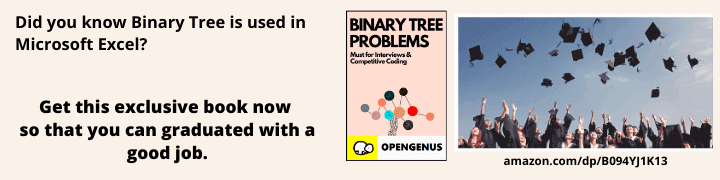
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we were going to learn about the different ways with which we will be able to terminate the program at any point of the execution of the C++ program.
A program used to terminate when the final lines of code gets executed.This leads to free up the allocated resources.However, at times a need may arise to terminate a program when some condition is met. Like every other programming languages, there are certain functionalities available to end or terminate the program in C++ on call.
Here are the multiple ways to terminate a program in C++:-
- Using the return statement.
- Using try and catch block.
- Using the exit() function.
- Using the _Exit() function.
- Using the quick_exit() function.
- Using the abort() function.
- Using the terminate() function.
We will, now, discuss different methods to terminate or exit a program in C++.
return
In C++ the program terminates when the return statement is encountered in the main() function of the program. We used to return 0 int the main() function to represent a normal exit.
When the compiler encounters the return statement int the main() function, all the static objects are destroyed leading to freeing up resources. This happens in the reverse order of the initialization of the objects.
Let us take an example,
#include <iostream>
using namespace std;
int main()
{
cout << "Hi";
return 0;
cout << "Bye";
}
The result of the above code:-
Output:-
Hi
In the above output we notice that it prints only Hi since the program terminates on encountering the return statement.
try and catch block
There also exists another way to use the return statement.We know about the try and catch block in C++. If we try to think that a code block can throw some exception. we place it in the try block and if the exception occurs it gets trapped in the catch block. This prevents the program from terminating or exiting in case an exception occurs in C++.
If we use this in the main() function and place the return statement inside the catch block, then the program will close normally as happens and simultaneously the required cleanup of resources happens.
Let us take an example,
#include <iostream>
#include <stdlib.h>
using namespace std;
int main()
{
cout<< "Shikhar" << endl;
try{
throw;
}
catch(...){
return 0;
}
cout << "Dhawan";
}
The result of the above code:-
Output:-
Shikhar
In the above output we notice that it prints only Shikhar since we placed the return statement inside the catch block and due to exception it gets trapped in the catch block which leads to termination of the program.
exit()
The exit() function is present in the stdlib.h header file and used to exit the program when encountered. We always have to specify the exit code inside the function. This code can be constants EXIT_SUCCESS (which is 0) and EXIT_FAILURE which are also specified in the same header file.
It ignores all the statements after the exit() function is encountered. It also performs the same cleanup as it is done in the previous method.
The general prototype of the exit() is :-
void exit(int ExitCode);
Let us see in the example,
#include <iostream>
#include <stdlib.h>
using namespace std;
int main()
{
cout << "Good Bye";
exit(0);
cout << "Sayonara";
}
The result of the above code:-
Output:-
Good Bye
In the above output we notice that it prints only Good Bye since as soon as the control encounters exit() function, it ignores the rest of the code and the program terminates.
_Exit()
This function terminates a program normally without performing any cleanup of resources as what used to happen in the above defined functions. It also does not call the atexit() function (which registers the given function to be called at normal process termination, either via exit(3) or via return from the program's main(). Functions so registered are called in the reverse order of their registration; no arguments are passed) handler during termination of the program.
We also need to specify the exit code in this function.
The general prototype of the _Exit() is :-
void _Exit(int exit_code); // Here the exit_code represent the exit
// status of the program which can be
// 0 or non-zero.
For example,
#include <iostream>
#include <stdlib.h>
using namespace std;
int main()
{
cout << "Hello" << endl;
_Exit(0);
cout << "Hi";
}
The result of the above code:-
Output:-
Hello
In the above output we notice that it prints only Hello since as the control encounters _Exit(0), it terminates the program with no cleanup of the resources.
quick_exit()
The quick_exit() function exits a program normally without performing complete cleanup of the resources relating to _Exit() function. It calls the specifiers of at_quick_exit() (which registers a function to be called on quick program termination i.e. terminated via quick_exit().The function registered with at_quick_exit() function is called when quick_exit() function is called) instead of calling the at_exit() during termination.
After calling the at_quick_exit() function, it calls the _Exit() function to terminate the program.
The general prototype of the quick_exit() is :-
void quick_exit(int exit_code);
So for example,
#include <iostream>
#include <stdlib.h>
using namespace std;
int main()
{
cout << "Hello Hi" << endl;
quick_exit(0);
cout << "Bye Bye";
}
The result of the above code:-
Output:-
Hello Hi
In the above output we notice that it prints only Hello Hi since as soon as control encounters quick_exit(0) function which calls the specifiers of at_quick_exit() which in turn calls _Exit() function to terminate the program.
abort()
This function is defined in the cstdlib.h header file and causes an abnormal exit from the program and should must be used in extreme cases only. No cleanup of resources is done and only a signal is sent to the OS about the termination.
It does not call the at_exit() or at_quick_exit() functions during the termination.
The above statement would be clear from the sample code mentioned below,
#include <iostream>
#include <stdlib.h>
using namespace std;
int main()
{
cout << "Hi" << endl;
abort();
cout << "Hello";
}
The result of the above code:-
Output:-
Hi
In the above output we notice that it prints only Hi since as soon as the controls encounters the abort() function an abnormal termination of the program happens.
terminate()
This function is defined in the exception header file. The C++ directly calls this function when the program cannot execute further due to reasons like an exception being thrown and not caught and more, during runtime .
When called directly, it calls the terminate_handler() function and then executes the abort() function as discussed previously to cause an abnormal termination of the program.
For example,
#include <iostream>
#include <stdlib.h>
using namespace std;
int main()
{
cout << "Rahul" << endl;
terminate();
cout << "Amitabh";
}
The result of the above code:-
Output:-
Rahul
terminate called without an active exception
In the above output we notice that it prints only Hi with a message "terminate called without an active exception" since as soon as the control encounters terminate() function an abnormal termination of the program happens as it happened previously.