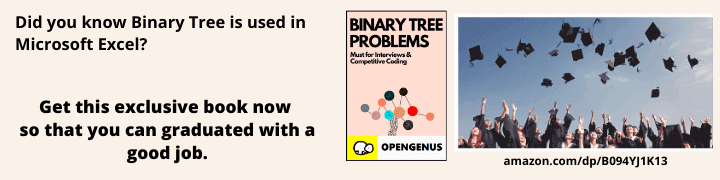
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
This article at OpenGenus list the key topics and tools you must be aware of practically to be a strong Web Developer. This Web Dev Roadmap is the perfect starting point.
Types of web developer
Front end development refers to the creation of the visible parts of a website or application that users interact with, such as the layout, design, and functionality of buttons, menus, and forms.
Back end development involves the creation of the underlying code and infrastructure that powers the front end of a website or application, including the server-side logic, database management, and API integrations.
Full stack development combines both front end and back end development, allowing a developer to create a complete and functioning web application or website from start to finish.
basic tools
-
Basic laptop or computer. You do not need high end system for web development, unless you are rendering the heavy animations. A safe requirement would be 8gb ram, i3 11th gen. and rest of sepcs can vary. It would be preferable to expand your ram later on, as you would be working with lot of open tabs in your browser and that takes up a lot of memory.
-
Operating system
macOS, windows, linux use any operating system that suits your workspace. I would highly recommend using Linux or maxOS, these operating systems are well tuned for programmer and at one point you will have to use linux for server handling, administration usage etc. -
text editor / ide : There are many integrated code editors that helps you in writing in easier and faster codes. vsocde is the most popular among them and it is also open source.
Visual Studio code (vscode)
vim
atom -
web browser
Chrome and Firefox is the most commonly used for web development as they provide users with best build tools. Firefox is my recommendation. -
Terminal (bash): You should learn how to use linux terminal as it will later become part of your coding jouney to be used in daily task. It is a Text interface that let's you give commands to the operating system's brain Kernal.
HTML & CSS
These are the basic building blocks of a website. HTML serves as the documentation part of the web, displaying raw data such as text, images, and videos. CSS, on the other hand, is responsible for designing and presenting that data, making the website more presentable and visually appealing. Learning these two languages is essential to get started, and later on, you can dive into more advanced topics.
- html layout, tags and attributes (doctype, head, body, p, img)
- semantic elements (header, footer, section)
- IDs vs classes
- CSS fundamentals
- CSS specificity
- flexbox & css grid
- responsivie layouts
Javascript
JavaScript is an all-in-one language/tool that is essential for creating a full-fledged website. It adds dynamic and interactive features on top of HTML and CSS, allowing you to manipulate styles and make your website more engaging. With JavaScript, you can add or remove HTML tags and take full control of your website from one place. Additionally, it can work with APIs to fetch data from the web, making your website more versatile.
JavaScript also offers several frameworks designed specifically for web development. For front-end development, you can use React and Angular, while for backend development, you can use Node.js.
- learn the basics
- The browser environment
- document object model (DOM)
- ES6+ features (arrow functions desturcturing, template literals)
- array methods
- asynchronous JS & https (callbacks, promises, fetch, JSON)
CSS preprocessors
These are used to make your stylesheet more maintanable. It adds up to the functionality of CSS and makeing it easier to write codes. you can create varibles, functions to reuse the code in css. You can group the parent and child (nesting) class in one block. By writing less code and having a better understanding of class hierarchy, you can make your stylesheets more efficient and powerful
- SASS
- POST CSS
CSS framework
These CSS framework are pre-build designs and component that you can use to get started quick and you can change the styles according to your requirements. I would prefer using TailwindCSS it is everything like css but less codes and it written inside of class function of HTML tags so you do not have come up with class name or search which class is where.
It gives you freedom to work to the very scratch like css.
- TailwindCSS
- Bbootstrap
UI design priciples
It would help you work with designs better. As designs come from different people and in order to code you have understand the basic principle of those desings.
- Color & contrast
- Whitespace
- Scale
- Visual hierarchuy
- Typography
design software
- Figma
- Adobe xd
tools and utilities
These tools will help you improve your work and time. It helps you in code backup, how to work in a group, debugging the code etc.
- Git and Github
- Browser developer tools
- npm (node package manager)
- Markdown Lightweight markup language
- editor / ide extensions
- https clients ( axios, postman, curl)
AI
Taking help of AI in forming a basic idea of a project or solving you doubt can be of great help.
- github copilot
- chatgpt
Front end deployment
Making a website will require you to host it somewhere to show others and make it avaible worldwide. These tools will help you in that.
- domain names
- hosting
- netlify
- vercel
- github pages
Build tools
These build tools helps you in automating the same task such as compiling, debugging and most importantly deploying the code.
- webpack
- parcel
- vite
- showpack
Front end frameworks
React is the most widely used JS framwork and most industry adapted. It has the concept of Hooks that deals with different aspects of the website, like state, re-render, response etc.
- React (context api "Gatsby") , material ui
- vue
- angular
- svelte
- solidjs
Typescript
It is a superset of Javascript, which means it is built on top of js with added functionality like static typing and class based object oriented object progamming. Varibles can be defined with a specific type such as string or number and the type will be forced by the TS which helps in catching error early on.
testing
Part of writing good code is testing it against various scenarios which makes you code less breakable. It is a necessary part of being a good developer.
- Unit testing
- Integration testing
- End to End testing
- Performance testing
languague specific testing library
- Javascript
- Jest
- Mocha
- Python
- robot
- pytest
- doctest
- Java
- selenium
- JUnit
WEB APIs
- canvas api (dynamic graphics and visualization)
- geolocation (get user's loation)
- speech API (speech recognition and synthesis)
- Audio and video api
- web RTC (realtime communication with audio/video
- local storage (store data in broser)
Full stack frameworks (ssr)
These are framworks built on top of JS libraries to improve certain parts of the code. Next.js which is built with React solves Routing, rendering etc.
- nextjs uses react
- remix uses react
- nustjs uses vue
- universal uses angular
- redwood ( build on top of nextjs)
- blitz (nextjs toolkit, authentication)
static site generators (SSG)
- Astro: Generates static html with little to no js, loads components on server first
- gatsby: uses react components, has graphQl data layer, image optimization, code splitting
- gridsome: uses vue
headless CMS
Admin area to create content without being bound to a specific front-end,
output JSON, so that you can just fetch the data and use a custom fron-end / UI,
great solution for clients that want to be able to update and add their own content
- Strapi (build on nodejs)
- sanity io
- keystone (powerful content management)
- contentful (cloud based cms)
The Jamstack
Architectural approach to building websites with javascript, Api's and markup,
emphasizes the use of SSG, SSR, headless CMs, serverless functions,
jamstack websites are decoupled and extremely fast, secure and easily maintained
ALpines.js
Alpine is technically a framework, but works more like a lirbrary. it actually labels itself modern jQuery.
- Data binding
- event handling
- Hide / Show elements
- Data synchronization
- conditionals & loops
Visualizations
- Motion UIL: sass library for animation)
- Framer: animations in react, support of 3d animations)
- Three.js: very popular, js API for 2d/3d graphics)
- Gsap: high performance timeline based animations)
no code tools
No code tools helps beginners and small startups to get started quickly and work with the application on their own, as it does not requires coding knowledge.
- wordpress
- webflow
- shopify
- wix
server-side language
MVC (modern view controller) it is common design pattern that these framework use it separates applications concerns into three components.
model- provides data and associated logic to the view,
view - renders the model to the view
controller - interacts with model and view
- node js
- express
- fastify
- nestJS
- Adonis
- python
- Django
- flask
- fast api
- php
- laravel
- sumfony
- codeigniter
- slimPHP
- java
- Spring
- Struts
- blade
- C#
- ASP.NET MVC
- Golang
- gin
- beego
- echo
- ruby
- Rails
- Sinatra
- kotlin
- Spring
- javelin
- rust
- rocket
- scala
- R
- swift
DATABASE
Data management is an essential aspect of modern life. In today’s world, data is considered to be one of the fundamental building blocks of existence. It can be found everywhere on the web, and everything around us is made up of some sort of data. The internet, in particular, is a platform for the sharing of data.
So, it is natural to have different ways to handle that data. Here are listed few ways/types of data.
_
Relational databases
As the name suggests, it forms a relation between all sorts of gathered data with a common key code.
- traditional table-based structure
- data stored in cloumns and rows
- usually use SQL
- been around since the 70s
- great for related data (social networks, etc)
- vergy rigid in terms of structure
- good with complex queries
type of SQL databases.
Postgress SQL
MySQL
Oracle
MS SQL
NoSQL database
Unline traditional SQL databases it is different as it does not work with structured query or tables. Instead they use various models such as document, key-value, column family, or graph, to store and retrieve data.
- many types (dociment, columns-based, key/value)
- data stored in JSON-like docuemnts
- Newer type of database
- veru flexible structure
- used in big data applications
- great scalability
- not as well suited for complez queries
Type of NOSQL database
MongoDB
Cassandra
Couchbase
DynamoDB
serverless/cloud databases
- MongoDB Atlas
- Firebase
- Supabase
- FaunaDB
file-based databases
- sqlite
- .md files (markdown file)
- h2 db
- redis (caching) explore
ORMS (Object realtional mapper)
ORMS provides a way to work and interact with data from within your code without writing raw SQL queries.
It simplifies database interaction, increases productivity and readability and allows the appllication to be independent of the database
- Prisma type-sage database client, support many database
- mongoose (orm for node.js and MongoDB, create models and schemas)
- Sequelize (orm for nodejs and mysql)
- sqlalchemy (orm for python, support mysql, postgres, sqlite and oracle)
BAckend API
-
REST API
- representational state transfer
- traditional client-server model
- much more common
- uses specific https methods and endpoints
- fetches all or nothing
-
GraphQL
- query language and runtime for APIs
- allows client to specify the data they want
- improved performance
- One /graphql endpoints
- use a client such as apollo
authentication & authorization
- sessions & cookies
- JSON web tokens
- OAuth
- third party services
- other related topics
- password hashing
- two-factor auth
- protecting routes
Devops skills
It is a skills that refers to the developers working in a team to collaborate, build, test and deploy applications faster and more efficient.
- Terminal/linus commands
- web servers
nginx, apache configuration - containerization and virtualization
- assets and file strorage (cloudinary, amazon s3)
- CI/CD
- jenkins, travis, CI, cirlceCI
- Infrastructure as code
- terraform, cloudformation
WEB3
- Blockchain
- dApps
- smart contracts
- cryptocurrency
- identity solutions
With this article at OpenGenus, you are all set to become a top Web Developer.