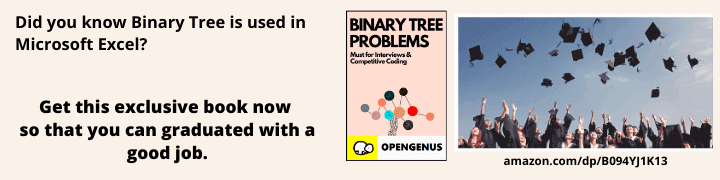
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained step by step How to Create Google Chrome Extension along with complete code.
Table of contents
- What is a browser extension?
- Technologies used
- Components of a google chrome extension
- HelloWorld extension
- Running your extensions
- Conclusion
What is a browser extension?
It is a program that enhances the functionality of the browser like password managers, porn blockers. Despite the stock features that come shipped with the browsers, they may not suffice to accomplish user's goals.
And by tapping into the Browser API, developers can develop extensions tailored to the user's needs.
Technologies used
- HTML
- CSS
- JavaScript
Components of a Google Chrome Extension
-
Input Components
- These are the components that are closest to the user. This is what the user interacts with. They make up the User Interface (UI) of the extension. Forexampe:
- BrowserAction
- PageAction
- Omnibox Input
- Shortcut key
- Context Menu
- Shortcut key
- These are the components that are closest to the user. This is what the user interacts with. They make up the User Interface (UI) of the extension. Forexampe:
-
Scripting Components
- As you guessed right, they provide the application logic (behavior) of the extension, listen for events i.e what happens when the user clicks this button, presses this key.
-
Popup Scripts
If your mind drove you straight to pop ads, you are not alone. I would like you to picture a popup as a UI element floating in the air. And any events fired on button clicks, mouse movements in that popup, they are handled by the popup script. Event scripts can also listen for events from popups. -
Event Scripts (background scripts)
They are analogous to the popup scripts, though for these, their lifetime is long lived, they run in the background. They represent the extension runtime better than popup scripts. -
Content Scripts
They are injected into visited pages and this makes them a right candidate when you need access to the DOM (Document Object Model). They can access the Standard Javascirpt API just like regular web page scripts do. They have limited access to the Chrome Extension API, they can only access chrome.runtime, chrome.extensions, chrome.storage.
-
- As you guessed right, they provide the application logic (behavior) of the extension, listen for events i.e what happens when the user clicks this button, presses this key.
-
Manifest Component
- This is the core component of a google chrome extension. It is a JSON formatted file that provides information about extension's metadata, permissions, features used by the extension i.e PageAction, BrowserAction.
{
"manifest_version": "2",
"name": "Hello World",
"version": "1.0.0"
}
The minimum set of attributes required for a google chrome extension.
HelloWorld extension
Directory heirachy
manifest.json
{
"manifest_version": 2,
"name": "HelloWorld Extension",
"version": "1.0.0",
"browser_action": {
"default_title": "HelloWorld",
"default_icon": "icon.png",
"default_popup": "popup.html"
}
}
Note: I prefer to use semantic versioning i.e 1.0.0 than to use some vague incremented numbers like 1, 2, 3.
Lets now move on to the popup.html file.
<!doctype html>
<html>
</head>
<link rel="stylesheet" href="styles.css">
<script src="popup.js"></script>
</head>
<body>
<div class="container">
<p>Hello World</p>
</div>
</body>
</html>
As you see, it is a simple html file. But you can make it as complex as your needs might demand.
Note: Popups do not allow Inline scripts. All paths used are relative to the extension root folder.
<script>
// Inline scripts are not allowed
console.log("Hello World!");
</script>
After laying out our HTML, I think its time we put on our designer hats and paint something on the canvas.
body {
/* height and width of the popup */
width: 200px;
height: 300px;
background-color: #112B3C;
color: gray;
font-size: 20px;
}
.container {
width: 100%;
height: 100%;
/* Centering the paragrah vertically and horizontally */
display: flex;
justify-content: center;
align-items: center;
}
And here comes the popup.js
console.log("Hello from HelloWorld extension");
/* Listen for events, perform any application logic */
Note: A popup script is only executed when a browserAction or pageAction has been clicked, and this is the reason why it is not a perfect candidate for representing the runtime of the extension.
Running your extensions
-
Fire up Google Chrome and navigate to chrome://extensions
-
Turn on developer mode switch.
-
Click on Load unpacked
-
Select your root folder housing your extension files. In our case that will be HelloWorld.
-
Click on your extension and see the magic...
Note: There are are errors reported because I used a manifest_version of 2 which will be deprecated in 2023 but no worries, manifest_version 3 is not rocket science.
Conclusion
When I was learning to write software, I underestimated the importance of fundermentals (basics) and it cost me alot. However much, this article used HelloWorld extension for illustration, It provides a foundation on which more complex extensions can be built because
Success is all about consistency around the fundermentals.
By Robin S. sharma