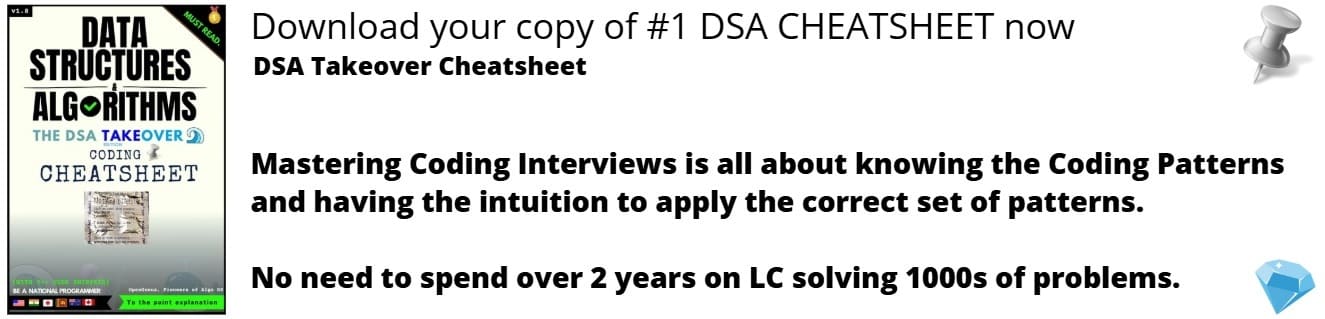
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we'll be discussing on MongoDB CRUD operations with Python. Initially we'll start with the introduction on the article. Secondly we'll discuss on the prerequisites for the readers to follow the further flow of the article. In later sections we'll discuss the main script that performs the CRUD Operation on MongoDB through Pythonic way using a Object Document Mapper(ODM
). We'll also implement an API using Flask Framework. At last we conclude with the output for same from Postman.
Following are the sections of this article:-
1. Introduction
In this article we'll be exploring the Python way of performing the CRUD operations on the MongoDB. Here we'll make use of the driver to communicate between the script/program which is written in Python Programming Language with the MongoDB. And the driver would be an Object Document Mapper(ODM
), PyMongo. We refer the documentation for PyMongo. Also we'll be making use of web-framework of python Flask
.
2. Prerequisites
Following will be a prerequisites to be able to follow the flow by the user,
A. A Python 3.5+ version must be installed at your machine
B. Mongo database needs to setup earlier.
C. A PyMongo & Flask to be installed usingpip
command.
D. Basic understanding on the NoSQL Database & API's.
3. Brief Background on CRUD
In this article we are aiming to learn on the CRUD operations of MongoDB through Pythonic way. We can go through the documentation of mongodb. The basic operations of any database are: Create, Read, Update, and Delete. Mongo’s format is as follows: Databases hold Collections, Collections hold Documents, and Documents hold attributes of data. Following are the relations of Create, Read, Update, and Delete between web-framework and the Database,
-
Create - If we want to CREATE a document in mongodb we need to use the method
collection.insert_one()
to insert a single document andcollection.insert_many()
to insert bulk documents. And if we want to perform this through API REQUEST, we need to use HTTP METHOD - POST REQUEST. -
Read - If we want to READ a document in mongodb we need to use the method
collection.find_one()
to get a single document andcollection.find()
to get bulk documents. And if we want to perform this through API REQUEST, we need to use HTTP METHOD - GET REQUEST. -
Update - If we want to UPDATE a document in mongodb we need to use the method
collection.update_one()
to update a single document andcollection.update_many()
to update bulk documents. And if we want to perform this through API REQUEST, we need to use HTTP METHOD - PUT REQUEST. -
Delete - If we want to DELETE a document in mongodb we need to use the method
collection.delete_one()
to update a single document andcollection.delete_many()
to update bulk documents. And if we want to perform this through API REQUEST, we need to use HTTP METHOD - DELETE REQUEST.
Above are the brief overview of the CRUD operations that can be performed on the MongoDB through API request using a WEB-FRAMEWORK of python. In the next section we are going to implement a ToDo CRUD API using mongodb and Flask as the web-framework.
4. CRUD Implementation
Below is the implemented script in python using PyMongo as driver for communicating with MongoDB as database through Flask app. Run this file after all prerequisites, python3 app.py
(Note: Here we have implemented a ToDo CRUD Implementation, and every time through the request body we are sending the database
, collection
name)
from flask import Flask, request, json, Response
from pymongo import MongoClient
# initialized the Flask APP
app = Flask(__name__)
class CrudAPI: # MongoDB Model for ToDo CRUD Implementation
def __init__(self, data): # Fetchs the MongoDB, by making use of Request Body
self.client = MongoClient("mongodb://localhost:27017/")
database = data['database']
collection = data['collection']
cursor = self.client[database]
self.collection = cursor[collection]
self.data = data
def insert_data(self, data): # Create - (1) explained in next section
new_document = data['Document']
response = self.collection.insert_one(new_document)
output = {'Status': 'Successfully Inserted',
'Document_ID': str(response.inserted_id)}
return output
def read(self): # Read - (2) explained in next section
documents = self.collection.find()
output = [{item: data[item] for item in data if item != '_id'} for data in documents]
return output
def update_data(self): # Update - (3) explained in next section
filter = self.data['Filter']
updated_data = {"$set": self.data['DataToBeUpdated']}
response = self.collection.update_one(filter, updated_data)
output = {'Status': 'Successfully Updated' if response.modified_count > 0 else "Nothing was updated."}
return output
def delete_data(self, data): # Delete - (4) explained in next section
filter = data['Filter']
response = self.collection.delete_one(filter)
output = {'Status': 'Successfully Deleted' if response.deleted_count > 0 else "Document not found."}
return output
# Achieving CRUD through API - '/crudapi'
@app.route('/crudapi', methods=['GET']) # Read MongoDB Document, through API and METHOD - GET
def read_data():
data = request.json
if data is None or data == {}:
return Response(response=json.dumps({"Error": "Please provide connection information"}),
status=400, mimetype='application/json')
read_obj = CrudAPI(data)
response = read_obj.read()
return Response(response=json.dumps(response), status=200,
mimetype='application/json')
@app.route('/crudapi', methods=['POST']) # Create MongoDB Document, through API and METHOD - POST
def create():
data = request.json
if data is None or data == {} or 'Document' not in data:
return Response(response=json.dumps({"Error": "Please provide connection information"}),
status=400, mimetype='application/json')
create_obj = CrudAPI(data)
response = create_obj.insert_data(data)
return Response(response=json.dumps(response), status=200,
mimetype='application/json')
@app.route('/crudapi', methods=['PUT']) # Update MongoDB Document, through API and METHOD - PUT
def update():
data = request.json
if data is None or data == {} or 'Filter' not in data:
return Response(response=json.dumps({"Error": "Please provide connection information"}),
status=400, mimetype='application/json')
update_obj = CrudAPI(data)
response = update_obj.update_data()
return Response(response=json.dumps(response), status=200,
mimetype='application/json')
@app.route('/crudapi', methods=['DELETE']) # Delete MongoDB Document, through API and METHOD - DELETE
def delete():
data = request.json
if data is None or data == {} or 'Filter' not in data:
return Response(response=json.dumps({"Error": "Please provide connection information"}),
status=400, mimetype='application/json')
delete_obj = CrudAPI(data)
response = delete_obj.delete_data(data)
return Response(response=json.dumps(response), status=200,
mimetype='application/json')
if __name__ == '__main__':
app.run(debug=True, port=5000)
5. CRUD Operation break down
Following are the section wise description of the above implementation,
I. In the above file app.py
initially at the top of the file we are importing all the necessary module for our task like - Flask
& pymongo
are major modules.
II. We have written a line, app = Flask(__name__)
. This'll initialize a Flask web application instance to the app
variable.
III. Next, we have defined a model as a class CrudAPI
, through which we communicate to the MongoDB to perform CRUD on the same.
IV. We have a single end point for our Flask API as /crudapi, through which we perform - Create Read Update and Delete from the mongo database.
IV. Now lets explore more on the above class CrudAPI
.
a. An init() method has been written to establish the connection to the mongo database, when ever any other method of the class CrudAPI
has been called through the API /crudapi
from a Flask application.
b. To achieve the CRUD operation on the Mongo Database from Python, we have defined other methods for the class CrudAPI they are - insert_data(), read(),update_data(), delete_data().
c. Let us consider each in relation with the Flask Application's routes-
(Note: After running the Flask Server- python3 app.py
which we are running at our localhost-127.0.0.1, we have started accessing the API http://127.0.0.1/crudapi)
1. insert_data() - This Class method used For Creating a new mongo document into the mongo database, when we call the Flask API /crudapi
by HTTP method - POST, which actually calls the insert_one() method of collection from module pymongo to create/insert a SINGLE document. Following in the output from Postman-
From above image, we have requested our /crudapi Flask API by passing the database
- ToDoDB & collection
- ToDos. Also we are sending the new document as a request as - Document
and provided respective key-value data for the same. And we get the response as Document_ID
that is created successfully.
2. read() -This Class method used To Read the documents that are available from the collection in a mongo database we call the Flask API /crudapi
by HTTP method - GET, which actually calls the find() method of collection from module pymongo that returns a pymongo cursor
which has all the documents, Hence we used a for loop to access each document. Following in the output from Postman-
From above image, we have requested our /crudapi Flask API by passing the database
- ToDoDB & collection
- ToDos to read the available Documents. And as a response we get all the Documents
with their respective fields details that are available.
3 update_data() - This Class method used for Updating a mongo document, when we call the Flask route API /crudapi
by HTTP method - PUT, which actually calls the update_one() method for collection from module pymongo to update a SINGLE document. Following in the output from Postman-
From above image, we have requested our /crudapi Flask API by passing the database
- ToDoDB & collection
- ToDos. Also we pass Filter
to represent the existing document to be replaced with the DataToBeUpdate
. As a response we get a status - Successfully Updated
from the API.
After update if we call the /crudapi
with GET request as in point 2, we get the following response-
4. delete_data() - This Class method For Deleting a mongo document, when we call the Flask API /crudapi
by HTTP method - DELETE, that calls the delete_one() method for collection from module pymongo to delete a SINGLE document. Following in the output from Postman-
From the above image, we have requested out /crudapi Flask API by passing the database
- ToDoDB, collection
- ToDos and ToDo_Name
to be deleted inside the Filter
. As A response we get the status - Successfully Deleted
from the API.
After delete if we call the /crudapi
with GET request as in point 2, we get the following response-
6. Conclusion
At last we are at the end of this article, So in this article we learned about a new module pymongo that actually acts a mapper between the Flask framework and the MongoDB. Later we saw the code implementation for the same and also we have break down the CRUD implementation with brief explanation.
Hope this was an informative article, Thanks!!