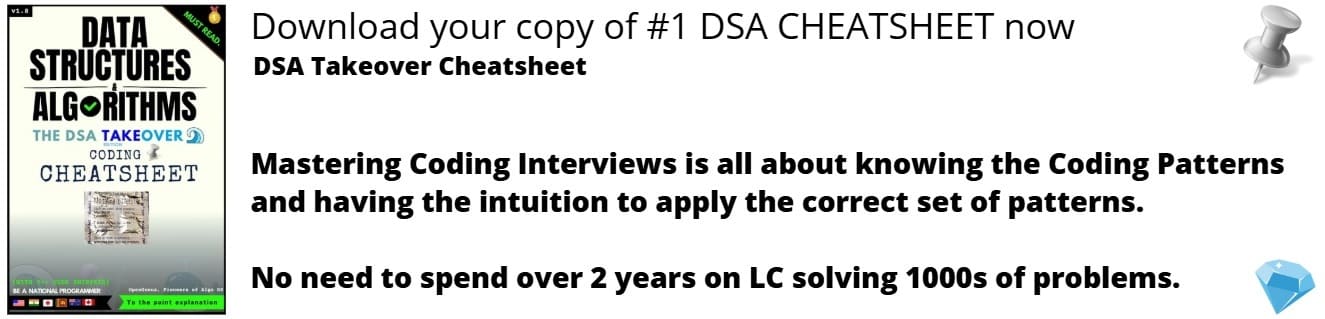
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored different ways to delete string in C++ which include clear, resize, erase, swap functions along with other techniques.
Table of content
- What is a string
- Ways to delete a string
- delete operator
- clear, resize, erase or swap functions
- out of scope
What is a string
Before delete something we must understand what it is. So, what is a string ?
Well, that depends on the compiler you are using !
If you use the C compiler a string might look like this:
char *s = "A simple text";
If you are using the C++ compiler a string declaration might look different:
char *s = "A simple text";
or
char *s = new char[ (int)sizeof("A simple text") ];
s = "A simple text";
or
string t ("A simple long text");
string t0;
string *t1 = new string();
string t2 =t;
string t3 (t);
string t4 (t, 9, 4);
string t5 ("A character sequence");
string t6 ("Another character sequence", 12);
string t7a (10, 'x');
string t7b (10, 42); // 42 is the ASCII code for '*'
string t8 (t.begin(), t.begin()+8);
In all cases a string is a declaration of a variable that stores a sequence of characters, characters that are defined in the ASCII table.
That is not the case of declaring a vector of elements of type char
char s[4] = {'A',' ','s','i','m','p','l','e',' ','t','e','x','t'};
Note that in C++ the statement
char *s = "A simple text";
will give you a warning message
ISO C++ forbids converting a string constant to ‘char*’
that can be fixed by adding const in front of the char
const char *s = "A simple text";
Lets now take a look at the s and t variables.
While the s variable is a pointer that stores the address of a character string, the t is an object of the class type string defined in the std namespace, meaning the t will have some member types and functions to work with.
Ways to delete a string
When we declare a pointer to a string we can deallocate memory by using the next statement:
char *s = "text";
s = NULL;
Things are more complicated when we work with string objects.
-
using delete operator
When you declare a simple instance of an object you can call delete operator
string *t = new string();
delete t;
When you declare a vector of elements and allocate memory for them with the new operator the statement for delete will be:
string *t = new string[3];
delete []t;
Note the brackets signs after delete operator.
The delete operator is applied to the object t that calls the destructor method of the class. The members are released in the reverse order of the declaration and you can use it only when an object has been created with the new operator !
Meaning, if you use this statement:
string t;
delete t;
results into a compiler error
type ‘std::string’ {aka ‘class std::__cxx11::basic_string’} argument given to ‘delete’, expected pointer
So, what else we can do ?
-
clear, resize, erase or swap functions
Fortunately, C++ language is an object oriented, that means it can be expandable.
We saw earlier that delete operator is appling only to a pointer with a memory allocated by the new operator.
How can we deallocate memory in case of a string object declaration ?
Since this is a class it comes with different methods of implementation.
clear
One of them is clear which erases the contents of the string, which becomes an empty string
(with a length of 0 characters)
Example:
string t ("A simple long text");
t.clear();
resize
The second one is resize with its overloaded declarations which resizes the string to a length of n characters, greater or lower than the initial one.
void resize (size_t n);
void resize (size_t n, char c);
Example:
string t ("A simple long text");
t.resize(0);
erase
The third one is erase which erases part of the string, reducing its length
string& erase (size_t pos = 0, size_t len = npos); //sequence (1)
iterator erase (iterator p); //character (2)
iterator erase (iterator first, iterator last); //range (3)
Example:
string t ("A simple long text");
t.erase();
Notice that erase is invoked without any argument even though there is no definition for it.
swap
The fourth one is using the swap function from the string class which exchanges the content of the container by the content of str, which is another string object. Lengths may differ.
void swap (string& str);
Since we want to empty the space of t without declaring another variable of string, the next statement will do that:
string t ("A simple long text");
string().swap(t);
There is another approch of swap, by calling it direclty from std namespace.
void swap (string& x, string& y);
But in this case we'll need to declare a second empty variable of string.
string s;
string t ("A simple long text");
swap(s,t);
-
out of scope
Depending on what is the purpose of a variable, its life depends on where it is declared.
Lets look at the next example:
#include <iostream>
using namespace std;
int main () {
string x = "outer block";
{
string x = "inner block";
cout << "x: " << x << '\n';
}
cout << "x: " << x << '\n';
return 0;
}
Even though we have a variable x declared twice in the same program, the use of curly braces makes the visibility of the second instantiation of x = "inner block" to not be affected by the first instantiation of x = "outer block" . The second x is declared in an anonymous block similar with a function declaration without a name, that makes all the variables declared inside of it will have their scope only until the block ends.
So, the output will be
x: inner block
x: outer block
Only if we would have not use the second string instantiation then the x would have had the same visibility.
#include <iostream>
using namespace std;
int main () {
string x = "outer block";
{
x = "inner block";
cout << "x: " << x << '\n';
}
cout << "x: " << x << '\n';
return 0;
}
x: inner block
x: inner block
With this article at OpenGenus, you must have the complete idea of different ways to delete a string in C++.