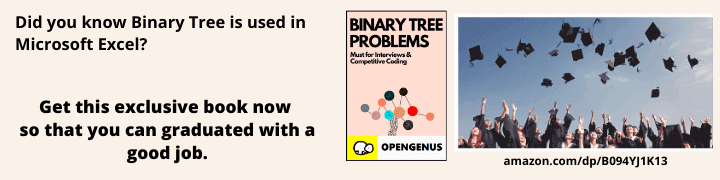
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the approach to find the ASCII value of a character in various Programming Languages along with the introduction to ASCII.
Table of contents:
- Introduction to ASCII
- Why is ASCII Important?
- Displaying Characters and their ASCII Values
- ASCII characters and their range of values
- Conclusion
Introduction to ASCII
ASCII Stands for American Standard Code for Information Interchange, it is a character encoding standard. ASCII is used to facilitate the exchange of information between different systems or devices. ASCII allows for the conversion of text and other symbols (control characters) into binary which is easier to move across devices. The first edition of ASCII was released in 1963, and most modern character encoding schemes are based on ASCII even though they may support additional characters. Originally ASCII was used to represent the 128 english characters as numbers (binary or hexadecimal). The original ASCII code uses 7 binary digits, this gave it a capacity of encoding 128 different characters.
For example the binary digit 1010000 represents an upper case P while the code 1110000 represents a lower case p.
Why is ASCII Important?
Computers and other electronic devices only understand binary and are only able to save data as a string of binary digits. These data does not usually come in binary format, for example a text file typed by a human user. In order to save such data it has to be converted in to a series of binary numbers. Due to the fact that information has to be transferred from one device to the other, it is important that a file saved by one device can be properly understood by other devices. ASCII was introduced as a standard for computers to follow when saving data in a way that allows for easy transfer of such data to different devices without any confusion or corruption of data.
Displaying Characters and their ASCII Values
let char = prompt("please enter a character")
let value = string.charCodeAt(0)
console.log(value)
The code sample above is a simple javascript program that can be used to display the ascii value of a character. The first line displays a prompt which asks the user to enter a character. The entered character is save in a variable called char. On line two the charCodeAt javascript function is used to get the ascii (decimal) value of the character. Finally on line three the ascii value is displayed in the browser console.
#include<iostream>
using namespace std;
int main ()
{
char c;
cout << "Enter a character : ";
cin >> c;
cout << "ASCII value of " << c <<" is : " << (int)c;
return 0;
}
The code snippet above is a C++ program which accepts a character from the user and also prints its ascii value back to the screen. The decimal (integer) value of the character is printed just like in the previous code snippet.
ch = input("Enter any character: ")
print("The ASCII value of char " + ch + " is: ",ord(ch))
This last code snippet is a python program which asks the user to enter a character and then proceeds to print out the ascii value of the character to the screen. The ascii value is gotten by using the python ord function.
ASCII characters and their range of values
The table below shows the various characters and their corresponding ASCII values.
Character(s) | ASCII VALUE/Range | Description |
---|---|---|
0-31 | Control characters | |
! | 32 | Space |
! | 33 | Exclamation mark |
" | 34 | Quotation mark |
# | 35 | Hash symbol |
$ | 36 | Dollar sign |
% | 37 | Percent sign |
& | 38 | ampersand |
' | 39 | apostrophe |
( | 40 | Left parenthesis |
) | 41 | Right parenthesis |
* | 42 | asterisk |
+ | 43 | plus sign |
, | 44 | comma |
- | 45 | hyphen |
. | 46 | period |
/ | 47 | forward slash |
0-9 | 48-57 | numbers |
: | 58 | colon |
; | 59 | semi colon |
< | 60 | less than |
= | 61 | equals to |
> | 62 | greater than |
? | 63 | question mark |
@ | 64 | at sign |
A-Z | 65-90 | upper case alphabets |
[ |
91 | left square bracket |
\ | 92 | backslash |
] | 93 | right square bracket |
^ | 94 | caret |
_ | 95 | underscore |
' | 96 | grave accent |
a-z | 97-122 | lower case alphabets |
{ | 123 | left curly bracket |
124 | ||
} | 125 | right curly bracket |
~ | 126 | tilde |
Conclusion
The American Standard Code for Information Interchange has been of great importance in the field of electronics. It provided a way for devices to exchange data seamlessly between each other. There have been newer standards such as utf-8 which encode a larger set of characters but they are mostly based on the same concept as ASCII.
With this article at OpenGenus, you must have the complete idea of ASCII value of a character.