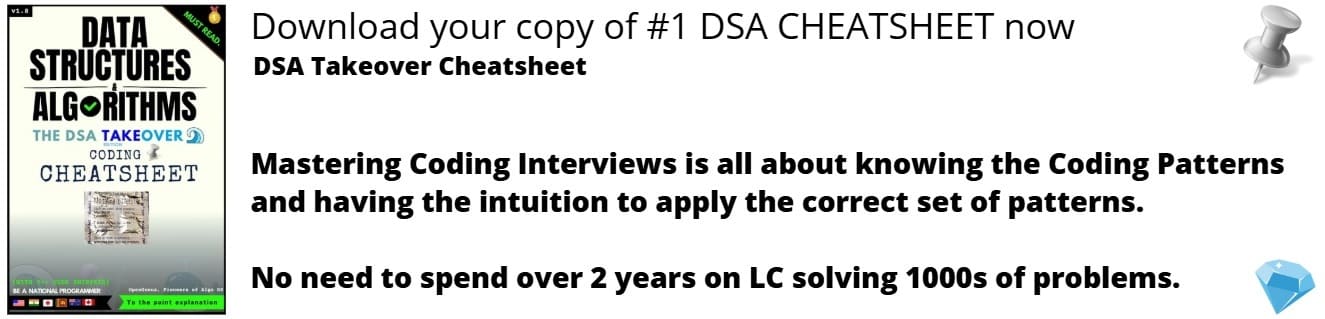
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Dynamic Memory Allocation in C++ is the process of allocating memory while a program is running or at run time rather than at compile time. C++ uses new and delete operator for dynamic allocation and freeing up of memory. In this article, we will discuss about it in detail.
Table of Content:
- Why Dynamic Memory Allocation is needed?
- Stack Memory vs Heap Memory
- How Dynamic Memory is different?
- How is Dynamic Memory Allocation done?
- new Operator
- Allocation of memory block
- Initialization with value
- Allocation of multiple memory blocks
- How Normal Array is different from
new data-type[]
? - delete Operator
Why Dynamic Memory Allocation is needed?
Often while programming, two types of situation arises.First,when our memory needs can't be determined before compile time, it can only be determined during run time. We need some mechanism to allocate our resources at run time. Second, named object's lifetime is limited to its scope. For example, suppose an array is local to function it gets deallocated as function terminates or completes. Hence, we need to create an object that exists independently of its scope.(Pointers comes in handy here). Such situations creates a need for programmer to opt for Dynamic Memory Allocation Techniques. To know about Dynamic Memory Allocation in C, refer Dynamic memory allocation in C
Stack Memory vs Heap Memory
In C++, allocated memory is of two types:
- Stack Memory: Non-static and local variables get memory allocated on Stack.
- Heap Memory: Dynamically allocated memory is allocated on Heap. Also called Free Store.
How Dynamic Memory is different?
In static memory allocation, memory is automatically allocated and deallocated. For dynamically allocated memory like int p = new int[100]
, it's the responsibility of programmers to deallocate memory when no longer needed. If deallocation of memory doesn't happen,then it causes memory leak.
How is Dynamic Memory Allocation done?
In C, malloc()
, calloc()
and free()
functions are used to allocate/deallocate memory dynamically at run time. Along with it, C++ has two additional operators new
and delete
that perform the task of allocating and freeing the memory in a better and easier way.
So, there are 5 functions for Dynamic Memory Allocation:
- malloc
- calloc
- free
- new
- delete
new Operator
new
operator requests for memory allocation on free store. new
is followed by a data type specifier and, if a block of memory is required, then number of elements is specified within brackets []
. If sufficient memory is available, it returns a pointer to the beginning of the new block of memory allocated.
Allocation of memory block
To allocate memory to contain one single element of type data-type, it is written next to new
. On successful allocation, it return pointer to allocated memory block. Hence, pointer-variable is used to store the pointer of type data-type.
Syntax
pointer-variable = new data-type;
Data-type could be any built-in data type including array or any user defined data types including structure and class.
Example
int *foo=new int;
Initialization with value
Using new
operator, we can even initialize the memory with value. To do so, Value is specified within the parenthesis next to data-type.
Syntax
pointer-variable = new data-type(value);
Example
int *foo=new int(5);
On successful execution, foo
points to memory block containing value 5
.
Allocation of multiple memory blocks
Multiple blocks of memory can be allocated at once using new
operator. To allocate contiguous blocks of memory, number_of_elements is provided in []
brackets with data-type. On successful execution, it returns pointer to the first element of the memory block.
Syntax
pointer-variable = new data-type[number_of_elements];
Example
int *foo=new int[5];
On successful execution of this statement, foo
points to a block of memory with space for five elements of type int
.
How Normal Array is different from new data-type[]
?
The main difference between them is that normal arrays are deallocated by compiler automatically whereas dynamically allocated arrays always remain until they are deallocated explicitly or program terminates.
Question
If sufficient memory is not available while dynamically allocating memory using new operator, what happens?
delete Operator
Memory allocated dynamically is only needed during specific periods of time within a program; once it is no longer needed, it needs to be freed back so that the memory becomes available again for other requests of dynamic memory. For this purpose delete
is used , whose syntax is:
// Release memory pointed by pointer-variable
delete pointer;
It releases the memory of a single element allocated using new
.
/ Release block of memory pointed by pointer-variable
delete[] pointer;
It releases the memory allocated multiple blocks using new
.
The delete
operator may be applied only to a pointer returned by new
or to NULL
. (In case of NULL, it has no effect)
Example
//It will free the entire array pointed by foo
delete []foo;
With this article, you must have a strong idea of Dynamic Memory Allocation in C++.