push_back() function is used to initilize the vector i.e. i is pushed onto the vector as the loop goes from 1 to 5 and terminates when i=6. so the elements in the vectors are 1,2,3,4,5. Then the size() function is used to print the size of the vector which comes out to be 5 and the capacity is 8 in this case.
size() vs empty()
empty() function is used to check whether the vector is empty or not.It does not give any information about size of the vector. It only returns bool value which is true if the vector is empty or false otherwise.
Return Values
- returns bool value
- true: if the vector is empty.
- false: if the vector is not empty.
Example 1
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vect;
if (vect.empty())
{
cout << "True"<<endl;
}
else
{
cout << "False"<<endl;
}
return 0;
cout<<"size of the vector is "<<vect.size();
}
Output:
True
size of the vector is 0
Explanation of the code
The code above shows the difference between empty() and size() function.The vector vect does not contain any element i.e. the vector is empty. The empty() function in this case returns True because the vector does not contain any element, Whereas
the size() returns 0.
let's see what happens when the vector is not empty.
Here is another example that shows the results in that case:
Example 2
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vect;
vect.push_back(3);
vect.push_back(4);
vect.push_back(6);
vect.push_back(9);
if (vect.empty())
{
cout << "True"<<endl;
}
else
{
cout << "False"<<endl;
}
return 0;
cout<<"size of the vector is "<<vect.size();
}
Output:
False
size of the vector is 4
Explanation of the code
So, in this example we have initilized the vector with 4 elements.so the vector contains 3,4,6,9. As it is clear that the vector is not empty the empty() function will return false, whereas the size() will return the size of the vector which will be 4 in this example.
Application of empty()
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> vect;
int sum=0;
for (int i = 1; i < 6; i++)
{
vect.push_back(i);
}
while(!vect.empty())
{
sum += vect.back;
vect.pop_back();
}
cout<<sum;
return 0;
}
Explanation of the code
The above example illustrates the use of empty() function , the code sums the elements of the vector, it starts adding the elements from the last of the vector using the back() function. Each time an element is added to the sum that element is popped from the vector using the pop_back() function and it continues to do the sum until the vector is empty. Each time the condition is checked using empty() function, the function returns false until the vector is not empty. once the vector is empty the empty() function returns true and the condition becomes false.
QUESTIONS
If the vector container is empty what will size() and empty() returns?
With this article at OpenGenus, you must have a complete idea of Vector::size() in C++ STL to get the size of any vector and its comparison with other related functions. Enjoy.