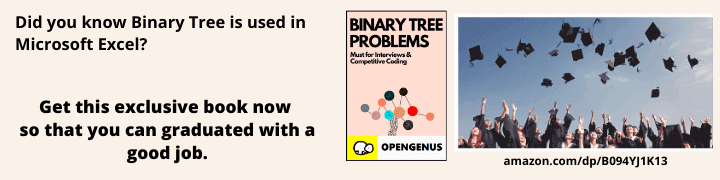
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained Abstraction in C++ in depth with different types of abstraction and code examples.
Table of contents:
- Abstraction
- Data abstraction with real life Example
- Advantages of Abstraction
- Types of Abstraction
- Example code of abstraction in C++
- Implementation Technique
Abstraction
Abstraction means hiding the background details or functioning and displaying only important information. We have explained abstraction in C++ in depth.
Data Abstraction
Data abstraction means it is a technique which depends on seperation of the interface and its implementation.It provides only important information to outside world about the data and hide the background details without presenting it to the user.
Example: Consider the operation of TV
A TV can be turned ON/OFF and its channel or its volume can be adjusted by external devices and can be connected to other external devices also. But how the signal is generating/ translating and how the screen is functioning, the mechanism behind it is unknown to the user.
Therefore, TV seperates external interface and internal implementation and still user can use it without having zero background knoeledge of its internal functioning.
Access specifiers plays an important role in abstraction and we can say that access specifiers enforces abstraction.We can implement private and public labels in our program to hide the important information.Members defined public can be accessed by class from anywhere in the program and members defined private can be only accessed within the classes.Their usage is prohibited from outside class.
Advantages of Abstraction
- It avoids duplication of code and increases its reusability.
- It protects class internals from unintended user level errors, which might can corrupt the object state.
- It gives security to the application as only needed information is showed to the user and essentials are hiden from it.
- Class implementation can be changed when there are some bug errors in the program without affecting its internal implementation.
Types of Abstraction
Abstraction can be achieved by two methods:
1.Abstraction using classes: In c++, we use access specifiers to decide which data member or function will be visible to the outside world.These access specifiers helps to group data members and functions where its accessibility can be further decided.
2.Abstraction in header files: We have header files like iostream and math.h defined in C++. We simply use cout without knowing the algorithm that how it is displaying the text on screen.In math.h library we have pow() declared, when we need to calculate the power of any number we just give arguments to it and we get our desired results, we dont know how it is calculating power internally.
Example code of abstraction in C++
1. Using classes:
#include <iostream>
using namespace std;
class Subtraction
{
private: int a, b, c; // private variables
public:
void sub()
{
cout<<"Enter two numbers: ";
cin>>a>>b;
c= a-b;
cout<<"Difference of two number is: "<<c<<endl;
}
};
int main()
{
Subtraction sb;
sb.sub();
return 0;
}
Output:
2. Using header files:
#include <iostream>
#include<math.h>
using namespace std;
int main()
{
int n = 7;
int power = 2;
int answer = pow(n,power); //pow is the in built function defined in math.h library
cout << "Square of n is : " <<answer<<endl;
return 0;
}
Output
Implementation Technique:
Abstraction detach code implementation and its interface.So, when we are designing our code we should keep implementation and interfaces independent. So that, if a user is trying to change some of its underlying implementation then interface mechanism should remain same.Then the code will only be needing a recompilation with latest information.