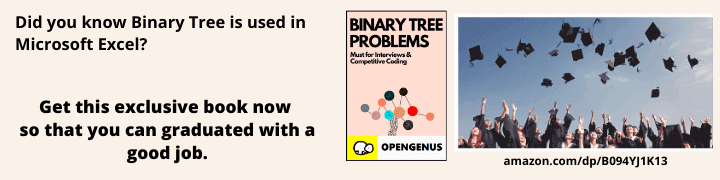
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Generally, we just use a right click to create or delete a folder in our windows OS! But there are other ways also to do the same task. One of the very common way is to use command prompt (i.e., cmd).
Creating Folder
So first we will see how we create a folder using cmd. For this open a command prompt, navigate to the folder where you want to create a new folder using cd. Then use command mkdir followed by the name of the folder you want to create. After that you can simply use command dir to check if the folder has been created.
Now we will see how to create a folder using C++:
To accomplish this task we are going to use mkdir() function. mkdir() function creates an empty folder with the specified path name.
Function format: int mkdir(const char* pathname)
mkdir() function works little bit differently on POSIX(Linux, Unix, etc). It takes two parameter where first parameter is pathname and second is mode.
int mkdir(const char *pathname, mode_t mode);
Here mode is a bitwise-OR field that specifies what permissions (read,write or both) the directory has when it is created.
Also while working in Unix we have to include <unistd.h> instead of <direct.h>
Following program shows how to create folder in C++:
#include<iosrtream>
#include<direct.h>
using namespace std;
int main(){
//Creating File
if(mkdir("E:/MyFolder") == -1)
cerr << " Error : " << strerror(errno) << endl;
else
cout << "File Created";
}
Explanation for above code:
iostream - It provides basic input and output services for C++ programs.
<direct.h> - <direct.h> is a C/C++ header file provided by Microsoft Windows OS which contains functions for manipulating files/folders. mkdir() is one of the functions under this file.
mkdir() - It takes path of the folder as argument and creates a folder.
Return Type of mkdir(): After successfully creating a folder mkdir() returns a 0. Otherwise it returns -1, no folder is created and error is disppayed.
After successully creating a folder it displays following output:
In above code we can see "errno" which displays error message when one of the following errors occur.
1) [EACCES] -This error occurs a) If parent directory in which we are creating new file does not allow "write" permission. b) If one of the directories in the path name does not allow search permission.
2) [EEXIST] - This error is diplayed if file which we are trying to create with specified name is already present.
3) [ELOOP] - This type of error occurs if number of symbolic links in the pathname specified is more than POSIX_SYMLOOP (This is specified in limit.h header file). Symbolic Link: It is not an actual file, it is an entry to a file system that points to the file
4) [EMLINK] - If the link count of the directory in which we are creating new file exceeds LINK_MAX then EMLINK error occurs. The value of LINK_MAX can be determined using the pathconf() or the fpathconf() function. Link cout: It is the number of hard links to that file, it is always atleast two and increases with each subfile in the particular file.
5) [ENAMETOOLONG] - If the pathname (argument specified inside the mkdir() function) is longer than is longer than PATH_MAX characters or if some component of the name is longer than NAME_MAX characters then ENAMETOOLONG error occurs. The values of PATH_MAX and NAME_MAX values can be determined using pathconf() function.
6) [ENOENT] - This error occurs ifthe pathname specified inside mkdir() function or one of the components in path name does not exist.
These are some of the situations where mkdir() fails to create a folder and returns -1.
One of the situations where mkdir fails( [EEXIST] - The named file exist)
Deleting Folder:
So first we will see how we delete a folder using cmd. For this open a command prompt, navigate to the folder where the folder you want to delete is located using cd. Then use command rmdir followed by the name of the folder you want to delete. After that you can simply use command dir to check if the folder has been deleted.
Now we will see how to delete a folder using C++:
To accomplish this task we are going to use rmdir() function. rmdir() function deletes a folder with the specified path name.
Function format : int rmdir(const char *path);
Following program shows how to delete a folder using C++:
#include<iosrtream>
#include<direct.h>
using namespace std;
int main(){
//Creating File
if(mkdir("E:/MyFolder") == -1)
cerr << " Error : " << strerror(errno) << endl;
else
cout << "File Created";
}
Explanation for above code:
iostream and <direct.h> has already been explained above.
rmdir() - It take path of the folder as argument and delets the specified folder.
Return Type of rmdir() : After successfully deleting a folder rmdir() returns 0. Otherwise it returns -1, folder is not deleted and error is displayed.
After successfully deleting a folder it displays following output:
In above code we can see "errno" which displays error message when one of the following errors occur.
1) [EACCES] - This error occur a) if the directory in the pathname (argument specifies in rmdir() function) does not allow search permission or b) if one of the directory in the pathname does not allow search permission.
2) [EBUSY] - If the file we are trying to delete is in the process or used by some other process which is running at the same time then the EBUSY error occurs.
3) [ENOTEMPTY] - This error occurs if we are trying to delete a file which is not empty (we can only delete a file which is empty i.e. it should not contain any other objects) or if there are hard links to the directory other than dot or a single entry in dot-dot.
5) [EINVAL] - This error occurs if the arguments passed in rmdir function are incorrect or if the operations specified the particular object is not supported by the object.
6) [EIO] - A physical I/O error has occurred or if a referenced object was damaged.
7) [ENAMETOOLONG] - If the pathname (argument specified inside the rmdir() function) is longer than is longer than PATH_MAX characters or if some component of the name is longer than NAME_MAX characters then ENAMETOOLONG error occurs. The values of PATH_MAX and NAME_MAX values can be determined using pathconf() function.
8) [ENOENT] - This error occurs ifthe pathname specified inside rmdir() function or one of the components in path name does not exist.
9) [ENOTDIR] - If the pathname specified inside a rmdir() function exist but it is not a directory when it is expected to be a directory.
These are some of the situations where rmdir() fails to delete the folder and returns -1.
example:
So today we have seen how to use mkdir() and rmdir() functions to create and delete folder in C++.
NOTE : Above codes will not run on online IDE, you have to run them on your local IDEs.