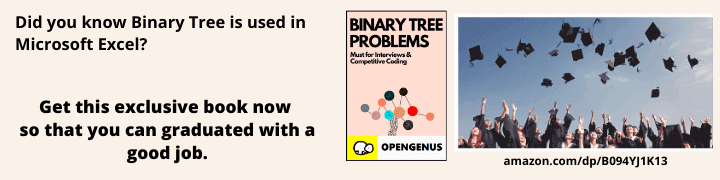
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
An unordered multiset is an unordered set (a set in which a key can be stored in any random order) that allows different elements to have equivalent values (allows duplicate items).
How are elements of an Unordered multiset stored internally?
1. The elements of the Unordered multiset are organized into buckets.
2. It uses hashing to insert these elements into buckets.
3. This reduces the access time of an individual element because with the help of hash values we can directly access the bucket which contains the element.
4. It contains a count variable that counts the total no of occurrences of that particular element in the Unordered multiset.
5. As it needs extra space to store count variable in Unordered multiset, it takes more space than unordered_set (if all values are distinct).
Syntax of Unordered multiset
unordered_multiset <data_type> var_name;
- where
data_type
is the type of data being stored in the Unordered multiset. var_name
is the name of the Unordered multiset.
for ex: unordered_multiset <int> view_set;
where int
is the data type of Unordered multiset and view_set
is the name of Unordered multiset.
Note: To work with Unordered multiset we need to include unordered_set library (#include<unordered_set>).
Basic methods of Unordered multiset
- insert() is a function in c++ STL that inserts new elements into the Unordered multiset.
syntax of insert function:
Unordered_multiset_name.insert(value);
- where
Unordered_multiset_name
is the name of the Unordered multiset. value
is the element which is to be inserted.
C++ program implementing insert function
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
view_set.insert(5);
cout << "Elements present in view_set are :";
for (const int& x : view_set)
cout << " " << x;
cout << endl;
return 0;
}
Output
Elements present in view_set are : 11 8 7 5 5 3
2.Bucket_count() is a function in C++ STL that returns the total number of buckets present in the unordered_multiset.
syntax of bucket_count function:
Unordered_multiset_name.bucket_count();
- where
Unordered_multiset_name
is the name of the Unordered. multiset. - It does not accept any parameter.
c++ program implementing bucket_count function
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
view_set.insert(5);
auto n = view_set.bucket_count();
cout<<"view_set has "<< n<< " buckets.\n";
//prints the elements present in each bucket.
for (auto i=0; i<n; ++i) {
cout<< "bucket "<< i << " contains:";
for (auto it = view_set.begin(i); it!=view_set.end(i); ++it)
cout << " " << *it;
cout << "\n";
}
return 0;
}
Output
view_set has 7 buckets.
bucket 0 contains: 7
bucket 1 contains: 8
bucket 2 contains:
bucket 3 contains: 3
bucket 4 contains: 11
bucket 5 contains: 5 5
bucket 6 contains:
3.Begin() is a function in C++ STL that returns an iterator pointing towards the first element of the Unordered multiset or to the first element in one of its buckets.
syntax of begin function:
Unordered_multiset_name.begin(n);
- where
Unordered_multiset_name
is the name of the Unordered multiset. - where
n
is the bucket no which should be less than bucket count. - If a parameter is not passed, then it returns an iterator pointing towards the first element in the unordered_multiset.
- If a parameter is passed, it returns an iterator pointing to the first element in the bucket.
c++ program implimenting begin function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
view_set.insert(5);
cout << "First element in the Unordered multiset :";
cout<<*view_set.begin();
return 0;
}
Output
First element in the Unordered multiset :11
4.End() is a function in C++ STL that returns an iterator pointing towards the location immediately after the last element in the Unordered multiset or to the location immediately after the last element in one of its bucket.
syntax of end function:
Unordered_multiset_name.end(n);
- where
Unordered_multiset_name
is the name of the Unordered multiset. - where
n
is the bucket no which should be less than bucket count. - If parameter is not passed, then it returns an iterator pointing to the location immediately after the last element in the unordered_multiset.
- If a parameter is passed, it returns an iterator pointing to the location immediately after the last element in the bucket.
c++ program implimenting end function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
view_set.insert(5);
// Using begin and end functions to traverse and prints all elements of the Unordered multiset
for (auto it = view_set.begin(); it != view_set.end(); it++)
cout << *it << " ";
return 0;
}
Output
Elements present in view_set are : 11 8 7 5 5 3
5.Count() is a function in C++ STL that returns the number of times a value occurs in the Unordered multiset.
syntax of count function:
Unordered_multiset_name.count(val);
- where
Unordered_multiset_name
is the name of the Unordered multiset. val
is the element in Unordered multiset whose total no of occurrence is to be returned.
c++ program implimenting count function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
view_set.insert(5);
view_set.insert(11);
view_set.insert(5);
cout<< "No of times 5 has been repeated in Unordered multiset is "<<view_set.count(5) <<"\n";
cout << "No of times 11 has been repeated in Unordered multiset is "<<view_set.count(11) <<"\n";
cout << "No of times 3 has been repeated in Unordered multiset is "<<view_set.count(3) <<"\n";
return 0;
}
Output
No of times 5 has been repeated in Unordered multiset is 3
No of times 11 has been repeated in Unordered multiset is 2
No of times 3 has been repeated in Unordered multiset is 1
6.Empty() is a built-in function that returns a boolean value. If the Unordered multiset is empty then it returns true.
syntax of empty function:
Unordered_multiset_name.empty();
- where
Unordered_multiset_name
is the name of the Unordered multiset. - It does not accept any parameter.
c++ program implimenting empty function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
//let us take 2 Unordered multiset one of which is not empty and the other one is empty
unordered_multiset <int>view_set;//not empty unordered multiset
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
unordered_multiset <int>empty_set;//empty unordered multiset;
if(view_set.empty()){
cout<<"view_set is empty\n";
}else{
cout<<"view_set is not empty\n";
}
if(empty_set.empty()){
cout<<"empty_set is empty\n";
}else{
cout<<"empty_set is not empty\n";
}
return 0;
}
Output
view_set is not empty
empty_set is empty
7.Clear() is a function in C++ STL that removes all elements of the unordered_multiset.
syntax of clear function:
Unordered_multiset_name.clear();
- where
Unordered_multiset_name
is the name of the Unordered multiset. - It does not accept any parameter.
c++ program implimenting clear function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
if(view_set.empty()){
cout<<"view_set is empty\n";
}else{
cout<<"view_set is not empty\n";
}
view_set.clear();//clears all elements from view_set
if(view_set.empty()){
cout<<"view_set is empty\n";
}else{
cout<<"view_set is not empty\n";
}
return 0;
}
Output
view_set is not empty
view_set is empty
8.size() is a function in C++ STL that counts total no of elements in the Unordered multiset.
syntax of size function:
Unordered_multiset_name.size();
- where
Unordered_multiset_name
is the name of the Unordered multiset.
c++ program implimenting size function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
cout<<"Unordered multiset contains "<<view_set.size()<<" elements";
return 0;
}
Output
Unordered multiset contains 5 elements
9.find() is a function in C++ STL that returns an iterator that points towards the location which contains the element val. If the element is not present in the Unordered multiset, then it returns an iterator that points to the location after the last element in the Unordered multiset.
syntax of find function:
Unordered_multiset_name.find(val);
- where
Unordered_multiset_name
is the name of the Unordered multiset. val
is the element in Unordered multiset whose location is returned.
c++ program implimenting find function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
auto it = view_set.find(5);
if (it != view_set.end())
cout << *it <<" is found"<<endl;
else
cout << "5 not found\n";
it = view_set.find(12);
if (it != view_set.end())
cout << *it << endl;
else
cout << "12 not found\n";
return 0;
}
Output
5 is found
12 not found
10.Equal_range() is a function in C++ STL that returns the range in which all the elements are equal to the given value.
- It returns a pair of iterators where the first is an iterator pointing towards the first element of the range and the second is an iterator pointing towards the last element of the range.
- If the given element is not present in the Unordered multiset it returns a pair where both lower and upper bound points towards the location after the end of the container.
Syntax of equal_range function.
Unordered_multiset_name.equal_range(val);
- where
Unordered_multiset_name
is the name of the Unordered multiset. val
is the element in Unordered multiset whose range is returned.
C++ program implimenting equal_range function.
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_multiset <int>view_set;
view_set.insert(5);
view_set.insert(3);
view_set.insert(7);
view_set.insert(8);
view_set.insert(11);
view_set.insert(5);
view_set.insert(11);
view_set.insert(5);
auto itr = view_set.equal_range(5);//pair of iterators pointing to range of 5
for (auto it = itr.first; it != itr.second; it++) {
cout << *it << " ";
}
cout << endl;
itr = view_set.equal_range(11); //pair of iterators pointing to range of 11.
for (auto it = itr.first; it != itr.second; it++) {
cout << *it << " ";
}
return 0;
}
Output
5 5 5
11 11