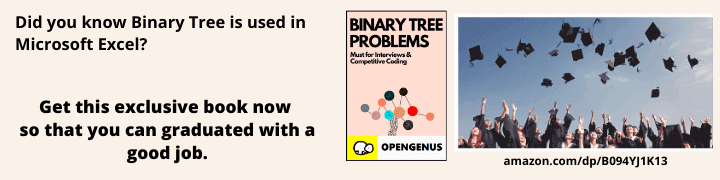
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Explicit is a keyword in C++ which was introduced with C++ 11 this is used to cast a data type and also to change the by default implicit conversion in C++ , but what is implicit conversion then, so let's first learn about implicit conversion then we will see how explicit keyword is used to stop implicit conversion.
Explicit is a new keyword introduced in C++20 in 2020.
What is implicit conversion ?
Implicit conversion in c++
implicit conversion is the automatic conversion done by the compiler if the programmer doesn't specify it.
we cannot perform operations on two variables with different data types therefore if we don not explicitly specify it then compiler automatically converts one data type to other data type.
lets take a example
int x = 10 ;
float y = x + 3.2 ;
In the above program x is automatically converted to float data type before performing operation and the result stored in y is -
10.0 + 3.2 = 13.2
let's take other example
int y = 'A' + 1 ;
In the above code first 'A' is converted to int to it's ascii value then 1 is added to it so value stored in y is 66.
This type of conversion is implicit conversion.
Explicit conversion
Explicit
explicit means the conversion which is stated by user at the time of writing of the program.
let's say that two variables are added but the programmer doesn't want to have default implicit conversion but rather wants the conversion to be defined so in that scenario explicit conversion can be used .
let's see a example
float x = 3.5 , y = 3.5 ;
int z = x + y ;
In the above case the value stored in z is 7.
but let's say use want to add only the floor value of both the variables the
then can explicitly define it as follows -
float x = 3.5 , y = 3.5 ;
int z = (int)x + (int)y ;
In the above case the value stores in z is 6 which is the sum of base value of both variables.
Other uses of explicit conversion
explicit keyword is also used to stop some implicit conversions which are sometimes unexpected , let's take a example to understand this point -
#include <iostream>
using namespace std;
class OpenGenus{
public :
int a ;
string s ;
OpenGenus( std::string &str ){
this->s = a ;
}
OpenGenus( int a ){
this->a = a ;
}
} ;
int main(){
string s = "openGenus" ;
OpenGenus y(s) // works as expected
OpenGenus x = s ; // works only because of implicit conversion
return 0 ;
}
The above program runs and compiles fine , In this you might be confused with line
defining the class object (OpenGenus x = s) , This works only because of implicit conversion and we have a constructor which can instantiate the object based on the string but let's say we don't want this default functionality then -
we can use the explicit keyword as follows -
#include <iostream>
using namespace std;
class OpenGenus{
public :
int a ;
string s ;
explicit OpenGenus( std::string &str ){
this->s = a ;
}
explicit OpenGenus( int a ){
this->a = a ;
}
} ;
int main(){
string s = "openGenus" ;
OpenGenus y(s) // This works fine without any issues
OpenGenus x = s ; // now this gives compilation error
return 0 ;
}
Now the line ( Opengenus x = s ) gives compilation error because we have already defined the constructor using the explicit constructor and therefore implicit conversion is not allowed .
Use case of Explicit keyword
Existence of Explicit keyword in C++ is very important because In very large codebase we might be accidentally casting things without knowing it which may cause performance issues or bugs.
In some of the math libraries numbers are directly converted to vectors so to avoid such conversions be as safe as possible and avoid accidental conversions we can use explicit keyword.